Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In this lab, you will implement a few methods for Binary Search Tree ( BST ) . You are given the class for the Tree
In this lab, you will implement a few methods for Binary Search Tree BST You are given the class for the Tree node that supports generic types. As we demonstrated in the class discussion, you will add the following methods. We demonstrated how to implement the following methods.
Insert: insert a key value pair in the Binary Search Tree
Get: returns the value of a given key.
Inorder traversal: prints the key value pair from the left subtree, root, and right subtree.
Insert keyvalue pairs in BST if a key already exists update the value
public void insertKey key, Value value
Implement
Search BST for a given key
public Value getKey key
Implement search for a given key
return null;
public void inorderNode root
Implement Inorder Traversal
import java.util.Scanner;
public class BinarySearchTree, Value
private Node root; root of binary search tree
class Node
private Key key; sorted by key
private Value value; data stored in the node
private Node left, right; pointers to left and right subtrees
private int size; number of nodes in subtree
public NodeKey k Value v
this.key k;
this.value v;
Insert keyvalue pairs in BST if a key already exists update the value
public void insertKey key, Value value
Implement insertion of a keyvalue pair in a BST
Search BST for a given key
public Value getKey key
Implement search for a given key
return null;
public void inorderNode root
Implement Inorder Traversal
Bonus problem
public int size
return the number of keyvalue pairs nodes in the BST
public static void mainString args
Scanner input new ScannerSystemin;
BinarySearchTree bst new BinarySearchTree;
System.out.printlnEnter key int and value string pairs to end;
System.out.printlnFor example Phoenix";
whileinputhasNext
int key input.nextInt;
ifkey break;
String value input.next;
bstinsertkey value;
System.out.printlnSearch for key: ;
System.out.printlnbstget;
System.out.printlnInorder Traversal prints:";
bstinorderbstroot;
input.close;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
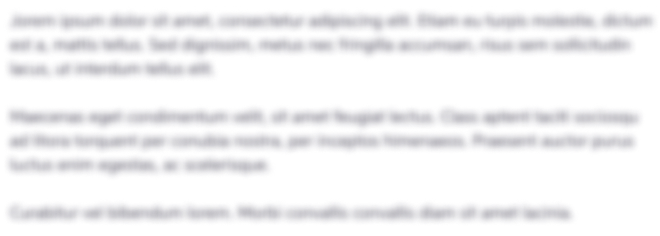
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started