Question
In this problem you will write a program that plays connect four. The program should start by asking the user for the dimensions of the
In this problem you will write a program that plays connect four. The program should start by asking the user for the dimensions of the board. Two players proceed by dropping coins into the columns. Coins fall to the bottom, and if a player has 4 coins in a row (horizontally, vertically, or diagonally), then that player wins the game. See http://www. connectfour.org/connect-4-online.php if you are unfamiliar with the game.
Skeleton code has been written for you, though you do not need to use it. If you use the skeleton code, you should define the body of main and the body of the functions in gameboard.c. For example output, see example*.txt.
Files to turn in: 1. connectfour.c
2. gameboard.c
3. gameboard.h
4. Makefile
We must be able to create the code on the command line using make.
(The appropriate codes for 1, 2, and 3, are provided, the make file must be created in a separate file.)
1. connectfour.c
#include
#include
#include
#include "gameboard.h"
int main() {
printf("Enter the number of rows and columns of your gameboard: ");
int rows, cols;
scanf("%d %d", &rows, &cols);
gameboard* board = gameboard_create(rows, cols);
// Homework TODO: complete this function
gameboard_destroy(board);
}
2. gameboard.c
#include "gameboard.h"
#include
#include
// allocates space for the gameboard and its squares
gameboard* gameboard_create(int numRows, int numCols) {
if (numRows < 3 || numCols < 3) {
fprintf(stderr, "Error: board must be at least 4x4 ");
exit(EXIT_FAILURE);
}
// Homework TODO: complete this function by adding code here
gameboard_initialize(result);
return result;
}
// deallocates space for the gameboard and its squares
void gameboard_destroy(gameboard* board) {
for (int i = 0; i < board->numRows; i++) {
free(board->squares[i]);
}
free(board->squares);
free(board);
}
// sets coinsInBoard to 0, state to STILL_PLAYING, and all squares to EMPTY
void gameboard_initialize(gameboard* board) {
// Homework TODO: define this function
}
// returns RED_COIN, YELLOW_COIN, or EMPTY depending on square (row, col)
square gameboard_square(const gameboard board, int row, int col) {
if (0 <= row && row < board.numRows && 0 <= col && col < board.numCols) {
return board.squares[row][col];
} else {
fprintf(stderr, "Error: board index %d %d out of bounds ", row, col);
exit(EXIT_FAILURE);
}
}
// attempts to insert coin into column col for player p
bool gameboard_insert_coin(gameboard* board, int col, player p) {
// Homework TODO: define this function
}
// prints the coins currently in the board
void gameboard_print(const gameboard board) {
for (int i = 0; i < board.numRows; i++) {
for (int j = 0; j < board.numCols; j++) {
switch (gameboard_square(board, i, j)) {
case EMPTY:
printf(" ");
break;
case RED_COIN:
printf("R ");
break;
case YELLOW_COIN:
printf("Y ");
break;
}
}
printf(" ");
}
for (int j = 0; j < board.numCols - 1; j++) {
printf("==");
}
printf("= ");
}
// returns true if STILL_PLAYING, false otherwise
bool gameboard_still_playing(const gameboard board) {
return board.state == STILL_PLAYING;
}
// returns state field
gamestate gameboard_state(const gameboard board) {
return board.state;
}
// check if game is over due to square (row, col) modification
void gameboard_check_square(gameboard* board, int row, int col) {
// Homework TODO: define this function
}
// check horizontal strips containing square (row, col)
void gameboard_check_square_horizontal(gameboard* board, int row, int col) {
// Homework TODO: define this function
}
// check vertical strips containing square (row, col)
void gameboard_check_square_vertical(gameboard* board, int row, int col) {
// Homework TODO: define this function
}
// check diagonal strips containing square (row, col)
void gameboard_check_square_diagonal(gameboard* board, int row, int col) {
