Question
In this lab, you will write a simple stopwatch program that uses SysTick Interrupts to keep track of time. You will use polling to monitor
In this lab, you will write a simple stopwatch program that uses SysTick Interrupts to keep track of time. You will use polling to monitor the Start and Stop buttons on the MSP432 LaunchPad.The flowcharts for the main program and the SysTick ISR are shown in Figure 1. Your stopwatch should measure time to the hundredth of a second. The time will be displayed as seconds.hundredths. You do not have to convert the time to show minutes when seconds exceeds 59. Of course, hundredths should always be between 0 and 99. You must use global variables in order to keep track of seconds and hundredths. These can be individual variables or you can use a structure and have seconds and hundredths as members of the structure. In either case, these variables should be declared as volatile. The initialization block should include stopping the watchdog timer, properly configuring gpio pins for switch inputs and outputs to control the RGB LED, turning off all LEDs, and setting the SysTick period. Pushbutton S1 (P1.1) will be the Start button and pushbutton S2 (P1.4) will be the Stop button. You should not print to the console while your stopwatch is running because printf() disables interrupts, which will make your stopwatch timing inaccurate. Also, it is bad practice to print from an ISR since your ISR should be as fast as possible. Therefore your program will not display the running time on the console. Instead, the program will update the RGB LED color once per second when the stopwatch is running in order to provide a visual indication that SysTick interrupts are working and that the elapsed time is being updated.
This is code is going to upload onto a launchpad, from Code Composer Studio
Step by Step Solution
There are 3 Steps involved in it
Step: 1
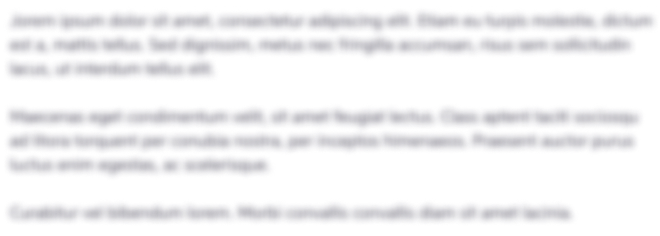
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started