Question
In this project, you will be practicing the use of Java lists and enum types by im-plementing a command line Blackjack game. Using the classes
In this project, you will be practicing the use of Java lists and enum types by im-plementing a command line Blackjack game. Using the classes Card and CardDeck, which will be provided, you will implement a simulator that plays a simplified hand of Blackjack against a computer dealer. The program will terminate when either you win, the dealer wins, or you tie.
Part 1 - Blackjack simulator
You have been given two classes from the textbook which have been slightly modified to use Java ArrayLists instead of the textbook implementations of lists (you will also use Java library lists in your project if your implementation requires them). Your job is to simulate a hand of Blackjack according to the following rules. Implement your program in the main() method of a class Blackjack.java. You will NOT need to modify Card or CardDeck:
1. The goal of the game is to get the sum of cards in your hand higher than the dealer's without going over 21. Each card is worth the rank value, where face cards are worth 10 and aces are worth either 1 or 11.
2. The game begins by shuffling and dealing two cards to you and two cards to the dealer. The program should reveal both cards in your hand but only the first card of the dealer's hand (the other card, called the hole" card, remains hidden).
3. After the initial cards are dealt, you will repeatedly ask the player if they would like to hit" or stay". If the player hits, the program deals them another card and repeats the query. If the player stays, this phase of the game ends. If, when the player asks to hit, the sum value of the cards in their hand exceeds 21, the game immediately ends and the player loses.
4. Once the player stays and has a hand of 21 or less, the dealer plays their hand. The rules for the dealer are that they must hit" until the sum value in their hand is greater than or equal to 17.
5. If the player's hand is greater than the dealer's OR if the dealer goes over 21, the player wins. If the dealer's hand is greater than the player's the dealer wins. If both hands are equal, the game is a tie.
Here are some tips to help you with your project:
1. Implement your code in phases and test before moving on to the next phase
2. Print out the the player's hand and the value of the hand every time it changes.
3. Clearly comment each phase of the game so that I will be able to award full credit for your last project
4. A simple way to terminate your program immediately is with the System.exit(0) command. The 0" input parameter indicates normal program termination.
5. You will have to use the enum construction as implemented in the class Card. Remember, these enumerated types can be treated just like objects, with method calls on the enum to learn more information about it.
6. Because aces may be 1 or 11, determining the value of a hand will be challenging. You should implement a separate method countHand() that takes your current hand and calculates the highest possible sum that does not exceed 21 (if all possible sums exceed 21, return the lowest, you've gone over). Think carefully about how many possible combinations of aces exist and how many you need to check in countHand().
Submit Blackjack.java and please provide screen shot of compiled code.
__________________________________________
Card Class
import javax.swing.ImageIcon;
public class Card implements Comparable { public enum Rank {Two, Three, Four, Five, Six, Seven, Eight, Nine, Ten, Jack, Queen, King, Ace}
public enum Suit {Club, Diamond, Heart, Spade}
protected final Rank rank; protected final Suit suit; protected ImageIcon image; Card(Rank rank, Suit suit, ImageIcon image) { this.rank = rank; this.suit = suit; this.image = image; }
public Rank getRank() { return rank; } public Suit getSuit() { return suit; } public ImageIcon getImage() {return image;}
@Override public boolean equals(Object obj) // Returns true if 'obj' is a Card with same rank // as this Card, otherwise returns false. { if (obj == this) return true; else if (obj == null || obj.getClass() != this.getClass()) return false; else { Card c = (Card) obj; return (this.rank == c.rank); } }
public int compareTo(Card other) // Compares this Card with 'other' for order. Returns a // negative integer, zero, or a positive integer as this object // is less than, equal to, or greater than 'other'. { return this.rank.compareTo(other.rank); }
@Override public String toString() { return suit + " " + rank; } }
_______________________________________________________
CardDeck class
import java.util.Random; import chapter.lists.ABList; //see class details below import java.util.Iterator; import javax.swing.ImageIcon;
public class CardDeck { public static final int NUMCARDS = 52; protected ABList deck; protected Iterator deal; public CardDeck() { deck = new ABList(NUMCARDS); ImageIcon image; for (Card.Suit suit : Card.Suit.values()) for (Card.Rank rank : Card.Rank.values()) { image = new ImageIcon("support/cards/" + suit + "_" + rank + "_RA.gif"); deck.add(new Card(rank, suit, image)); } deal = deck.iterator(); }
public void shuffle() // Randomizes the order of the cards in the deck. // Resets the current deal. { Random rand = new Random(); // to generate random numbers int randLoc; // random location in card deck Card temp; // for swap of cards for (int i = (NUMCARDS - 1); i > 0; i--) { randLoc = rand.nextInt(i); // random integer between 0 and i - 1 temp = deck.get(randLoc); deck.set(randLoc, deck.get(i)); deck.set(i, temp); } deal = deck.iterator(); } public boolean hasNextCard() // Returns true if there are still cards left to be dealt; // otherwise, returns false. { return (deal.hasNext()); } public Card nextCard() // Precondition: this.hasNextCard() == true // // Returns the next card for the current 'deal'. { return deal.next(); } }
________________________________
ABList
import java.util.Iterator;
