Answered step by step
Verified Expert Solution
Question
1 Approved Answer
#include #include battleShip.h using namespace std; int main(){ //TODO: Declare 3 instances of the battleship class: Destroyer Carrier Cruiser //TOD0: Give the ships a size:
#include#include "battleShip.h" using namespace std; int main(){ //TODO: Declare 3 instances of the battleship class: Destroyer Carrier Cruiser //TOD0: Give the ships a size: Destroyer-3 Carrier-5 Cruiser-2 // you will need to call the appropriate methods // Once you have this running for one, expand this while loop to include the other // two instances. Have the while loop end when all three ships have been sunk while(ship_one.isSunk() == false){ ship_one.recordHit(); } }
#ifndef BATTLESHIP_H #define BATTLESHIP_H #includeusing namespace std; class battleShip{ public: battleShip(string); ~battleShip(); void setShipName(string); string getShipName(); void setSize(int); int getSize(); void recordHit(); bool isSunk(); private: string name; int size; int hits; }; #endif // BATTLESHIP_H
#include// TODO: Include the header files for the planet class (part 2) and the solar system class (part 3) using namespace std; int main(){ /// Part 2: Planets //TODO: Declare 4 instances of the planet class, using the following table for reference /* NAME RADIUS (km) ORBIT RADIUS (AU) Mercury 2440 0.387 Venus 6052 0.723 Earth 6371 1.000 Mars 3390 1.524 */ // Use the following print statements to test your planet class code for(int i = 0; i
#include "planet.h" #includeplanet::planet(){ } planet::planet(string planetName, float planetRadius, float planetDist){ name = planetName; radius = planetRadius; distance = planetDist; } planet::~planet(){ //letting compiler handle deconstruction of the class } void planet::setName(string planetName){ name = planetName; } string planet::getName(){ return name; } void planet::setRadius(float planetRadius){ radius = planetRadius; } float planet::getDiameter(){ return 2*radius; } void planet::setDist(float planetDist){ distance = planetDist; } float planet::getDist(){ return distance; } float planet::getOrbitPeriod(){ // Kepler's 3rd Law: // The square of the orbital period of a planet is proportional to the cube of its orbital distance. // P*P = A*A*A: [P] = years, [A] = Astronomical Units, // With these units, the constant of proportionality is 1. (only true for our Solar System) // see also: http://spiff.rit.edu/classes/phys440/lectures/kepler3/kepler3.html return sqrt(distance*distance*distance); }
#ifndef SOLAR_SYSTEM_H #define SOLAR_SYSTEM_H #include#include using namespace std; class solarSystem{ public: solarSystem(string); ~solarSystem(); string findLargest(); // return the name of the largest planet string getName(); // return the name of the solar system float getDiameter(); // return the diameter of the largest orbit int getNumPlanets(); // return the number of planets in the system bool addPlanet(string, float, float); private: int maxNumPlanets = 10; string systemName; int numPlanets; string planetNames[10]; float planetDiameters[10]; float planetOrbitDist[10]; }; #endif // SOLAR_SYSTEM_H
this is all the information I was given to complete this activity and I've been trying for several hours to decipher it with no results
Recitation Activity For today's recitation activity you are going to implement three separate classes. Part 1: Battle Ship The first class you will implement is called battleship. The battleShip h file has been made available on Moodle. You are also given the the beginning of a main file for Battle Ship: Battleship main.cpp. Download these files and place them into the same folder on your computer. Open them with CodeBlocks. You will implement the battle Ship class and fill out the main file for part 1 of the recitation You will create your class file battleship.cpp. In battleShip.cpp you will define the methods. (Remember: methods define what your object does). How many methods do you see in your header file? It will be your job to fill in these methods so that they work.The constructor always has the same name as your class file, and it never has a data type. Make sure to import the proper class file "battleship.h" and librariesetc. in the header of your battleship.cpp file. See the BattleShip main.cpp for further instructions. You will need to create 3 instances of your class, set up some of the methods, and expand on the logic of the while loop When you have finished, implementing these methods, show your TA to check off your work. Then move onto the next part Part 2: Planet Class Parts 2 and 3 of this recitation activity have a shared main file Solarsystem main.cpp, provided to you on Moodle The next class you will implement is called planet. This time we have given you the class file planet.cpp. It is your job to create the header file for the planet class. Create the file planet.h in CodeBlocks in the same directory as the main file. The planet class has two constructors, a default constructor (which takes no arguments) and a constructor which takes the arguments: string planetName, float planetRadius (the radius of the planet, in km), float planetDist (the distance the planet orbits from the sun, in Astronomical Units) Once you have implemented the header file for the planet class, you will need to modify main to test your class. Use the table of planet data provided to you in the main file to create four planet objects of the Solar System. It will be helpful to use an array for this task, as the code to test your planets assumes an array of planet objects. Recitation Activity For today's recitation activity you are going to implement three separate classes. Part 1: Battle Ship The first class you will implement is called battleship. The battleShip h file has been made available on Moodle. You are also given the the beginning of a main file for Battle Ship: Battleship main.cpp. Download these files and place them into the same folder on your computer. Open them with CodeBlocks. You will implement the battle Ship class and fill out the main file for part 1 of the recitation You will create your class file battleship.cpp. In battleShip.cpp you will define the methods. (Remember: methods define what your object does). How many methods do you see in your header file? It will be your job to fill in these methods so that they work.The constructor always has the same name as your class file, and it never has a data type. Make sure to import the proper class file "battleship.h" and libraries etc. in the header of your battleship.cpp file. See the BattleShip main.cpp for further instructions. You will need to create 3 instances of your class, set up some of the methods, and expand on the logic of the while loop When you have finished, implementing these methods, show your TA to check off your work. Then move onto the next part Part 2: Planet Class Parts 2 and 3 of this recitation activity have a shared main file Solarsystem main.cpp, provided to you on Moodle The next class you will implement is called planet. This time we have given you the class file planet.cpp. It is your job to create the header file for the planet class. Create the file planet.h in CodeBlocks in the same directory as the main file. The planet class has two constructors, a default constructor (which takes no arguments) and a constructor which takes the arguments: string planetName, float planetRadius (the radius of the planet, in km), float planetDist (the distance the planet orbits from the sun, in Astronomical Units) Once you have implemented the header file for the planet class, you will need to modify main to test your class. Use the table of planet data provided to you in the main file to create four planet objects of the Solar System. It will be helpful to use an array for this task, as the code to test your planets assumes an array of planet objects
Step by Step Solution
There are 3 Steps involved in it
Step: 1
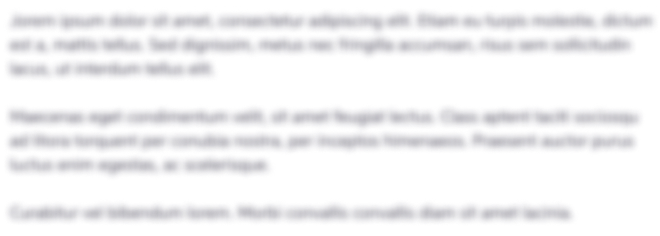
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started