Question
In this file is a function called 'equals' that doesn't quite work with floating point values due to round off errors. There are two examples,
In this file is a function called 'equals' that doesn't quite work with floating point values due to round off errors. There are two examples, one of which doesn't work. Do the following: 1. Why is the first example not working? 2. Fix the function 'equals' so that it works for both examples. We want the two numbers to be considered equal if they are equal in the first 3 decimals after the decimal point. NOTE: There are several ways to do this. You can take the absolute value of the difference between a and b and compare it to a tolerance value. In other words, if |a-b| < tol is true, then we can say the values are 'equal'. The tolerance value should be just small enough to act as a 'cutoff' point for equality but no smaller.
CODE
================================================
/* PUT YOUR NAME HERE * Put answers to * questions here. * * */
#include
int equals(float a, float b) { if(a == b) return 1; return 0; }
void example1() { float a=100.6; float b=100.0; for(int i=0; i < 6; i++) { a = a - .1; } printf("Example 1: these should equal each other "); if(equals(a, b)) { printf("%f and %f are equal. ", a, b); } else { printf("%f and %f are NOT equal. ", a, b); } }
void example2() { float a=100.006; float b=100.0; for(int i=0; i < 7; i++) { a = a - .001; } printf("Example 1: these should NOT equal each other "); if(equals(a, b)) { printf("%f and %f are equal. ", a, b); } else { printf("%f and %f are NOT equal. ", a, b); } }
int main() { example1(); example2(); return 0; } ==========================================
Step by Step Solution
There are 3 Steps involved in it
Step: 1
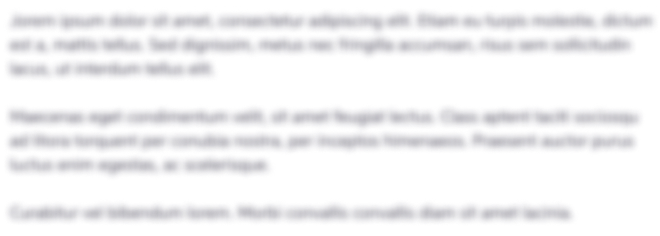
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started