Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In this exercise, you are going to implement a hash table from scratch. You must not use any of the Java collection APIs. In this
In this exercise, you are going to implement a hash table from scratch. You must not use any of
the Java collection APIs.
In this implementation of hash table, you will create a hash table of keyvalue pairs where both key and value are String objects. The hash table will be implemented using a fixed capacity array and chaining if multiple key values have the same hash function value, the keyvalue pairs are put into a linked list. The basic unit of storage is a Node, consisting of a key, a value, and a reference to the next node, the same as the node used in a raw implementation of a linked list.
Download the provided Java file. There is a menudriven driver program class to test. Your job is to implement the missing methods to put key value pairs into the table as nodes, and perform various other operations on them.
public class LabCS
public static void mainString args
MyHashTable table new MyHashTable;
String key,value;
while true
System.out.println
Menu:";
System.out.println Populate table";
System.out.println Test putkeyvalue;
System.out.println Test getkey;
System.out.println Test containsKeykey;
System.out.println Test removekey;
System.out.println Show contents of hash table.";
System.out.println EXIT";
System.out.printEnter your command: ;
java.util.Scanner scnew java.util.ScannerSystemin;
int item scnextInt;
switch item
case :
table.putjan;
table.putfeb;
table.putmar;
table.putapr;
table.putmay;
table.putjun;
table.putjul;
table.putaug;
table.putsep;
table.putoct;
table.putnov;
table.putdec;
break;
case :
scnew java.util.ScannerSystemin;
System.out.printEnter Key : ;
key scnext;
System.out.printEnter Value: ;
value scnext;
table.putkeyvalue;
break;
case :
scnew java.util.ScannerSystemin;
System.out.printEnter Key : ;
key scnext;
System.out.printValue for this key is table.getkey;
break;
case :
scnew java.util.ScannerSystemin;
System.out.printEnter Key: ;
key scnext;
System.out.println
containsKey key is table.containsKeykey;
break;
case :
System.out.printEnter Key to remove: ;
scnew java.util.ScannerSystemin;
key scnext;
table.removekey;
break;
case :
table.show;
break;
case :
return;
default:
System.out.println Illegal command.";
break;
System.out.println
Hash table size is table.size;
class MyHashTable
private static class Node
String key;
String value;
Node next;
public NodeString key, String value
this.keykey; this.valuevalue; nextnull;
public String toString
return keyvalue;
private Node nodeArray; fixed size Array to hold the head of linked lists
public int count; total key value pairs in the entire hash table
public MyHashTable
nodeArray new Node; a very small table
public MyHashTableint initialSize
if initialSize
throw new IllegalArgumentExceptionIllegal table size";
nodeArray new NodeinitialSize;
public void putString key,String value
Change this Your Code here
return;
public int hashString key
return key.hashCodenodeArray.length;
public String getString key
Change this YOur Code
return ;
public void show
Change this Your Code
public boolean containsKeyString key
Your Code change this
return false;
public void removeString key
Change this Your Code
return;
public int size
return count;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
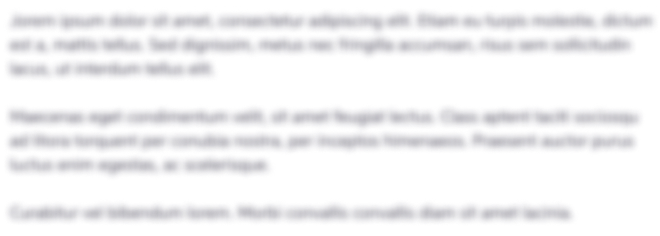
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started