Question
In this programming assignment, you will write a client ping program in Java based on UDP. Your client will do the following: send a simple
In this programming assignment, you will write a client ping program in Java based on UDP.
Your client will do the following:
-
send a simple ping message to a server, receive a corresponding pong message back from the server.
-
determine the delay between when the client sent the ping message and received the pong message. This delay is called the Round Trip Time (RTT).
-
your ping program is to send 10 ping messages to the target server over UDP. For each message, your client is to determine and print the RTT when the corresponding pong message is returned.
*Because UDP is an unreliable protocol, a packet sent by the client or server may be lost. For this reason, the client cannot wait indefinitely for a reply to a ping message. You should have the client wait up to one second for a reply from the server; if no reply is received, the client should assume that the packet was lost and print a message accordingly. (set a timer)
In this assignment, you will be given the complete code for the Server(No need to change anything in Server Class) & Client Class(this is what you will work on it) (available via this link).
Your job is to write the client code, which will be very like the server code. It is recommended that you first study carefully the server code.
Note:
Due Date: Check each Part
King Saud University CCIS- SWE Dept.- Spring 2019 [CEN303]
*You can then write your client code, liberally cutting and pasting lines from the server code with some functionalities to do the required tasks.
* To calculate RTT, you may use your system clock (system.nanotime();) to calculate the RTT.
the server code is :
9 import java.net.DatagramPacket; 10 import java.net.DatagramSocket; 11 import java.net.InetAddress; 12 13 public class Server { 14 15 16 17 public static void main(String args[]) throws Exception { 18 // create a server socket. 19 DatagramSocket serverSocket = new DatagramSocket(9999); // you can use any port number that is not publicly reserved 20 // set up the socket buffer for receiving data. 21 byte[] receiveData = new byte[1024]; 22 byte[] sendData = new byte[1024]; 23 24 // communication loop. 25 while (true) { 26 // assign the socket buffers as Datagram Packet. 27 DatagramPacket receivePacket = new DatagramPacket(receiveData, receiveData.length); 28 serverSocket.receive(receivePacket); 29 30 InetAddress IPAddress = receivePacket.getAddress(); 31 int port = receivePacket.getPort(); 32 // to send and ACK of receiving data back to the client. 33 sendData = "ACK".getBytes(); 34 DatagramPacket sendPacket = new DatagramPacket(sendData, sendData.length, IPAddress, port); 35 serverSocket.send(sendPacket); 36 37 } 38 39 } 40 }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
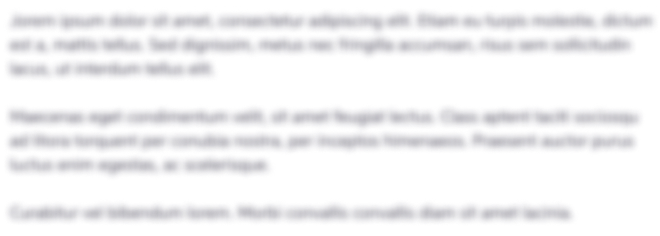
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started