public class ABList implements ListInterface { protected final int DEFCAP = 100; // default capacity protected int origCap; // original capacity protected T[] elements; // array to hold this lists elements protected int numElements = 0; // number of elements in this list
// set by find method protected boolean found; // true if target found, otherwise false protected int location; // indicates location of target if found
public ABList() { elements = (T[]) new Object[DEFCAP]; origCap = DEFCAP; }
public ABList(int origCap) { elements = (T[]) new Object[origCap]; this.origCap = origCap; }
protected void enlarge() // Increments the capacity of the list by an amount // equal to the original capacity. { // Create the larger array. T[] larger = (T[]) new Object[elements.length + origCap]; // Copy the contents from the smaller array into the larger array. for (int i = 0; i < numElements; i++) { larger[i] = elements[i]; } // Reassign elements reference. elements = larger; }
protected void find(T target) // Searches list for an occurence of an element e such that // e.equals(target). If successful, sets instance variables // found to true and location to the array index of e. If // not successful, sets found to false. { location = 0; found = false;
while (location < numElements) { if (elements[location].equals(target)) { found = true; return; } else location++; } }
public boolean add(T element) // Adds element to end of this list. { if (numElements == elements.length) enlarge(); elements[numElements] = element; numElements++; return true; }
public boolean remove (T target) // Removes an element e from this list such that e.equals(target) // and returns true; if no such element exists, returns false. { find(target); if (found) { for (int i = location; i <= numElements - 2; i++) elements[i] = elements[i+1]; elements[numElements - 1] = null; numElements--; } return found; } public int size() // Returns the number of elements on this list. { return numElements; }
public boolean contains (T target) // Returns true if this list contains an element e such that // e.equals(target); otherwise, returns false. { find(target); return found; }
public T get(T target) // Returns an element e from this list such that e.equals(target); // if no such element exists, returns null. { find(target); if (found) return elements[location]; else return null; }
public boolean isEmpty() // Returns true if this list is empty; otherwise, returns false. { return (numElements == 0); }
public boolean isFull() // Returns false - the list is unbounded. { return false; }
public void add(int index, T element) // Throws IndexOutOfBoundsException if passed an index argument // such that index < 0 or index > size(). // Otherwise, adds element to this list at position index; all current // elements at that index or higher have 1 added to their index. { if ((index < 0) || (index > size())) throw new IndexOutOfBoundsException("Illegal index of " + index + " passed to ABList add method. "); if (numElements == elements.length) enlarge(); for (int i = numElements; i > index; i--) elements[i] = elements[i - 1];
elements[index] = element; numElements++; } public T set(int index, T newElement) // Throws IndexOutOfBoundsException if passed an index argument // such that index < 0 or index >= size(). // Otherwise, replaces element on this list at position index with // newElement and returns the replaced element. { if ((index < 0) || (index >= size())) throw new IndexOutOfBoundsException("Illegal index of " + index + " passed to ABList set method. "); T hold = elements[index]; elements[index] = newElement; return hold; } public T get(int index) // Throws IndexOutOfBoundsException if passed an index argument // such that index < 0 or index >= size(). // Otherwise, returns the element on this list at position index. { if ((index < 0) || (index >= size())) throw new IndexOutOfBoundsException("Illegal index of " + index + " passed to ABList get method. "); return elements[index]; }
public int indexOf(T target) // If this list contains an element e such that e.equals(target), // then returns the index of the first such element. // Otherwise, returns -1. { find(target); if (found) return location; else return -1; } public T remove(int index) // Throws IndexOutOfBoundsException if passed an index argument // such that index < 0 or index >= size(). // Otherwise, removes element on this list at position index and // returns the removed element; all current elements at positions // higher than that index have 1 subtracted from their position. { if ((index < 0) || (index >= size())) throw new IndexOutOfBoundsException("Illegal index of " + index + " passed to ABList remove method. ");
T hold = elements[index]; for (int i = index; i < numElements-1; i++) elements[i] = elements[i + 1]; elements[numElements-1] = null; numElements--; return hold; }
public Iterator iterator() // Returns an Iterator over this list. { return new Iterator() { private int previousPos = -1;
public boolean hasNext() // Returns true if the iteration has more elements; otherwise returns false. { return (previousPos < (size() - 1)) ; } public T next() // Returns the next element in the iteration. // Throws NoSuchElementException - if the iteration has no more elements { if (!hasNext()) throw new IndexOutOfBoundsException("Illegal invocation of next " + " in ABList iterator. "); previousPos++; return elements[previousPos]; }
public void remove() // Removes from the underlying representation the last element returned // by this iterator. This method should be called only once per call to // next(). The behavior of an iterator is unspecified if the underlying // representation is modified while the iteration is in progress in any // way other than by calling this method. { for (int i = previousPos; i <= numElements - 2; i++) elements[i] = elements[i+1]; elements[numElements - 1] = null; numElements--; previousPos--; } }; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
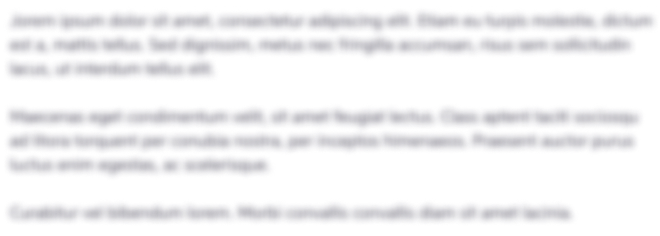
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started