Question
#include #include #include #define MIN_BALANCE 1000 using namespace std; class Account { string firstName, lastName; int accountNumber; int balance; int pin; public: void setFname(string fname);
#include
class Account { string firstName, lastName; int accountNumber; int balance; int pin; public: void setFname(string fname); void setLname(string lname); void setAccNo(int accNo); void setBalance(int sbalance); void setpin(int pin); Account(string fname = " ", string lname = " ", int accNo = 0) { setFname(fname); setLname(lname); setAccNo(accNo); setpin(pin); } string getFname(); string getLname(); int getAccNo(); int getBalance(); void getWithdrawal(int wtd); int getDeposit(int dpo); //~Account(); };
void Account::setFname(string fname) { firstName = fname; }
void Account::setLname(string lname) { lastName = lname; }
void Account::setAccNo(int accNo) { accountNumber = accNo; } void Account::setpin(int pin) { pin=123456; } void Account::setBalance(int sbalance) { try { if(sbalance < MIN_BALANCE) { throw 100; } balance = sbalance; } catch(int e) { cout << " Error: " << e; cout << " Minimum Balance: " << MIN_BALANCE; } }
string Account::getFname() { return firstName; }
string Account::getLname() { return lastName; }
int Account::getAccNo() { return accountNumber; }
int Account::getBalance() { return balance; }
void Account::getWithdrawal(int wtd) { fstream fi; fi.open("account.txt", ios::in | ios::app); try { if(balance - wtd < MIN_BALANCE) { throw 101; } balance -= wtd; fi << " Withdrawal: " << wtd; } catch(int e) { cout << " Error: 101 Withdrawal cannot exceed " << MIN_BALANCE; } fi.close(); }
int Account::getDeposit(int dpo) { fstream fi; fi.open("account.txt", ios::in | ios::app); balance += dpo; fi << " Deposit: " << dpo; fi.close(); return balance; }
int main() { fstream fi; fi.open("account.txt", ios::in | ios:: out | ios::trunc); Account a; string fname, lname; int accNo, balance, choice, deposit, withdraw; int pin = 123456; cout << "\t********************************************************** " << endl << " \t\t THE BMMH FTKMP BANK \t\t\t ------* WELCOME *------ " << endl << "\t********************************************************** " << "\t\t\t\tUSER LOGIN" << endl; cout << " \t\tEnter First Name: "; cin >> fname; a.setFname(fname); fi << "First Name: " << fname << endl; cout << " \t\tEnter Last Name: "; cin >> lname; a.setLname(lname); fi << "Last Name: " << lname << endl; cout << " \t\t\tEnter PIN: "; cin >> pin; cout << " "; a.setAccNo(accNo); fi << "A/c No: " << accNo << endl; while (pin != 123456) { cout << "\t********************************************************** " << endl << " \t\t THE BMMH FTKMP BANK \t\t\t ------* WELCOME *------ " << endl << "\t********************************************************** " << "\t\t\t\tUSER LOGIN " << endl << "\t\t INVALID PIN WAS ENTERED " << endl; cout <<" Enter PIN: "; cin >> pin; cout << " "; } cout << " Enter Balance: "; cin >> balance; a.setBalance(balance);
while(choice != 3) { cout << " 1)Deposit"; cout << " 2)Withdraw"; cout << " 3)Exit"; cout << " Select any given option to proceed: "; cin >> choice; switch(choice) { case 1: cout << " Enter Deposit Amount: "; cin >> deposit; a.getDeposit(deposit); cout << " Your Current Balance: " << a.getBalance(); break;
case 2: cout << " Enter Withdrawal Amount: "; cin >> withdraw; a.getWithdrawal(withdraw); cout << " Your Current Balance: " << a.getBalance(); break; case 3: break;
default: cout << " Enter Valid Option"; break; } } fi.close(); return 0; }
Make a flowchart based on the program code above
Step by Step Solution
There are 3 Steps involved in it
Step: 1
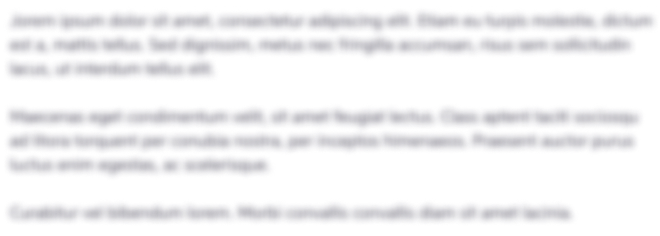
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started