Question
In this project, we will make up a banking system. You are an employee of MadeUp Banking. Your job is to manage multiple bank accounts.
In this project, we will make up a banking system. You are an employee of MadeUp Banking. Your job is to manage multiple bank accounts. Here, you would create new accounts, deposit to an account, withdraw from an account, delete account, sort the accounts, or do inspection on one or all bank accounts. In order to do this job, you would need the tool for each of those items. The bank maintains a list of accounts. This list has to be available as long as the bank is in business.
Write a program in C++ to run the MadeUp Banking business. As state above, the list of account has to be available as long as you run the program. This means the list of the account has to be initialized in the main() function. Write a function for each of the items above. You will call these functions to perform any task on the bank accounts. Each account will have account number, name (last, first), and balance. The header file, function file, and main file are as the sample below. All functions are of type void. You will fill in the necessary function parameters.
In order to do this project, you would need to understand pointers, functions, and vectors among other basic C++ knowledge. You will follow the exact requirement and format of the program. Any unnecessary changes will result in points taken off. REFERENCE BELOW
In this project, you need the following essential functions:
menu() - this function displays all the available options that a bank clerk can perform.
The menu should be display every time for the new selection. The format as follow:
Welcome to MadeUp Banking. Select options below: 1. Make new account.
2. Display all accounts. 3. Deposit to an account. 4. Withdraw from an account. 5. Print account. 6. Delete an account. 7. Quit.
Selection:
makeAccount() - this function create the new bank account. The bank account has account number, first name, last name, and account balance. The account number is a 4-digit number. It can be generated randomly. Two bank accounts cannot have the same account number. When generating the new bank account number, make sure that it is not existed in the current list of bank accounts. The user can input first name, last name, and the starting balance. The starting balance is a double number. The format as follow:
Creating bank account number 9205 Enter first name: John Enter last name: Smith Enter starting balance: 320.56
printAllAccounts() - this function print out all the current accounts. The format as follow:
Account number: 9119 Balance: 124.56 Last name: Doe First name: John
Account number: 9205 Balance: 320.56 Last name: Smith First name: John
Account number: 9305 Balance: 562.34 Last name: Jane First name: Mary
printAccount() - this function print out only one account. The function prompts for the account number from the user. If the account exists, the function print out the bank account with the format as before. If the account does not exist, the function print out the a prompt that the account does not exist.
depositAccount() - this function deposit an amount to the bank account. The function prompt the user for bank account and balance to be deposited. After the deposit, the bank account should be updated with the balance. You can use the print out function to check this. If the account does not exist, display a message that the account does not exist. The format as follow:
Enter account number for deposit: 9119 Enter amount to be deposited: 23.45
withdrawAccount() - this function withdraw an amount from the bank account. The function prompts the user for bank account and balance to be withdrawn. After the withdrawal, the bank account should be updated with the balance. You can use the print out function to check this. If the account does not exist, display a message that the account does not exist. The format as follow:
Enter account number for withdrawal: 9119 Enter amount to be withdrawn: 56.78
deleteAccount() - this function delete an account. The function prompts the user for the account number to be deleted. After deletion, the list of bank accounts should be updated. You can use the the print out function to check this. If the account does not exist, display a message that the account does not exist. The format as follow:
Enter account number to be deleted: 9119
sortAccounts() - this function sort the bank account by the account numbers. Use this function after the makeAccount() function to sort the accounts after making new account.
validInput() - this is a general function. This function check if the user input is valid. This function only checks the input number. The number could be the account number (for printing, deposit, or withdraw an account), or amount input (starting balance, deposit, or withdraw). Write this function in a way that it can be reused for all the function that needs inputting numbers. This function should be used with makeAccount(), printAccount(), depositAccount(),withdrawAccount(), and deleteAccount() functions.Thefollowings are the few examples.
Creating new account:
Creating bank account number 2717 Enter first name: Jane Enter last name: Smith Enter starting balance: -12
Enter positive number: ewq
Invalid input. Enter new number: 12
Deposit to an account (1):
Enter account number for deposit: 2717 Enter amount to be deposit: -12 Enter positive number: rew Invalid input. Enter new number: 12
Deposit to an account (2):
Enter account number for deposit: eqwr
Invalid input. Enter new number: 1234 Account number not found.
B. Program Structure
Create a vector of the bank accounts. Each element of the vector will have all the account information. To do this, you would have to use data structure. The keyword struct in C++ will make a data structure. Inside the data structure, you will define all of the account information. The following is an example:
struct Account{ int accountNumber;
string lastName; string firstName; double accountBalance;
}; vectorbankAccounts;
The code snippet above defines data structure type Account and a vector name bankAccounts of type Account. Each element of the vector will have member accountNumber, lastName, firstName, and accountBalance.
The vector should be defined in the main() function. This means that as long as the program running (the bank is operating), we have the list of bank accounts. This should not be the global variable. The program should be running until terminated by the user.
In order to update the information of bank accounts in the vector, you would have to pass by reference to the functions. Another option is pass by pointer. You can explore this option if desire. Because the data in the vector is of the special type, you have to make a template for the typename. Template allows you to write functions independent of the data type being passed to the functions. The example of a prototype function as follow:
templatevoid makeAccount(vector &);
In the code snippet above, we create a new typename typeStruct using the keyword template and typename. typeStruct is just a random typename. You can name it to some other names. This typename will now take whichever type being passed to the function. In our case, were passing the vector of data structure to the function. The typename will take the type Account as we defined in the main() function.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
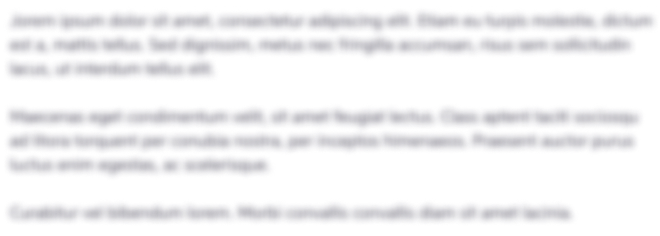
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started