Question
In this problem you'll get to use one of Java's random number generators, java.util.Random (see the docs for Random). This class generates a random double
In this problem you'll get to use one of Java's random number generators, java.util.Random (see the docs for Random). This class generates a random double precision number uniformly distributed in the range [0.0 ... 1.0). That is, the numbers go from 0 to 1, including 0 but excluding 1. It is a common need in Java programming to generate a random integer number that's uniformly distributed in the range of [0 ... n), such as we saw in the dice rolling question a few weeks ago for n=6. The code to do this looks like:
double randomDouble = n * randomGenerator.nextDouble();
Do the following steps:
Create a class FillRandom.
Do your work in the fillRandom() method.
Prompt the user with "Enter a positive integer:".
Set up a Scanner to read from the keyboard and take in a positive integer randomSize from the user.
Allocate a double array whose length is 1,000,000.
Loop over the elements of the array, assigning array[k] the next double random number between 0 and randomSize. You will have to scale up the java.util.Random.nextRandom() value, which is in the range [0...1). You want it to be in the range [0...randomSize). So you must multiply it by randomSize, as shown in code above.
Now loop over the array again, computing the min, max and average of its values. You may want to use the Math.min() and Math.max() methods from java.lang.Math. (SAVE YOUR CODE before clicking on this link)
Print out the min value with "Min = " and the minimum
Print out the max value with "Max = " and the maximum
Print out the average with "Average = " and the average
Notice that the minimum over many random numbers in [0...n) is close to zero, the max is close to n, and the average is close to n/2.
In order to pass tests, use the following format:
Enter a positive integer: Min = <> Max = < > Average = < > Arrange it has: // TODO: Add necessary import statements for Scanner and Random. // Class to do array assignment and reading. public class FillRandom { private static final int constArraySize = 1000000; public static void fillRandom(Random randomGenerator) { System.out.println("Enter a positive integer:"); // TODO: Read a positive integer from the keyboard. // TODO: Allocate an array of constArraySize doubles. // TODO: Fill array[k] with random numbers in [0...randomSize). // TODO: Loop over the array again, computing min, max and average. // Print out the values. (Hint: these statements make use of variables you should declare above.) System.out.println("Min = " + min); System.out.println("Max = " + max); System.out.println("Average = " + average); } public static void main(String[] args) { // DO NOT MODIFY the main() method. Random randomGenerator; int seed = 314159265; if (args.length > 0) { seed = Integer.parseInt(args[0]); } randomGenerator = new Random(seed); // Call the student's function to do the rest of the work. fillRandom(randomGenerator); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
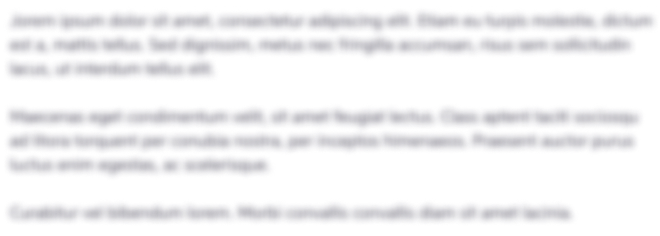
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started