Question
In this lab we will learn how to work with different data types for variables, and perform operations on those variables. By the end of
In this lab we will learn how to work with different data types for variables, and perform operations on those variables. By the end of the lab, you should have a Lab6Tester class that will entirely test your code for this week. (Note that your tester class should have a main method). The entire lab will be due next week. PLEASE COMMENT YOUR CODE. You will have points taken off if you do not comment your code. Keep your code neat. By the end of this lab assignment you will run your code one time, and capture the output to add to your submission. Create a new project in BlueJ. Create a class called Lab6Tester. Create a main method in your class. All of your code will go in this main method. (See the book or previous labs on how to declare a main method). For each of the tasks listed below, simply continue to add code to your main method of your Lab6Tester class. In this lab we are focusing on data types and calculations, and not object-oriented programming. As you add code, your output will continue to grow, and you can take just a single screen-shot of your output once you finish all the tasks below. Make sure your output matches what is asked for in the tasks below. Sometimes a question is asked, and you have to print out your answer (using the println method). Sometimes you are asked to put an answer to a question as a comment. I will review your output and your code to make sure all tasks have been completed. 1) Create eight variables, one for each of the primitive types in Java (pg 132), and initialize each variable with any appropriate value. Print out the name of each variable and its value. Modify the value of each variable with an assignment statement and print out the names of the variables and their new values. Next, create eight constants, one for each of the primitive types in Java. Print the name of the constant and its value. (pg 134-135) What happens if you try to assign a value to a constant? Try to assign a value to a constant to see what happens. List your answer as a println() statement. I have started it for you: System.out.println("When you try to assign a value to a constant ..."); //fill in dots with your answer 2) Execute the code below. (Paste it into your program). Each invocation of println outputs an arithmetic expression. The first two println commands are preceded by comments that describe the operations that occur in each expression. Complete the program by adding a comment before each println statement that describes all the arithmetic operations that occur when evaluating the expression that is printed.
2 int a = 3; int b = 4; int c = 5; int d = 17; // 3 and 4 are added with sum 7 // 7 is divided by 5 with quotient 1 System.out.println((a + b)/ c); // 4 is divided by 5 with quotient 0 // 3 is added to 0 with sum 3 System.out.println(a + b / c); // your comments here for next 2 lines a++; System.out.println(a); // your comments here for next 2 lines a--; System.out.println(a); // etc ... System.out.println(a + 1); System.out.println(d % c); System.out.println(d / c); System.out.println(d % b); System.out.println(d / b); System.out.println(d + a / d + b); System.out.println((d + a) / (d + b)); System.out.println(Math.sqrt(b)); System.out.println(Math.pow(a, b)); System.out.println(Math.abs(-a)); System.out.println(Math.max(a, b)); 3) Write a program that prompts the user to enter two integers. Print the smaller of the two numbers entered. Youll need to use a Scanner and a Math method, Math.min(). 4) Suppose you have 5 1/2 gallons of milk and want to store them in milk jars that can hold up to 0.75 gallons each. You want to know ahead of time how many completely filled jars you will have. The following program has been written for that purpose. (Paste it into your project). What is wrong with it? Why? How can you fix it? Print your answer to the questions using println(). Then modify the code with your fix. (Hint: look at loss of precision when doing math between double and int. ). double milk = 5.5; // gallons double jarCapacity = 0.75; // gallons int completelyFilledJars = milk / jarCapacity; System.out.println("Number of Filled Jars: " + completelyFilledJars); 5.1) You want to know how many feet are in 3.5 yards, and how many inches are in 3.5 yards. You write the following code for that purpose: double yards = 3.5; double feet = yards * 3; double inches = feet * 12;
John Wiley & Sons, Inc. All rights reserved. 3 System.out.println(yards + "yards are" + feet + "feet"); System.out.println(yards + "yards are" + inches + "inches"); The problem with the program above is that using "magic numbers" makes it hard to maintain and debug. Modify the program so that it uses constants to improve legibility and make it easier to maintain. (You will fix the println() statements in the next step of the lab). 5.2) Run the code for 5.1. What is the output? (just look at the output, you don't need to answer this question). What change(s) would you make to the program to make the output more readable? (hint: add spaces to println() statements). Don't have to answer this question either, just MAKE the changes that you need to make in order to fix the output of the code to be more readable. 6) Adding (incrementing) or subtracting (decrementing) the value one from an integer variable is a common, everyday operation. To increment an int variable x, we could code x = x + 1; As an alternative, we could use the special operators ++ and -- to increment and decrement a variable . Use the first method to increment x in the program below (i.e. x=x+1). Print the value of x after incrementing (don't forget to also print a label for the value you are printing. println(x); is not a valid answer). Use the ++ operator to increment y in the program below. Print the value of y after incrementing. int x = 10; int y = -3; // Put your code here 7.1) An annuity (sometimes called a reverse mortgage) is an account that yields a fixed payment every year until it is depleted. The present value of the annuity is the amount that you would need to invest at a given interest rate so that the payments can be made. The present value of an annuity (PVann) at the time of the first deposit can be calculated using the following formula: PVann = PMT ({[(1 + i)n - 1 - 1] / i } / (1 + i)n - 1 + 1) where: PMT: periodic payment i: periodic interest or compound rate n: number of payments What is the present value of an annuity that will pay out $10,000 in each of the next 20 years if the interest rate is 8 percent? (Do this calculation by hand/calculator, then add a println() statement to print this as your expected results). You should get the value: 106035.99.
4 Write a program to calculate the present value of an annuity for these values. Remember that you can use Math.pow(x, y) to calculate xy. Print this value as your actual result. 7.2) Modify the program you created in 7.1 so that the user can provide the values for pmt, i, and n through the console. 8.1) What is the output of the following program? Why? (use a println() statement to put your answer in your code). You may run the code to help get your answer. (Do the calculation by hand or with a calculator to see what the answer should be). int age1 = 18; int age2 = 35; int age3 = 50; int age4 = 44; double averageAge = (age1 + age2 + age3 + age4) / 4; System.out.println("average age: " + averageAge); 8.2) Fix the program in 8.1 so that it yields the correct result. 9.1) What is the output of the following program? Why? (Use println() for your answer) double probability = 8.70; int percentage = (int) (100 * probability); System.out.println(percentage); 9.2) Fix the program from 9.1 so that it displays the correct result. Remember that you can use Math.round to convert a floating-point value to its closest integer. 10) Using the substring method and concatenation, write a sequence of commands that will extract characters from inputString = "The quick brown fox jumps over the lazy dog" to make outputString = "Tempus fugit". Then print outputString. (Along with a label for outputString). Run your code once when you are finished, and capture your output from the terminal window.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
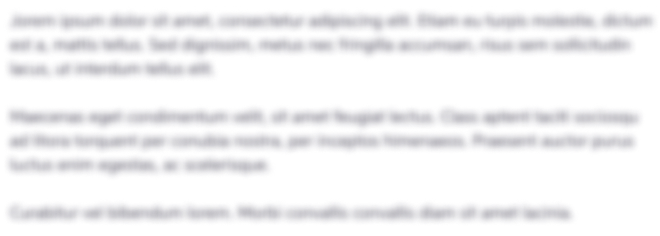
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started