Question
In this lab, you will have to generate a random list of PlayingCard objects for where the suit and rank combinations of each card are
In this lab, you will have to generate a random list of PlayingCard objects for where the suit and rank combinations of each card are randomly choosen. Using this list of randomly created playing cards, you will use dictionaries and lists to store important data about these playing cards, particularly the frequency distribution of each rank-suit combination you created (e.g. like if 10 queen of hearts cards are generated and counted.)
Thanks to the clearly described interface used to communicate to any PlayingCard object, specifically the get_rank() and get_suit() methods, you can just import your Classes lab as a module and use your previously written code!
Assignment Requirement:
Use the provided starter (skeleton) code file for your solution. You are required to use it.
from random import randrange
from lab_classes import PlayingCard
from math import sqrt
suit_size = 13 # Number of cards in a suit.
deck_size = 52 # Number of cards in a deck.
num_cards = 260 # Number of cards to create with random rank & suit values
def make_random_cards():
"""Generate num_cards number of random PlayingCard objects.
This function will generate num_cards RANDOM playing cards
using your PlayingCard class. That means you will have to choose a random
suit and rank for a card num_cards times.
Printing:
Nothing
Positional arguments:
None
Returns:
cards_list -- a list of PlayingCard objects.
"""
#
# REPLACE WITH YOUR CODE.
#
return cards_list
def freq_count(cards_list):
"""Count every suit-rank combination.
Returns a dictionary whose keys are the card suit-rank and value is the
count.
Printing:
Nothing
Positional arguments:
cards_list -- A list of PlayingCard objects.
Returns:
card_freqs -- A dictionary whose keys are the single letter in the set
'd', 'c', 'h', 's' representing each card suit. The value for each key
is a list containing the number of cards at each rank, using the index
position to represent the rank. For example, {'s': [0, 3, 4, 2, 5]}
says that the key 's', for 'spades' has three rank 1's (aces), four
rank 2's (twos), two rank 3's (threes) and 5 rank 4's (fours). Index
position 0 is 0 since no cards have a rank 0, so make note.
"""
# DO NOT REMOVE BELOW
if type(cards_list) != list or \
list(filter(lambda x: type(x) != PlayingCard, cards_list)):
raise TypeError("cards_list is required to be a list of PlayingCard \
objects.")
# DO NOT REMOVE ABOVE
#
# REPLACE WITH YOUR CODE.
#
return card_freqs
def std_dev(counts):
"""Calculate the standard deviation of a list of numbers.
Positional arguments:
counts -- A list of ints representing frequency counts.
Printing:
Nothing
Returns:
_stdev -- The standard deviation as a single float value.
"""
# DO NOT REMOVE BELOW
if type(counts) != list or \
list(filter(lambda x: type(x) != int, counts)):
raise TypeError("counts is required to be a list of int values.")
# DO NOT REMOVE ABOVE
#
# REPLACE WITH YOUR CODE.
#
return _stdev
def print_stats(card_freqs):
"""Print the final stats of the PlayingCard objects.
Positional arguments:
card_freqs -- A dictionary whose keys are the single letter in the set
'dchs' representing each card suit. The value for each key is a list of
numbers where each index position is a card rank, and its value is its
card frequency.
You will probably want to call th std_dev function in somewhere in
here.
Printing:
Prints the statistic for each suit to the screen, see assignment page
for an example output.
Returns:
None
"""
# DO NOT REMOVE BELOW
if type(card_freqs) != dict or \
list(filter(lambda x: type(card_freqs[x]) != list, card_freqs)):
raise TypeError("card_freqs is required to be a list of int values.")
# DO NOT REMOVE ABOVE
#
# REPLACE WITH YOUR CODE.
#
def main():
cards = make_random_cards()
suit_counts = freq_count(cards)
print_stats(suit_counts)
if __name__ == "__main__":
main()
2 Your lab assignment requires the use of your solution from the Class lab. You will import your code as a module and use your PlayingCard class. The skeleton code includes the necessary import line, just provide your file by placing a copy of your lab_classes.py file in the same directory as your solution code for this lab.The docstrings inside this file describe what each function should do. See below link:
https://www.chegg.com/homework-help/questions-and-answers/s-task-s-code-please-correct-thank-def-main-class-playingcard-def-init-self-rank-suit-indi-q22730011
3 Replace and complete missing code wherever you see:: #
# REPLACE WITH YOUR CODE.
#
Do not change anything else.
Sample output:
Using the simple set of test, above, this is what you should see (your numbers will be different!):
Standard deviation for the frequency counts of each rank in suit:
Hearts: 3.2816 cards
Spades: 2.13411 cards
Clubs: 4.1730 cards
Diamonds: 1.2354 cards
Step by Step Solution
There are 3 Steps involved in it
Step: 1
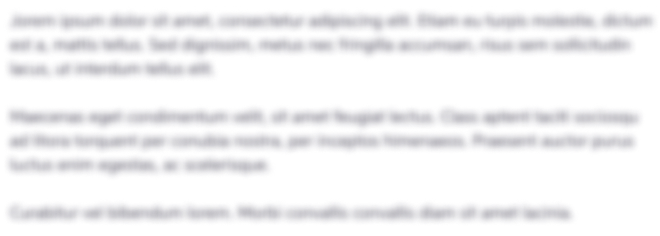
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started