Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In this project you will work on a program that will help visualize a bar graph chart of Air Quality Health Index [1] data,
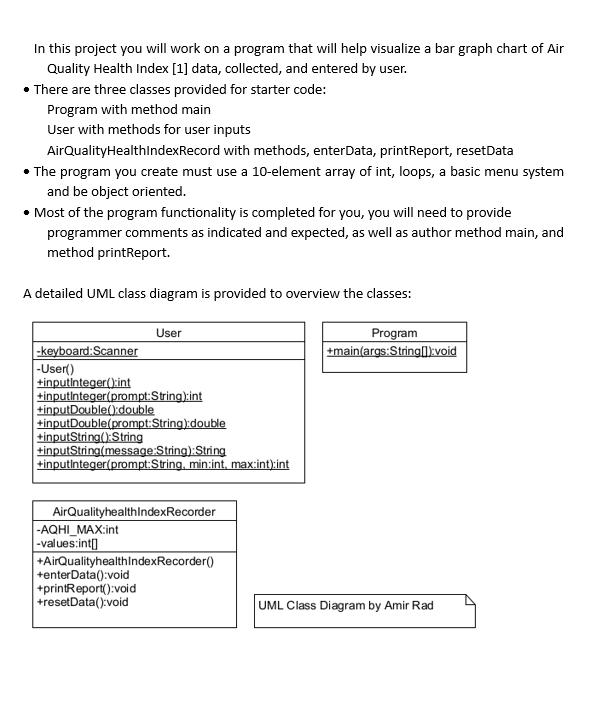
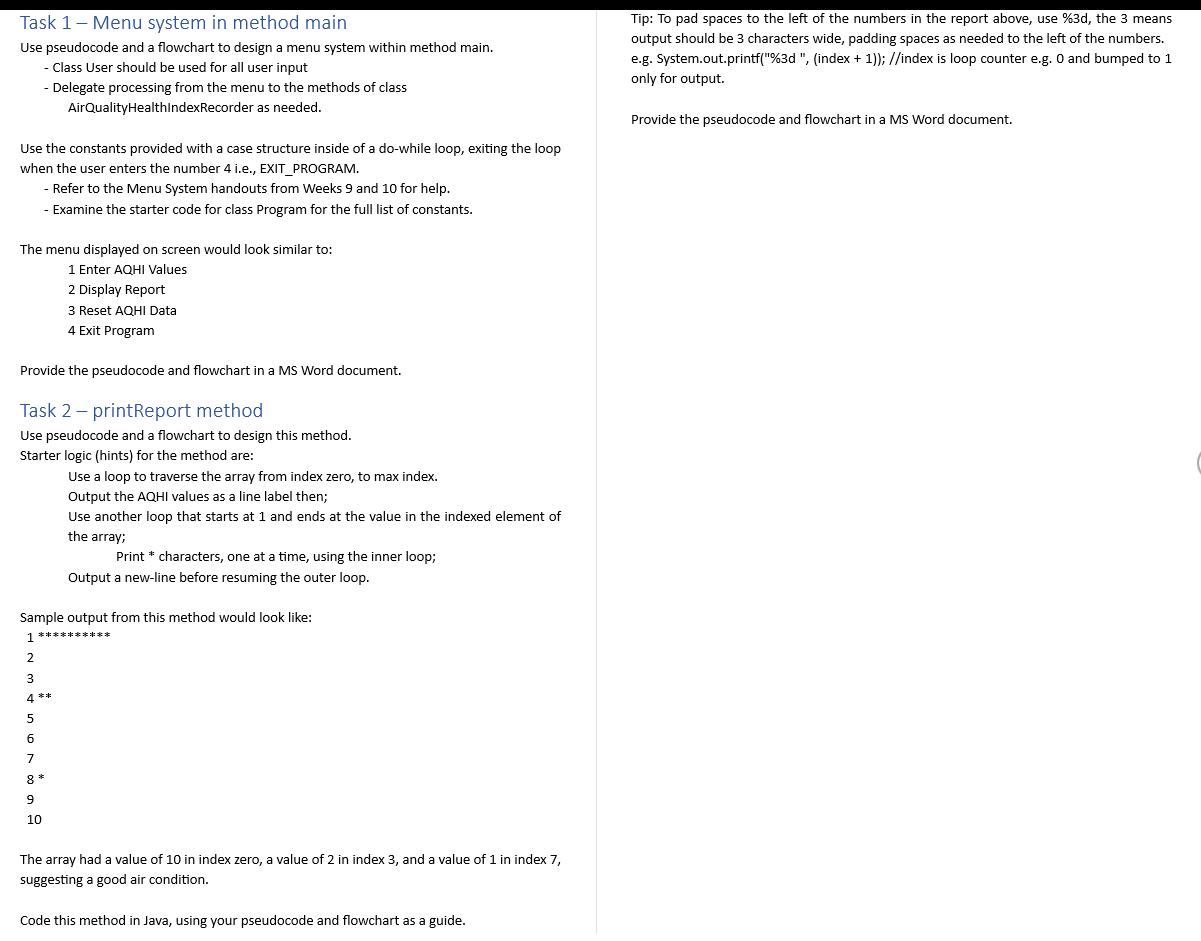
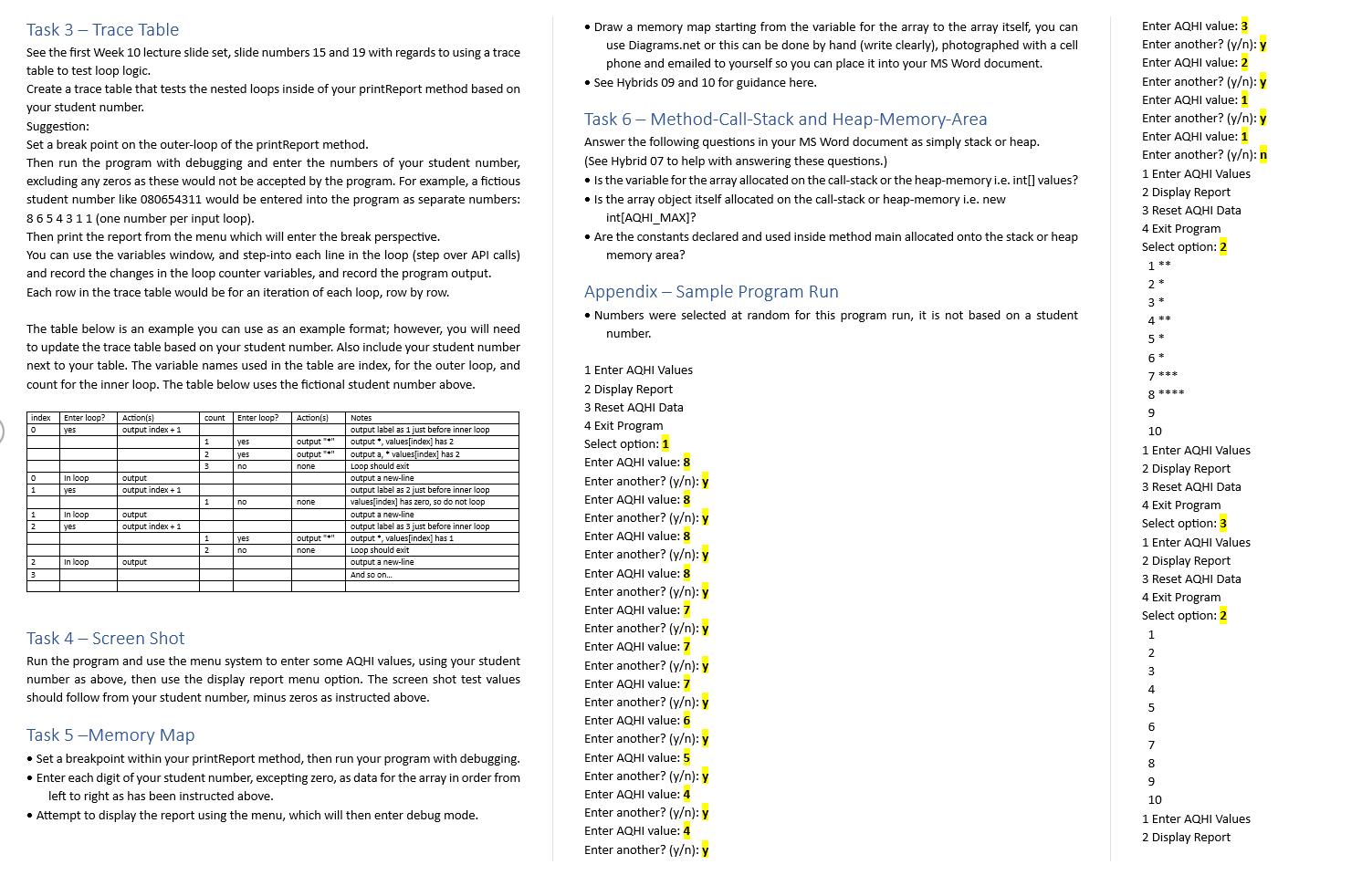
In this project you will work on a program that will help visualize a bar graph chart of Air Quality Health Index [1] data, collected, and entered by user. There are three classes provided for starter code: Program with method main User with methods for user inputs AirQualityHealthIndexRecord with methods, enterData, printReport, resetData The program you create must use a 10-element array of int, loops, a basic menu system and be object oriented. Most of the program functionality is completed for you, you will need to provide programmer comments as indicated and expected, as well as author method main, and method printReport. A detailed UML class diagram is provided to overview the classes: -keyboard:Scanner -User() +inputinteger() int +inputinteger(prompt:String): int +inputDouble():double +inputDouble(prompt:String):double +inputString():String +inputString(message:String): String +inputinteger(prompt:String, min:int, max:int):int User AirQualityhealthIndexRecorder -AQHI_MAX:int -values:int[] +AirQualityhealthIndexRecorder() +enterData():void +printReport():void +resetData():void Program +main(args:String[]):void UML Class Diagram by Amir Rad Task 1 - Menu system in method main Use pseudocode and a flowchart to design a menu system within method main. - Class User should be used for all user input - Delegate processing from the menu to the methods of class AirQualityHealthIndexRecorder as needed. Use the constants provided with a case structure inside of a do-while loop, exiting the loop when the user enters the number 4 i.e., EXIT_PROGRAM. - Refer to the Menu System handouts from Weeks 9 and 10 for help. - Examine the starter code for class Program for the full list of constants. The menu