Question
In this Java project you will: Create a package called accounts with the following classes Account Account stores private information about a customers account, including
In this Java project you will:
Create a package called accounts with the following classes
- Account Account stores private information about a customers account, including the account number, balance, transaction counter, pin, and an ArrayList of customers ID numbers (just numbers, not customer objects) that are owners of the account. Looking at the associated accountData.txt CSV data file, we see that each row is an account arranged as:
- Account number 4-digit integer
- Account owner the first owner (other will come later), 3-digit integer, also are
- Account pin 4-digit integer
- Account balance double
There has to be least one constructor with arguments for the number, first owner, pin, and balance. This should instantiate any needed objects and set the object data values with the incoming information. The transaction count is set to zero.
Create the methods that do the following:
- Retrieve account number
- Retrieve balance
- Retrieve list of owners
- Retrieve transaction counter
- Makes a deposit given an amount passed in, increments the transaction counter, returns the updated balance
- Makes a withdraw given an amount passed in, increments the transaction counter, returns the updated balance
- Checks a pin passed in to see if it matches the account pin
- Checks a number passed in to see if it matches the account number
- Checks a customer ID passed in to see it if matches an owner of the account
- Adds the owner to the owner list if it is not already in the list
- Removes an owner if they are in the list
- Add interests, interest = balance x (APR / 12.0) to the account using a given the APR passed in as a double and returns the balance. This is not an APR object (described later) passed in
- Prints the account information using the following as an example. Note that the owner is an ArrayList, therefore, you can print it by putting the variable in your print statement. Limit doubles to two decimal places.
#75646 owner=[4567, 9362] pin=2364 balance=$9874.42
- AccountList Holds the private ArrayList of accounts and an account number generator (described later). It must have a no-argument constructor that will instantiate the objects. It must also have a constructor with one argument that will bring in an ArrayList of accounts, instantiates the objects and sets the objects, and will add any account numbers to the number generator. It must also have methods that:
- Uses passed in arguments for owner, PIN, and balance, to create a new account. This method should generate the new account number, add the account to the list. and return the new account number.
- Adds a passed in account object to the list of accounts. Assume that the account object already has an unused account number that will need to be added to the account number generator (again described later). It should return the account number.
- Adds a new owner to an account of the account list given the account number and new owner number as arguments. It should search the list for the account, and if found, search the owner list to make sure the owner is not already in it. If everything checks out, it will add the owner to the owners list in the account. Note that this method does not remove any owners.
- Removes an owner from an account of the account list given the account number and owner number as arguments. Like adding an owner, it should search for the account and owner before trying to remove the owner.
- Prints the account information for each account and prints the account number generator information (described next)
- AccountNumbers A random account number generator that must also keep track of used numbers. Its private data includes an ArrayList of used account numbers and finalized values for the minimum and maximum possible account number (10000 99999). It must have a default no-argument constructor that instantiates the ArrayList. It must also have methods that:
- Adds a passed in number to the list of used numbers
- Generates and returns an unused random number within the range.
- Prints the list of used numbers. Note that it is an ArrayList so you can simply use the variable name in your print statement. It should format as:
[74658, 18273, 28374, 98475, 10293]
- SearchAccount It will eventually be used to search the account list, but for now it will hold privatedata about the account including the account number, pin, and owner. It must have a constructor with arguments to set the number, pin, and owner. It must have a print method that will print the data formatted as shown below. Note that this only holds one owner number, not an ArrayList of them.
#28374 owner=3847 pin=9283
- Create a Package called apr that has the following classes
- APRs The bank pays interest on accounts. The amount of interest depends on the current account balance and a range of APRs. APR is a class that holds private data about the rate and minimum account balance needed to get that rate. In the apr.txt data file, each row has two doubles.
- APR rate the rate later used to calculate the interested in an account
- APR minimum the minimum balance needed to get that interest rate.
- APRs The bank pays interest on accounts. The amount of interest depends on the current account balance and a range of APRs. APR is a class that holds private data about the rate and minimum account balance needed to get that rate. In the apr.txt data file, each row has two doubles.
Do not calculate interest right now, just build the objects for it. Each instantiated object will hold on rate and minimum.
There should be a constructor with two arguments, the rate and the minimum, which is used to set the data members. It must also have these methods:
- Retrieves rate
- Retrieves minimum
- Retrieves a new APR object that has this objects rate and minimum
- Prints the rate and minimum information like shown below. Limit the rate to four decimals and the minimum to two decimals
rate=5.0000 min=$2300000.00
- APRList This class will hold a public ArrayList of APR objects. It must have constructors:
- A default no argument constructor that instantiates the APR list as an empty list
- A constructor that must instantiate the APR list using an APR ArrayList passed in
This class should have the following:
- Add a new APR object to the list given a rate and minimum passed in. It must check to make sure the minimum does not already have an APR in the list because you do not want two rates for the same minimum. It must also keep the list in ascending order by minimum. It must also return true or false depending on if the APR was added to the list.
- Remove an APR object from the list if it meets a minimum passed in. The method should return true or false depending on if the APR is removed.
- Retrieve the APR for a balance passed in. If the balance is less than or equal to zero, it should return zero. When writing the method, keep in mind that that the APR list can change so you must write it to take advantage of the ordered list.
- Print the list of APRs using the APR objects print method
Step by Step Solution
There are 3 Steps involved in it
Step: 1
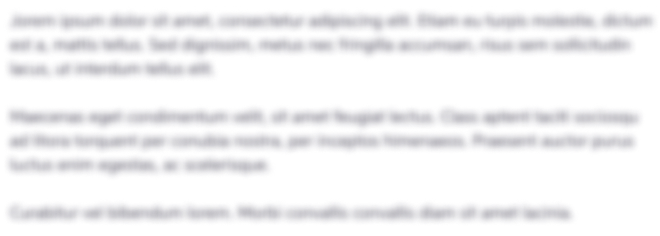
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started