Question
In this homework, youll get to work with polymorphism and apply it to some very low-budget graphics. The implementation that you choose is somewhat up
In this homework, youll get to work with polymorphism and apply it to some very low-budget graphics. The implementation that you choose is somewhat up to you provided it conforms to the functionality described below. In the end, you will submit 5 files: Collection.h, Shape.h, Square.h, Diamond.h, and Graphics.cpp.
Conventions: this problem is about plotting a Collection of Shapes. Each shape is made up of a discrete set of points at specific x- and y-values. A Collection will only display and count the points corresponding to Shapes it stores that are within its x- and y-ranges. The x-positions increase as we move right (increasing column number); and the y-positions increase as we move down (increasing row number).
Okay, now that weve established this, lets consider the classes. The following is the bare minimum necessary for you to understand what is required, but you may need to add further functions, specifications, or variables than those specified.
The Collection class stores a drawing area and shapes. It should
have a constructor accepting 4 int inputs that respectively denote the lowest x position, the highest x-position, the lowest y-position, and the highest y-position over which the class should display in its window;
have an addShape function that, when provided with a std::shared ptr object, adds it to the collection;
have a display function to display its shapes only over the appropriate range of x and y-values, from the lowest to highest values inclusive, printing * if there is a part of a shape at a point and - if there is no part of a shape a point; following this, it should list the shape types, in the order they were added to the collection and this function must make use of dynamic pointer cast (other means of doing the same thing are possible but the point of this exercise is to practice using dynamic pointer cast so you must do it here);
have a totalStars function to return the total number of stars that are within the plot range;
have a moveBy function taking two arguments, the first describing how much to move all shapes in the x-direction and the second describing how much to move all shapes in the y-direction; and
have a setSize function taking a single argument and setting the size property of all shapes.
The Shape class should abstractly represent a shape and it should
have a constructor accepting three parameters that respectively denote the shapes x- and y-position and size;
have a purely virtual function setStars that resets the values of the coordinates storing a shapes location should it be moved or resized;
have a moveBy function accepting two int inputs that respectively denote how much to move the shapes center in the x- and y-directions, respectively, and which invokes setStars afterwards; and
have a setSize function accepting an input for the new size parameter that invokes setStars afterwards.
The Square class should:
have a constructor accepting three parameters: the first two representing its x- and y-position (and for the square this denotes its top left position) and the third representing its size (and for the square this is the number of stars for its height/width).
The Diamond class should:
have a constructor accepting three parameters: the first two representing its x- and y-position (and for the diamond this denotes its center) and the third representing its size (and for the diamond, this is the number of stars from its center point to its top inclusive).
Note that the Diamond class looks like a diamond and it is symmetric about its centerline (see the output figure).
You may assume the user will only enter valid parameters and use the function names consistent with this homework.
Example main:
#include "Shape.h"
#include "Square.h"
#include "Diamond.h"
#include "Collection.h"
#include
#include
int main() {
// top left at (1,2), size 2x2
std::shared_ptr sp1 = std::make_shared(1, 2, 2);
// center at (5,8), size 3
std::shared_ptr sp2 = std::make_shared(5, 8, 3);
// top left at (-1,-1), size 4
std::shared_ptr sp3 = std::make_shared(-1, -1, 4);
// x from 0 to 10, y 0 to 10, inclusive
Collection c(0, 10, 0, 10);
// add the shapes
c.addShape(sp1);
c.addShape(sp2);
c.addShape(sp3);
// display the shapes
c.display();
std::cout << ' ';
// check total number of stars on display
std::cout << "Total visible stars: " << c.totalStars() << ' ';
//add another square
c.addShape(std::make_shared
// change all shapes to have size 2 including the newly added square
c.setSize(2);
// move sp3 right 2 and down by 4
sp3->moveBy(2, 4);
// move all shapes left 1
c.moveBy(-1, 0);
// display the shapes
c.display();
std::cout << ;
// check total number of stars on display
std::cout << "Total visible stars: " << c.totalStars() << ;
std::cin.get();
return 0;
}
Some initial guidance to get you on your way...
1. Start by writing the Shape class. It is, after all, the base class.
2. Although not required, you may wish to use the template class std::pair, which is found in the header. Look this up, if you havent seen pairs before; its easy to use, and a std::vector of them could be useful to store all the points where there is part of a shape present.
3. The functions moveBy and setSize can be implemented directly in the Shape class and just before the end of their function bodies, they can call setStars.
4. Both Square and Diamond should have their own implementation of setStars that will update the locations of all the stars after a shape is moved or resized.
5. For Diamond: think of a diamond as a juxtaposition of two triangles. It might be easiest to set its star positions by writing two separate nested for-loops.
6. You may wish to include a helper member function in Collection that returns a bool, true if there is a star present and otherwise false, when given an x- and y-position. This could help with the display member function.
7. The dynamic cast allows you to differentiate between Squares and Diamonds.
8. There is no is a relationship, one way or the other, between Collection and any of the other classes you need to write here.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
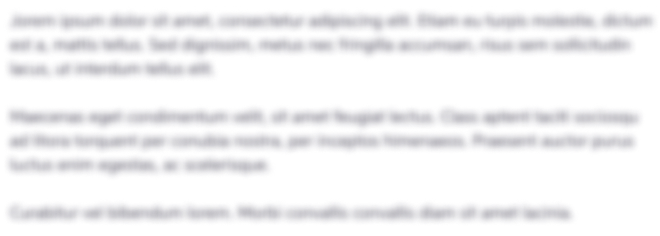
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started