Question
In this lab, you will assign dynamically allocated derived class objects to base class pointers and use polymorphism to display output based on the type
In this lab, you will assign dynamically allocated derived class objects to base class pointers and use polymorphism to display output based on the type of the object.
First, start by implementing the base class Employee. This class will have:
- One private int attribute empID
- One public constructor that will take an int as an argument and assign it to empID - A public function that will return the empID with the following prototype:
int getEmpID() const;
- A public pure virtual function with the following prototype:
virtual void printPay() =0;
Next, implement the derived class HourlyEmployee: public Employee. This class will have:
- One private double attribute hours
- One private double attribute payRate
- One public constructor that will take in three arguments: The employee id, the number
of hours worked, and the pay rate. The constructor will pass the employee id argument to the base class constructor and assign the value of the other two arguments to its attributes.
- A public function that will return the hours with the following prototype: double getHours() const;
- A public function that will return the payRate with the following prototype: double getPayRate() const;
- An overridden public printPay function that will print the hourly empolyees id number and the weekly pay (i.e. hours * payRate) with 2 digits after the decimal
place. The prototype is : void printPay();
Next, implement the derived class SalariedEmployee: public Employee. This class will have:
- -
- -
One private double attribute salary
One public constructor that will take in two arguments: The employee id and the salary. The constructor will pass the employee id argument to the base class constructor and assign the value of the other arguments to its attribute.
A public function that will return the salary with the following prototype: double getSalary() const;
An overridden public printPay function that will print the salaried empolyees id number and the weekly pay (i.e. salary/52) with 2 digits after the decimal place. The prototype is : void printPay();
You will also need to implement two stand-alone functions. The function :
void getInput(vector
will contain a loop that will give the user an option to enter information about an hourly employee or a salaried employee. Depending on the users choice, collect the appropriate input information from the user and use this information to dynamically construct a derived class object. Assign the address of the dynamically created derived class object to an element of the Ve vector. (Note: In this step, you are assigning the addresses of derived class objects to base class pointers).
The other function:
void printList(const vector
will simply loop through the elements of the Ve vector and call the printPay() function of each of the dynamically allocated objects. (Note: In this step, you see the polymorphism in action. That is, depending on the type of the object there will be dynamic binding and the appropriate version of the printPay() function will execute).
All numeric input must be validated . Page 2 of 3
Below is the code for the main function to be used in your program.
int main()
{
vector
getInput(VEmp);
printList(VEmp);
cout << "Lab 7 completed by: " << ??? << endl;
system("pause");
return 0; }
Where ??? represents your first and last name and the employee ID of the first employee in the list. If there are no employees in the list, use your first and last name.
Dont add anything extra to the program(s) and implement the code as described above.
Submission will be as one main CPP program with separate class files (class implementation files .cpp and header files .h). There will be 7 files to submit name all of them with a prefix of
Step by Step Solution
There are 3 Steps involved in it
Step: 1
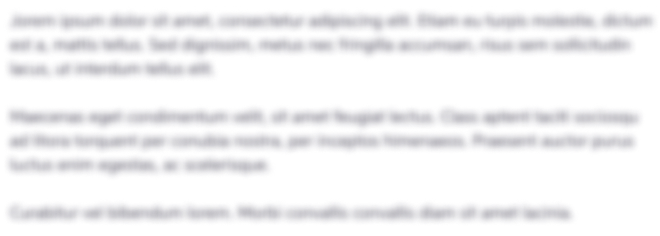
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started