displayed on screen would look similar to: 1 Enter AQHI Values 2 Display Report 3 Reset AQHI Data 4 Exit Program Provide the pseudocode and flowchart in a MS Word document. Task 2 printReport method Use pseudocode and a flowchart to design this method. Starter logic (hints) for the method are: Use a loop to traverse the array from index zero, to max index. Output the AQHI values as a line label then; Use another loop that starts at 1 and ends at the value in the indexed element of the array; 5 6 7 8* 9 10 Print* characters, one at a time, using the inner loop; Output a new-line before resuming the outer loop. Sample output from this method would look like: 1 ********** 2 3 4** The array had a value of 10 in index zero, a value of 2 in index 3, and a value of 1 in index 7, suggesting a good air condition. Code this method in Java, using your pseudocode and flowchart as a guide. Tip: To pad spaces to the left of the numbers in the report above, use %3d, the 3 means output should be 3 characters wide, padding spaces as needed to the left of the numbers. e.g. System.out.printf("%3d", (index + 1)); //index is loop counter e.g. 0 and bumped to 1 only for output. Provide the pseudocode and flowchart in a MS Word document. Task 3 - Trace Table See the first Week 10 lecture slide set, slide numbers 15 and 19 with regards to using a trace table to test loop logic. Create a trace table that tests the nested loops inside of your printReport method based on your student number. Suggestion: Set a break point on the outer-loop of the printReport method. Then run the program with debugging and enter the numbers of your student number, excluding any zeros as these would not be accepted by the program. For example, a fictious student number like 080654311 would be entered into the program as separate numbers: 8654311 (one number per input loop). Then print the report from the menu which will enter the break perspective. You can use the variables window, and step-into each line in the loop (step over API