Question
In this program, you need to complete these functions: 1. peak(): return the top of the element in the stack; return 0 and print out
In this program, you need to complete these functions:
1. peak(): return the top of the element in the stack; return 0 and print out an error if stack is empty; (You can not use popping function)
2. popdup(): duplicate the top element in the stack;
3. popswap(): swap the top two elements in the stack;
4. clear(): clear the stack; You can add code in main function to test your functions but please dont touch other parts of the code.
The program :
/****************************************************************************** In this example code we construct a calculator that uses postfix notation.
Example: (1-2)*(4+5) -> 1 2 - 4 5 + *
Pseudocode:
While (next operator or operand is not EOF) if (number) push it else if (operator) pop operands do operation push result else if (newline) pop and print top of stack else error
*******************************************************************************/ #include
#define MAXOP 100 #define NUMBER '0'
int getop(char []); void push (double); double pop(void); double peak(); void popdup(); void popswap(); void clear();
int main() { int type; double op2; char s[MAXOP]; //////////////////////////////////////////////// //test new functions push(10); push(11); printf("peak:\t%f ", peak()); printf("peak:\t%f ", peak()); popswap(); printf("popswap(), peak:\t%f ", peak()); popdup(); for (int k=0; k<3; ++k) printf("pop:\t%f ", pop()); //////////////////////////////////////////////// while ((type=getop(s)) != EOF){ switch(type){ case NUMBER: push(atof(s)); break; case '+': push(pop() + pop()); break; case '*': push(pop() * pop()); break; case '-': op2 = pop(); push(pop() - op2); break; case '/': op2= pop(); if (op2 != 0.0) push(pop()/op2); else printf("error: zero divisor "); break; case ' ': printf("\t%.8g ", pop()); break; default: printf("error: unknown command %s ", s); break; } } // ADD TEST CODE HERE FOR ALL THREE FUNCTIONS
return 0; }
#define MAXVAL 100 int sp =0; double val[MAXVAL];
void push(double f){ if (sp < MAXVAL) val[sp++]=f; else printf("error: stack full, can't push %g ", f); }
double pop(){ if (sp>0) return val[--sp]; else{ printf("error: stack empty "); return 0.0; } }
/******************************/ // returns the top of the stack double peak(){ // YOUR CODE HERE return 0.0; }
// duplicate the element at the top of the stack, at the top of the stack void popdup(){ // YOUR CODE HERE return; }
//swap the top two elements of the stack void popswap(){ // YOUR CODE HERE return; }
void clear(){ // YOUR CODE HERE return; } /******************************/
#include
int getch(void); void ungetch(int);
/* gets the next operator or operand */ int getop(char s[]){ int i, c; while( (s[0] = c = getch()) == ' ' || c == '\t') ; s[1]='\0'; if(!isdigit(c) && c != '.') return c; i = 0; if(isdigit(c)) while (isdigit(s[++i] = c = getch())) ; if ( c == '.') while (isdigit(s[++i] = c = getch())) ; s[i] = '\0'; if (c != EOF) ungetch(c); return NUMBER; }
#define BUFSIZE 100
char buf[BUFSIZE]; int bufp =0; /* get the next character, either from standard input or from the buffer (of character's that we placed 'back on' the buffer) */ int getch(void){ return (bufp > 0) ? buf[--bufp] : getchar(); }
/*populate the local buffer with characters you meant to 'put back' b/c you read too far*/ void ungetch(int c){ if (bufp >= BUFSIZE) printf("ungetch: too many characters "); else buf[bufp++] = c; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
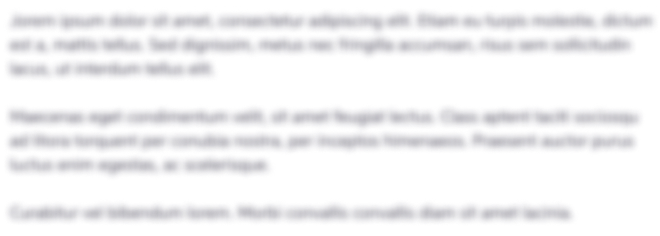
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started