Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In this journal assignment, you will reflect on the contact service you submitted in Module Three and the task service you submitted in this module.
In this journal assignment, you will reflect on the contact service you submitted in Module Three and the task service you submitted in this module. Specifically, you will describe your unit testing approach and describe your experience with writing the JUnit tests. This will help to prepare you for the Project Two submission, due in Module Seven, in which you will submit a summary and reflections report.
Address the following questions:
To what extent was your testing approach aligned to the software requirements? Support your claims with specific evidence.
Defend the overall quality of your JUnit tests for the contact service and task service. In other words, how do you know that your JUnit tests were effective on the basis of coverage percentage?
How did you ensure that your code was technically sound? Cite specific lines of code from your tests to illustrate.
How did you ensure that your code was efficient? Cite specific lines of code from your tests to illustrate.
Contact.java
public class Contact
private String contactId;
private String firstName;
private String lastName;
private String phoneNumber;
private String address;
public ContactString contactId, String firstName, String lastName, String phoneNumber, String address
this.contactId contactId;
this.firstName firstName;
this.lastName lastName;
this.phoneNumber phoneNumber;
this.address address;
Getters and setters
public String getContactId
return contactId;
public String getFirstName
return firstName;
public void setFirstNameString firstName
this.firstName firstName;
public String getLastName
return lastName;
public void setLastNameString lastName
this.lastName lastName;
public String getPhoneNumber
return phoneNumber;
public void setPhoneNumberString phoneNumber
this.phoneNumber phoneNumber;
public String getAddress
return address;
public void setAddressString address
this.address address;
ContactTest.Java
public class Contact
private String contactId;
private String firstName;
private String lastName;
private String phoneNumber;
private String address;
public ContactString contactId, String firstName, String lastName, String phoneNumber, String address
this.contactId contactId;
this.firstName firstName;
this.lastName lastName;
this.phoneNumber phoneNumber;
this.address address;
Getters and setters
public String getContactId
return contactId;
public String getFirstName
return firstName;
public void setFirstNameString firstName
this.firstName firstName;
public String getLastName
return lastName;
public void setLastNameString lastName
this.lastName lastName;
public String getPhoneNumber
return phoneNumber;
public void setPhoneNumberString phoneNumber
this.phoneNumber phoneNumber;
public String getAddress
return address;
public void setAddressString address
this.address address;
ContactService.java
import java.util.HashMap;
import java.util.Map;
public class ContactService
private Map contacts;
public ContactService
this.contacts new HashMap;
public void addContactContact contact
contacts.putcontactgetContactId contact;
public void deleteContactString contactId
contacts.removecontactId;
public void updateContactString contactId, String firstName, String lastName, String phoneNumber, String address
if contactscontainsKeycontactId
Contact contact contacts.getcontactId;
contact.setFirstNamefirstName;
contact.setLastNamelastName;
contact.setPhoneNumberphoneNumber;
contact.setAddressaddress;
public Contact getContactByIdString contactId
return contacts.getcontactId;
Cimport org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.;
public class ContactServiceTest
@Test
public void testAddContact
ContactService contactService new ContactService;
Contact contact new Contact "John", "Doe", Main St;
contactService.addContactcontact;
assertEqualscontact contactService.getContactById;
@Test
public void testDeleteContact
ContactService contactService new ContactService;
Contact contact new Contact
Step by Step Solution
There are 3 Steps involved in it
Step: 1
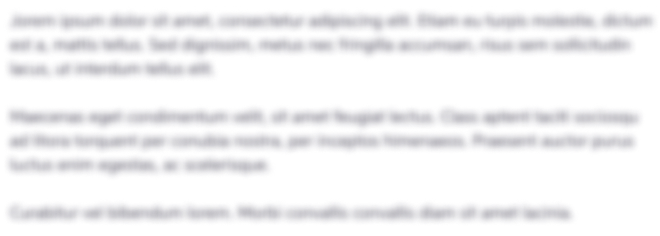
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started