calls) and record the changes in the loop counter variables, and record the program output. Each row in the trace table would be for an iteration of each loop, row by row. The table below is an example you can use as an example format; however, you will need to update the trace table based on your student number. Also include your student number next to your table. The variable names used in the table are index, for the outer loop, and count for the inner loop. The table below uses the fictional student number above. index 0 0 1 2 2 Enter loop? yes In loop ves In loop yes In loop Action(s) output index +1 output output index + output output index +1 output count Enter loop? 2 3 1 1 2 yes yes no no yes Action(s) output output none none output **" none Notes output label as 1 just before inner loop output *, values[index] has 2 output a, * values[index] has 2 Loop should exit output a new-line output label as 2 just before inner loop values[index] has zero, so do not loop output a new-line output label as 3 just before inner loop output, values[index] has 1 Loop should exit output a new-line And so on.. Task 4-Screen Shot Run the program and use the menu system to enter some AQHI values, using your student number as above, then use the display report menu option. The screen shot test values should follow from your student number, minus zeros as instructed above. Task 5-Memory Map Set a breakpoint within your printReport method, then run your program with debugging. Enter each digit of your student number, excepting zero, as data for the array in order from left to right as has been instructed above. Attempt to display the report using the menu, which will then enter debug mode. Draw a memory map starting from the variable for the array to the array itself, you can use Diagrams.net or this can be done by hand (write clearly), photographed with a cell phone and emailed to yourself so you can place it into your MS Word document. See Hybrids 09 and 10 for guidance here. Task 6-Method-Call-Stack