Answered step by step
Verified Expert Solution
Question
1 Approved Answer
#include #include #include #include #include #include using namespace std; // implementing the dynamic List ADT using array // operations to be implemented: read, Modify, delete,
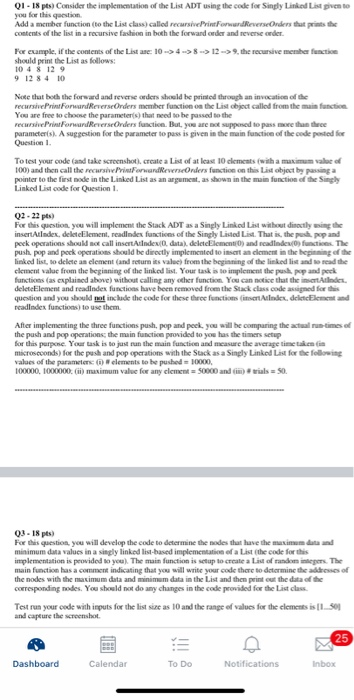
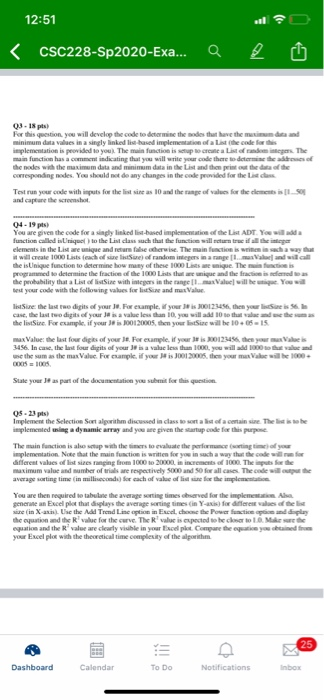
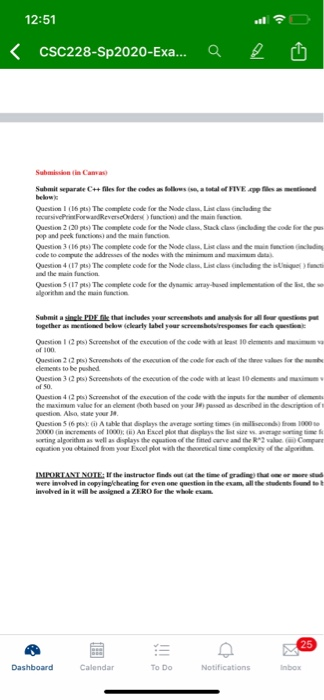
#include
#include
#include
#include
#include
#include
using namespace std;
// implementing the dynamic List ADT using array
// operations to be implemented: read, Modify, delete, isEmpty, insert, countElements
class List{
private:
int *array;
int maxSize; // useful to decide if resizing (doubling the array size) is needed
int endOfArray;
public:
List(int size){
array = new int[size];
maxSize = size;
endOfArray = -1;
}
void deleteList(){
delete[] array;
}
bool isEmpty(){
if (endOfArray == -1)
return true;
return false;
}
void resize(int s){
int *tempArray = array;
array = new int[s];
for (int index = 0; index
array[index] = tempArray[index];
}
maxSize = s;
}
void insert(int data){
if (endOfArray == maxSize-1)
resize(2*maxSize);
array[++endOfArray] = data;
}
void insertAtIndex(int insertIndex, int data){
// if the user enters an invalid insertIndex, the element is
// appended to the array, after the current last element
if (insertIndex > endOfArray+1)
insertIndex = endOfArray+1;
if (endOfArray == maxSize-1)
resize(2*maxSize);
for (int index = endOfArray; index >= insertIndex; index--)
array[index+1] = array[index];
array[insertIndex] = data;
endOfArray++;
}
int read(int index){
return array[index];
}
void modifyElement(int index, int data){
array[index] = data;
}
void deleteElement(int deleteIndex){
// shift elements one cell to the left starting from deleteIndex+1 to endOfArray-1
// i.e., move element at deleteIndex + 1 to deleteIndex and so on
for (int index = deleteIndex; index
array[index] = array[index+1];
endOfArray--;
}
int countList(){
int count = 0;
for (int index = 0; index
count++;
return count;
}
void print(){
for (int index = 0; index
cout
cout
}
};
void SelectionSort(List list){
// Implement the algorithm here for a dynamic array-based implementation of List
}
}
int main(){
using namespace std::chrono;
srand(time(NULL));
int maxValue;
cout
cin >> maxValue;
int numTrials;
cout
cin >> numTrials;
for (int listSize = 1000; listSize
double totalSortingTime = 0;
for (int trials = 1; trials
List integerList(1);
for (int i = 0; i
Q1.18 ) Consider the implementation of the List ADT using the code for SinglyLinked Liven to you for this question Add a member function to the List Class) called recursive PriForwardeweerde that the contents of the list in a recursive fashion in both the forward onder andere onder : 10-4 8 12- themsiemene t Foreumple, if the contents of the List should print the List as follows: 10 4 8 129 91284 10 Note that both the forward and anders should be printed through an in t e Fon d er member function on the list called from the functie You are free to choose the parameters that need to be puedo the Fonductie Buty do p ode A t for the parameter to pass is given is the functie de p e Question To test your code and take w hat create a Litwa 10 demesham 100) and the call the to u ch Liebe per to the first and the Link List a w ea t he in functie Linkediode for Quel 02-22 ) For this question you will get the Stack ADT a Singly Liked wh ich the insertides del mercadde functies of the Singhy Lid That is the und perkoperties or call de data del comen d o a The pushpad pork pereshed be directly implemented to set in the he a the linked list to delete a comment and return its value from the beginning the link e d the cleme value from the beginning of the linked it. Your task implement the p a ck functions as explained above without calling my her functie You can notice that the dellement and readinder functions have been removed from the Stackassende e d for his question and you like the code for these three functi e deemed readinder functions to use them After implementing the three functions push, pop and pack, you will be comparing the actual times the push and pop operations, the main functie provided to you has the times setup for this purpose. Your task is to just run the main function and measure the average time to ti microsconds) for the push and pop operations with the Stack as a Singly Linked for the following values of the paramen e lements to be pushed = 10000 100000 1000000, mimum value for any cle S 000 and was so 01.IS For this will develop the code d he duhethe d d in ang based impleme n te code for this implementatie is wided to you. The main functio n in functies indicating that you will write your code there to determine the nodes with the data and data in the L and then that responding modes. You should do my changes in the code provided for the List The how the Test your code with inputs for the issue as 10 and the range of values for the elements isso und capture the rest Dashboard Calendar To Do Notifications Inbox 12:51 int value = 1 + rand() % maxValue;
integerList.insertAtIndex(i, value);
}
high_resolution_clock::time_point t1 = high_resolution_clock::now();
SelectionSort(integerList);
high_resolution_clock::time_point t2 = high_resolution_clock::now();
duration sortingTime_milli = t2 - t1;
totalSortingTime += sortingTime_milli.count();
integerList.deleteList();
}
cout
} // list size loop
system("pause");
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
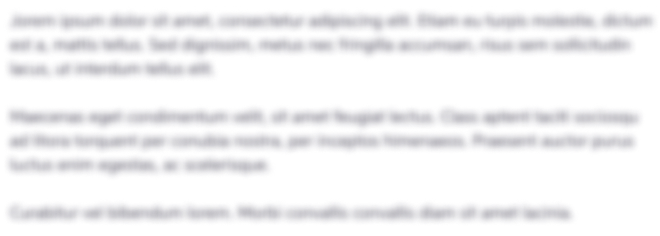
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started