// Homework TODO: define this function
}
// changes state to RED_WINS or YELLOW_WINS
void gameboard_declare_winner(gameboard* board, square color) {
// Homework TODO: define this function
}
3. gameboard.h (for your reference)
#ifndef GAMEBOARD_H
#define GAMEBOARD_H
#include
typedef enum {RED_WINS, YELLOW_WINS, TIE, STILL_PLAYING} gamestate;
typedef enum {RED_PLAYER, YELLOW_PLAYER} player;
typedef enum {EMPTY, RED_COIN, YELLOW_COIN} square;
typedef struct {
int numRows, numCols;
int coinsInBoard;
gamestate state;
square** squares;
} gameboard;
// allocates space for the gameboard and its squares
gameboard* gameboard_create(int numRows, int numCols);
// deallocates space for the gameboard and its squares
void gameboard_destroy(gameboard* board);
// sets coinsInBoard to 0, state to STILL_PLAYING, and all squares to EMPTY
void gameboard_initialize(gameboard* board);
// returns RED_COIN, YELLOW_COIN, or EMPTY depending on square (row, col)
square gameboard_square(const gameboard board, int row, int col);
// attempts to insert coin into column col for player p
bool gameboard_insert_coin(gameboard* board, int col, player p);
// prints the coins currently in the board
void gameboard_print(const gameboard board);
// returns true if STILL_PLAYING, false otherwise
bool gameboard_still_playing(const gameboard board);
// returns state field
gamestate gameboard_state(const gameboard board);
// check if game is over due to square (row, col) modification
void gameboard_check_square(gameboard* board, int row, int col);
// check horizontal strips containing square (row, col)
void gameboard_check_square_horizontal(gameboard* board, int row, int col);
// check vertical strips containing square (row, col)
void gameboard_check_square_vertical(gameboard* board, int row, int col);
// check diagonal strips containing square (row, col)
void gameboard_check_square_diagonal(gameboard* board, int row, int col);
// changes state to RED_WINS or YELLOW_WINS
void gameboard_declare_winner(gameboard* board, square color);
#endif
the following are examples of what should happen if the code is correct.
Ex 1:
Enter the number of rows and columns of your gameboard: 4 4
======= Enter column for red coin: 0
R ======= Enter column for yellow coin: 0
Y R ======= Enter column for red coin: 1
Y R R ======= Enter column for yellow coin: 1
Y Y R R ======= Enter column for red coin: 2
Y Y R R R ======= Enter column for yellow coin: 2
Y Y Y R R R ======= Enter column for red coin: 3
Y Y Y R R R R ======= Game over: red wins.
Ex. 2:
Enter the number of rows and columns of your gameboard: 4 4
======= Enter column for red coin: 0
R ======= Enter column for yellow coin: 1
R Y ======= Enter column for red coin: 0
R R Y ======= Enter column for yellow coin: 1
R Y R Y ======= Enter column for red coin: 0
R R Y R Y ======= Enter column for yellow coin: 1
R Y R Y R Y ======= Enter column for red coin: 0 R R Y R Y R Y ======= Game over: red wins.
Ex 3:
Enter the number of rows and columns of your gameboard: 6 8
=============== Enter column for red coin: 3
R =============== Enter column for yellow coin: 2
Y R =============== Enter column for red coin: 5
Y R R =============== Enter column for yellow coin: 3
Y Y R R =============== Enter column for red coin: 4
Y Y R R R =============== Enter column for yellow coin: 6
Y Y R R R Y =============== Enter column for red coin: 4
Y R Y R R R Y =============== Enter column for yellow coin: 4
Y Y R Y R R R Y =============== Enter column for red coin: 5
Y Y R R Y R R R Y =============== Enter column for yellow coin: 6
Y Y R R Y Y R R R Y =============== Enter column for red coin: 5
Y R Y R R Y Y R R R Y =============== Enter column for yellow coin: 5
Y Y R Y R R Y Y R R R Y =============== Game over: yellow wins.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
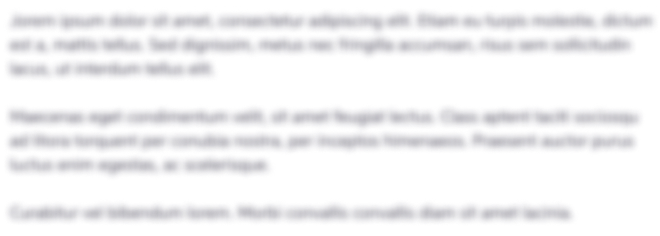
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started