Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In this homework, you are supposed to implement a Java program for a hospital database by using binary search trees. You must use your own
In this homework, you are supposed to implement a Java program for a hospital database by using
binary search trees. You must use your own implementation of binary search trees by taking
inspiration from your textbook or the web. You are not allowed to use any external library or jar
file.
In this hospital database, for each patient, you will have an entry in which you keep:
The first name and last name of the patient,
Date the patient visited the hospital month day, and year
The first name and last name of the doctor,
A list of its patients care team. For each medical staff member in the hospital, you should
keep:
a The first and last name of a medical staff member, and
b The professional role they fulfill Nurse Anesthesiologist, etc.
In your implementation, you must keep patient entries in the binary search tree based on the year
the patient visited the hospital. Then, to keep the care team of each patient, you should create a
binary search tree in which the medical staff members are kept by their names eg if you have
five patients in your binary search tree, you will have five more binary search trees: one for the
care team of each patient. To deal with patients of the same year, you can define your binary
search tree for a node such that elements with smaller values are kept in the left subtree of the
node, and elements with larger or equal values are kept in its right subtree.
Your system will have the following functionalities; the details of these functionalities are given
below see the end of this document for sample inputsoutputs:
Add a patient
Remove a patient
Add a medical staff member to the care team of a patient
Remove a medical staff member from the care team of a patient
Show the list of patients
Show detailed information about a particular patient
Query the patients who were seen by a particular doctor
Query the patients who visited in a given year
Add a patient: This function adds an entry to the hospital database for a given patient whose name
first name last name doctors name first name last name and visit date day month
year are specified as parameters. In this function, the care team is not specified; the medical staff
members that cared for the patient will be added later. In this system, the names first name last
name of the patients are unique. Thus, if the user attempts to add a patient with an existing name,
you should overwrite old information.
Remove a patient: This function removes a patient, whose name is specified as a parameter, from
the hospital database. If this patient does not exist in the database ie if there is no patient with a
specific name you should not allow this operation and give a warning message.
Add a medical staff member: This function adds a medical staff member to the care team of a
patient. For that, the name of the patient to which the medical staff member is added, the medical
staff members name first name last name and the title of the professional role fulfilled by the
medical staff member are specified as parameters. In this function, you should consider the
following issues:
If the patient with a specific name does not exist in the database, you should not allow the
operation and give a warning message.
In this system, all medical staff members names are unique within the same care team.
Thus, if the user attempts to add a medical staff member with an existing name within the
same care team, you should overwrite old information. Please note that a medical staff
member can work in different care teams.
Remove a medical staff member: This function removes a medical staff member from the care
team of a patient. For that, the name of the patient from which the medical staff member is removed
and a medical staff members name first name last name are specified as parameters. If the
patient with a specific name does not exist in the database, you should not allow the operation and
give a warning message. Similarly, if the medical staff member is not in the care team of the
specified patient, you should not allow the operation and give a warning message. Note that after
calling this function, the care team should remain sorted in ascending order.
Show the list of patients: You should list all patient entries in the hospital database on the screen
in the following format. If the database does not contain any patient, you should display
none Your system should display patients in ascending order by visit year.
NameFirst name Last Name visit year, visit name for the st patient
NameFirst name Last Name visit year, visit name for the nd patient
NameFirst name Last Name visit year, visit name for the rd patient
Show detailed information about a particular patient: You should display all of the information
about a patient whose name is specified as a parameter
Step by Step Solution
There are 3 Steps involved in it
Step: 1
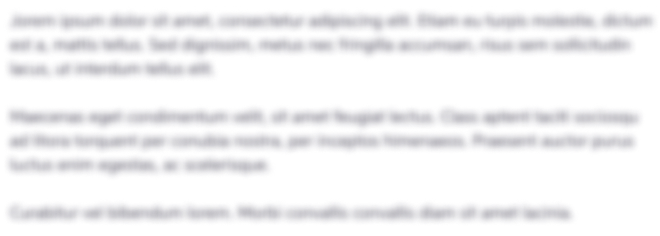
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started