Question
In this project I am importing a txt file full of numbers and using the directions below I will make the code. ... below the
In this project I am importing a txt file full of numbers and using the directions below I will make the code.
... below the directions I have posted how far I have come... but beyond that I am lost everything I try has become messy or is riddeled with errors. Can someone help.
Implement the insertion sort algorithm
public static void insertionSort(double[] a, int n)
// sorts the items in an array into ascending order
// Precondition: a is an array of n items
// Postcondition: a is sorted into ascending order
Implement the selection algorithm presented in the lecture:
public static int selectionSort(double [] a, int n) // sorts the items in an array into ascending order
// Precondition: a is an array of n items
// Postcondition: a is sorted into ascending order
Add static integers for counters to the class which will count the number of comparisons that are made by selectionSort and insertionSort
Read the file numbers.txt into an array of doubles. Then close the file. Use insertion sort to sort the array and display the number of comparisons needed to sort the data.
Then, reopen the file and use selection sort to sort the array once more time. Display the number of comparison for that algorithm.
Now that the data has been sorted (twice), reset your counters and sort the data again, counting the number of comparisons to sort data which is already ordered.
Finally, reverse the data in the array so it is in descending order each time for the next two runs. Once again reset your counters, and run the two algorithms and count and display the number of comparisons.
import java.util.*;
import java.io.File;
import java.io.IOException;
public class Sorts {
public static void main( String args[] ) throws IOException{
int MAX = 12000;
double [] array = new double [ MAX ];
File infile = new File ("Numbers.txt");
Scanner input = new Scanner(infile);
int i = 0;
while ( input.hasNext()){
array [ i ] = input.nextDouble();
}
input.close();
}// end of method
private static int count = 0 ;
public static void insertionSort ( double array [] , int n ){
for (int j = 1; j < n; j++) {
double key = array[j];
int i = j-1;
while ( (i > -1) && ( array [i] > key ) ) {
array [i+1] = array [i];
i--;
}
array[i+1] = key;
}
System.out.println(Arrays.toString(array));
}
public static double[] selectionSort ( double array [], int n){
for (int i = 0; i < n - 1; i++) {
int index = i;
for (int j = i + 1; j < n; j++)
if (array[j] < array[index])
index = j;
double smallerNumber = array[index];
array[index] = array[i];
array[i] = smallerNumber;
}
return array;
}
} //end of class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
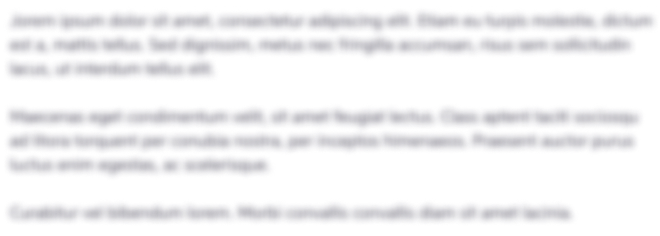
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started