Question
In this lab; you will start with a template script (below) and create a series of classes to satisfy the lab prompt. There is only
In this lab; you will start with a template script (below) and create a series of classes to satisfy the lab prompt. There is only one part to this lab; for details on grading criteria, see the rubric at the bottom of this page.
Bank Accounts (100 points)
- Define an Account class
- Define protected class variables to store the following
- Account holders name as string
- balance as float
- transaction counter as integer
- Define the following methods
- A constructor (__init__) that accepts the following values and populates appropriate class variables
- account holders name
- starting balance
- deposit(float)
- accepts a floating-point number and adds it to the balance
- increments the transaction counter
- returns the current balance
- withdraw(float)
- If the balance of the account is sufficient to withdraw funds; subtract amount from balance and return the new balance, increment the transaction counter
- If the balance in the account is insufficient, do not subtract the amount and return False, do not increment the transaction counter
- overload len()
- When an Account object is used as an argument to len(), return the transaction count
- overload str()
- When an Account object is passed to str(), return the account holders name
- overload the equivalence operator
- When your Account class is used with an equivalence operator "==" compare the balance in both accounts and return True if they are equal, False if they are not equal
- A constructor (__init__) that accepts the following values and populates appropriate class variables
- Define a CreditAccount class that inherits from Account
- Add the following private class variable
- limit
- rate
- Override the constructor to only accept an account name
- the starting balance of CreditAccount should always be 0
- Set the limit to 1000.00 and the rate to 1.24
- Override the deposit method
- Accept a float as a single argument
- Subtract the amount from balance and return the new balance
- If subtracting the amount results in a negative number; set the balance to 0.0
- do not increment the transaction counter
- return the new balance
- Override the withdraw function
- Accept a float as a single argument
- If the current account balance plus this new amount is less than limit
- multiply the amount by the rate and add the result to balance
- increment the transaction counter
- return the new balance
- If the balance plus this new amount is equal to or greater than limit
- return False
- do not increment the transaction counter
- Add the following private class variable
- Define a SavingsAccount
- Override the Deposit function
- On every deposit, multiply the amount by 1.05 before adding it to balance
- increment the transaction counter by 1
- return the new balance
- define a function called accrue
- does not take any arguments from the caller
- multiplies the balance by 1.05
- does not increment the transaction counter
- returns the new balance
- Override the Deposit function
- Define protected class variables to store the following
Template Script:
#!/usr/bin/python import math
# ==========================================================================
# YOUR SUBMISSION GOES HERE
# ==========================================================================
## DO NOT MODIFY BEYOND THIS POINT! ## Submissions with modifications beyond this line will be given a 0 score ## We will check... we have the technology :-) if __name__ == "__main__": myScore = 0
# Test base account acct = Account('Chelsey', 1024.32)
# test withdraw method overdraft if acct.withdraw(100000.00) == False: myScore += 5 else: print("ERROR: Account.withdraw(ammount) did not return False.")
# Test withdraw method balance OK if acct.withdraw(60.01) == 964.31: myScore += 5 else: print("ERROR: Value returned when attempting to make a withdrawal was unexpected")
# test deposit function if acct.deposit(36.50) == 1000.81: myScore += 5 else: print("ERROR: The deposit function on Account class returned an unexpected value")
# test transaction counter if len(acct) == 2: myScore += 5 else: print("ERROR: The transaction counter returned an unexpected result when using len()")
# Check toString() if str(acct) == 'Chelsey': myScore += 5 else: print("ERROR: Converting Account to a string had an unexpected resule")
# Test equivilance operator acct2 = Account('Sam', 1000.81) if acct == acct2: myScore += 5 else: print("ERROR: Equivilance op test #1 failed") acct2.withdraw(1000.00) if acct == acct2: print("ERROR: Equivilance op test #2 failed") else: myScore += 5
# Test the CreditAccount class acct = CreditAccount('Chelsey')
# Test inherited methods if str(acct) == 'Chelsey': myScore += 5 else: print("ERROR: When testing inherited methods for CreditAccount") # Test withdraw function if acct.withdraw(100000.00) == False: myScore += 5 else: print("ERROR: CreditAccount.withdraw did not return expected false") if acct.withdraw(100.00) == 124.00: myScore += 5 else: print("ERROR: CreditAccount.withdraw returned unexpected result")
# test deposit function if acct.deposit(100.00) == 24.00: myScore += 5 else: print("ERROR: CreditAccount.deposit returned unexpected result") # test transaction counter if len(acct) == 1: myScore += 5 else: print("ERROR: CreditAccount length was incorrect")
# test SavingsAccount acct1 = SavingsAccount('Hannah', 36.30) acct2 = SavingsAccount('Andrew', 156.33) if acct1.deposit(100.00) == 141.30: myScore += 5 else: print("ERROR: deposit for savings account had unexpected return")
# test accrue if acct1.accrue() == 148.365: myScore += 5 else: print("ERROR: deposit for savings account had unexpected return") print ("Grading complete: your score is %d out of 70" % (myScore) )
Step by Step Solution
There are 3 Steps involved in it
Step: 1
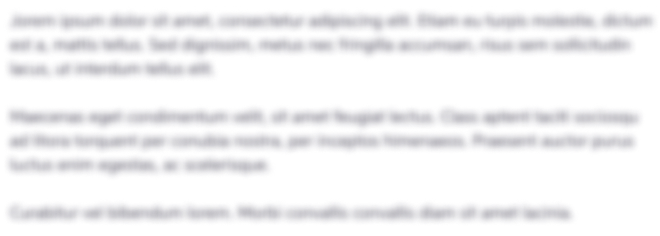
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started