Question
In this lab, you will add a user interface to the Dessert Shop application. To do this, you will make updates to your Dessert Shop
In this lab, you will add a user interface to the Dessert Shop application.
To do this, you will make updates to your Dessert Shop classes as described below:
DessertShop class:
- Create four new methods in the DessertShop class:
- private static DessertItem userPromptCandy()
- private static DessertItem userPromptCookie()
- private static DessertItem userPromptIceCream()
- private static DessertItem userPromptSundae()
- For each of these methods, you will need to:
- Create a Scanner object to take user input in the form of Strings
- Ask the user for the appropriate information to create each item
- Convert any numbers entered from string to the appropriate data type (int or double)
- Use input validation to ensure the user entered numbers when asked to do so.
- Store that information in appropriate variables local to the methods
- Instantiate an object of the appropriate type (class), populating the arguments of the constructors with the data you just received from the user.
- Return the newly created object back to where it was called in main()
- Add the following lines of code to your main() method exactly as written (copy/paste into your program). This should be placed near the top of your main() method, right after you instantiate the order object and before you start adding the items to the order.
public static void main(String[] args) { //already in your program Order order1 = new Order(); //already in your program
Candy candy1 = new Candy("Candy Corn", 1.5, .25); //already in your program order1.add(candy1); //already in your program Candy candy2......... //already in your program
------Begin Copy-----
Scanner sIn = new Scanner(System.in); String choice; DessertItem orderItem;
boolean done = false; while (!done) { System.out.println(" 1: Candy"); System.out.println("2: Cookie"); System.out.println("3: Ice Cream"); System.out.println("4: Sunday");
System.out.print(" What would you like to add to the order? (1-4, Enter for done): "); choice = sIn.nextLine(); if (choice.equals("")) { done = true; } else { switch (choice) { case "1": orderItem = userPromptCandy(); order1.add(orderItem); System.out.printf("%n%s has been added to your order.%n",orderItem.getName()); break; case "2": orderItem = userPromptCookie(); order1.add(orderItem); System.out.printf("%n%s has been added to your order.%n",orderItem.getName()); break; case "3": orderItem = userPromptIceCream(); order1.add(orderItem); System.out.printf("%n%s has been added to your order.%n",orderItem.getName()); break; case "4": orderItem = userPromptSundae(); order1.add(orderItem); System.out.printf("%n%s has been added to your order.%n",orderItem.getName()); break; default: System.out.println("Invalid response: Please enter a choice from the menu (1-4)"); break; }//end of switch (choice) }//end of if (choice.equals("")) }//end of while (!done) System.out.println(" ");
------End Copy-----
DessertItem class:
- No changes
All subclasses (Candy, Cookie, IceCream, Sundae):
- No changes
Step by Step Solution
There are 3 Steps involved in it
Step: 1
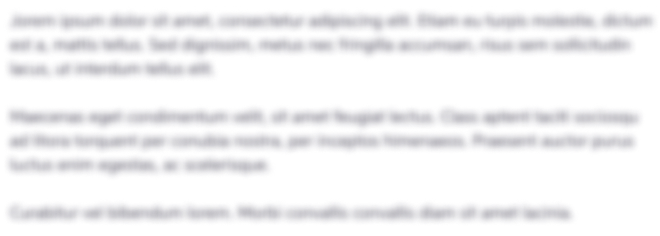
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started