Question
In this project you will create a program that will calculate the quarterly sales for a company. You will be using two arrays to store
In this project you will create a program that will calculate the quarterly sales for a company. You will be using two arrays to store the quarterly sales figures and the quarterly names Q1, Q2, Q3 and Q4. Since you will be dealing with money you will need to use a double datatype. The program will prompt the user for the quarterly sales figures for each quarter, Q1 through Q4. Here are some of the main variables you will need to create for the program: Quarter[4] Is a string array that holds quarterly names Q1, Q2, Q3, and Q4. quarterlySales[4] Is an array that holds the quarterly sales for the year Once you have gotten the quarterly sales numbers you will pass the quarterlySales array to the following functions: Function that will sum the quarterly sales and return the yearly sales Function that will average the quarterly sales and return the average quarterly sales Function that will return the index (subscript) of the quarter with the highest sales amount Function that will return the index (subscript) of the quarter with the lowest sales amount Function that will sort the array in ascending (lowest to highest) order 1, 2, 3, etc. You should display: Total quarterly sales for the year Average quarterly sales for the year Quarter with the highest sales and the sales amount Quarter with the lowest sales and the sales amount Quarter sales sorted from lowest to highest. Display the quarter and the amount Formatting the output: You will need to format all sales with a $ and 2 decimal points precision.
Since you will be sorting a parallel array I will give you that code: void sortAry(double qSales[], int size, string qName[]) { bool swap; double tempSales; string tempDays;
do { swap = false; for (int count = 0; count < (size - 1); count++) { if (qSales[count] > qSales[count + 1]) { tempSales = qSales[count]; qSales[count] = qSales[count + 1]; qSales[count + 1] = tempSales;
tempDays = qName[count]; qName[count] = qName[count + 1]; qName[count + 1] = tempDays;
swap = true; } } } while (swap); } This project covers methods you have learned in chapters 2 through 8.
Program Output: This program calculates total quarterly sales.
Please enter the gross sales received on Q1: $123652.36 Please enter the gross sales received on Q2: $356242.58 Please enter the gross sales received on Q3: $324562.85 Please enter the gross sales received on Q4: $120253.25
The total quarterly sales for the year were: $924711.04 The average quarterly sales for the year were: $231177.76 The quarter with the highest sales amount was Q2 with a sales total of $356242.58 The quarter with the lowest sales amount was Q4 with a sales total of $120253.25
Quarterly sales sorted from lowest to highest: Q4 had gross sales of $120253.25 Q1 had gross sales of $123652.36 Q3 had gross sales of $324562.85 Q2 had gross sales of $356242.58 Press any key to continue . . . |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
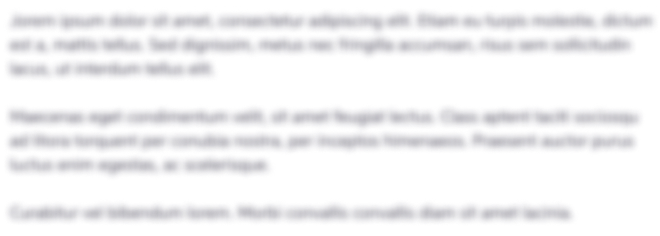
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started