and Heap-Memory-Area Answer the following questions in your MS Word document as simply stack or heap. (See Hybrid 07 to help with answering these questions.) Is the variable for the array allocated on the call-stack or the heap-memory i.e. int[] values? Is the array object itself allocated on the call-stack or heap-memory i.e. new int[AQHI_MAX]? Are the constants declared and used inside method main allocated onto the stack or heap memory area? Appendix - Sample Program Run Numbers were selected at random for this program run, it is not based on a student number. 1 Enter AQHI Values 2 Display Report 3 Reset AQHI Data 4 Exit Program Select option: 1 Enter AQHI value: 8 Enter another? (y/n): y Enter AQHI value: 8 Enter another? (y/n): y Enter AQHI value: 8 Enter another? (y/n): y Enter AQHI value: 8 Enter another? (y/n): y Enter AQHI value: 7 Enter another? (y/n): y Enter AQHI value: 7 Enter another? (y/n): y Enter AQHI value: 7 Enter another? (y/n): y Enter AQHI value: 6 Enter another? (y/n): y Enter AQHI value: 5 Enter another? (y/n): y Enter AQHI value: 4 Enter another? (y/n): y Enter AQHI value: 4 Enter another? (y/n): y Enter AQHI value: 3 Enter another? (y/n): y Enter AQHI value: 2 Enter another? (y/n): y Enter AQHI value: 1 Enter another? (y/n): y Enter AQHI value: 1 Enter another? (y/n): n 1 Enter AQHI Values 2 Display Report 3 Reset AQHI Data 4 Exit Program Select option: 2 1 ** 2* 3* 4 ** 5* 6* 7*** 8 **** 9 10 1 Enter AQHI Values 2 Display Report 3 Reset AQHI Data 4 Exit Program Select option: 3 1 Enter AQHI Values 2 Display Report 3 Reset AQHI Data 4 Exit Program Select option: 2 1 2 3 4 5 6 7 8 9 10 1 Enter AQHI Values 2 Display Report
Step by Step Solution
★★★★★
3.30 Rating (150 Votes )
There are 3 Steps involved in it
Step: 1
The project aims to develop a Java program for visualizing a bar graph of Air Quality Health Index AQHI data entered by the user The program utilizes ...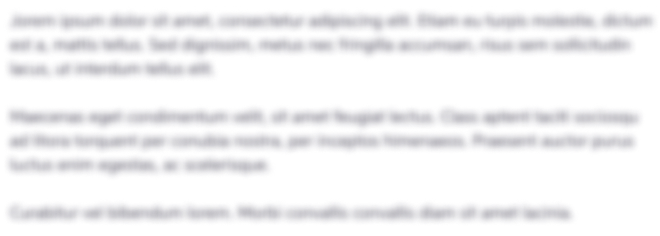
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started