Question
#include #include #include #pragma warning(disable: 4996) // for Visual Studio #define MAX_NAME 30 // global linked list 'list' contains the list of books struct libraryList
#include
#pragma warning(disable: 4996) // for Visual Studio
#define MAX_NAME 30
// global linked list 'list' contains the list of books struct libraryList { struct book* book; struct libraryList* next; } *list = NULL; // currently empty list
// structure "book" contains the book's title, aisle number and linked list of bookType struct book { char title[MAX_NAME]; unsigned int aisle; struct bookType* types; // linked list 'types' contains names of bookType };
// structure 'bookType' contains type name struct bookType { char type[MAX_NAME]; struct bookType* next; };
// forward declaration of functions (already implmented) void flushStdIn(); void executeAction(char);
// functions already implemented void addBook(char* bookTitleInput, unsigned int aisleInput); void displayBookList(struct libraryList* tempList);
// functions that need to be implemented struct book* searchBook(char* bookTitleInput); // 5 points
void addBookType(char* bookTitleInput, char* typeInput); // 15 points void displayBookTypeList(struct libraryList* tempList); // 15 points void removeBook(char* bookTitleInput); // 15 points
int main() { char selection = 'a'; // initialized to a dummy value do { printf(" CSE240 HW 7 "); printf("Please enter your selection: ");
printf("\t a: add a new book to the list "); printf("\t d: display book list (no book type) "); printf("\t b: search for a book on the list "); printf("\t c: add a book type of a book "); printf("\t l: display books who have a specific book type "); printf("\t r: remove a book "); printf("\t q: quit ");
selection = getchar(); flushStdIn(); executeAction(selection); } while (selection != 'q');
return 0; }
// flush out leftover ' ' characters void flushStdIn() { char c; do c = getchar(); while (c != ' ' && c != EOF); }
// Ask for details from user for the given selection and perform that action // Read the function case by case void executeAction(char c) { char bookTitleInput[MAX_NAME], bookTypeInput[MAX_NAME]; unsigned int aisleInput; struct book* searchResult = NULL;
switch (c) { case 'a': // add book // input book details from user printf(" Please enter books's title: "); fgets(bookTitleInput, sizeof(bookTitleInput), stdin); bookTitleInput[strlen(bookTitleInput) - 1] = '\0'; // discard the trailing ' ' char printf("Please enter aisle number: "); scanf("%d", &aisleInput); flushStdIn();
//if (searchBook(bookTitleInput) == NULL) // un-comment this line after implementing searchBook() if (1) // comment out this line after implementing searchBook() { addBook(bookTitleInput, aisleInput); printf(" Book successfully added to the list! "); } else printf(" That Book is already on the list! "); break;
case 'd': // display the list displayBookList(list); break;
case 'b': // search for an book on the list printf(" Please enter book's title: "); fgets(bookTitleInput, sizeof(bookTitleInput), stdin); bookTitleInput[strlen(bookTitleInput) - 1] = '\0'; // discard the trailing ' ' char
//if (searchBook(bookTitleInput) == NULL) // un-comment this line after implementing searchBook() if (0) // comment out this line after implementing searchBook() printf(" Book title does not exist or the list is empty! "); else { printf(" Book title exists on the list! "); } break;
case 'r': // remove book printf(" Please enter book's title: "); fgets(bookTitleInput, sizeof(bookTitleInput), stdin); bookTitleInput[strlen(bookTitleInput) - 1] = '\0'; // discard the trailing ' ' char
//if (searchBook(bookTitleInput) == NULL) // un-comment this line after implementing searchBook() if (0) // comment out this line after implementing searchBook() printf(" Book title does not exist or the list is empty! "); else { removeBook(bookTitleInput); printf(" Book title successfully removed from the list! "); } break;
case 'c': // add book type printf(" Please enter book's title: "); fgets(bookTitleInput, sizeof(bookTitleInput), stdin); bookTitleInput[strlen(bookTitleInput) - 1] = '\0'; // discard the trailing ' ' char
//if (searchBook(bookTitleInput) == NULL) // un-comment this line after implementing searchBook() if (0) // comment out this line after implementing searchBook() printf(" Book title does not exist or the list is empty! "); else { printf(" Please enter book type: "); fgets(bookTypeInput, sizeof(bookTypeInput), stdin); bookTypeInput[strlen(bookTypeInput) - 1] = '\0'; // discard the trailing ' ' char
addBookType(bookTitleInput, bookTypeInput); printf(" Book type added! "); } break;
case 'l': // list book type's books displayBookTypeList(list); break;
case 'q': // quit break;
default: printf("%c is invalid input! ", c); } }
// Q3: searchBook (5 points) // This function searches the 'list' to check if the given book exists in the list. Search by book title. // If it exists then return that 'book' node of the list. Notice the return type of this function. // If the book does not exist in the list, then return NULL. // NOTE: After implementing this fucntion, go to executeAction() to comment and un-comment the lines mentioned there which use searchBook() // in case 'a', case 'r', case 'l' (total 3 places) struct book* searchBook(char* bookTitleInput) {
struct libraryList* tempList = list; // work on a copy of 'list' // Enter code here
return NULL; }
// Q4: addBookType (15 points) // This function adds book type name to a book node. // Parse the list to locate the book and add the book type to that book's 'bookType' linked list. No need to check if the book title exists on the list. That is done in executeAction(). // If the 'bookType' list is empty, then add the bookType. If the book has existing book types, then you may add the new book type to the head or the tail of the 'bookType' list. // You can assume that the same book type does not exist. So no need to check for existing book type, like we do when we add new book. // NOTE: Make note of whether you add the bookType to the head or tail of 'bookType' list. // (Sample solution has book type added to the tail of 'bookType' list. You are free to add new book type to head or tail of 'bookType' list.)
void addBookType(char* bookTitleInput, char* bookTypeInput) {
struct libraryList* tempList = list; // work on a copy of 'list'
// Enter code here }
// Q5: displayBookTypeList (15 points) // This function prompts the user to enter a type name. This function then searches for books with this book type. // Parse through the linked list passed as parameter and print the matching book details ( title and aisle) one after the other. See expected output screenshots in homework question file. // HINT: Use inputs gathered in executeAction() as a model for getting the book type input. // NOTE: You may re-use some Q2 displayBookList(list) code here. void displayBookTypeList(struct libraryList* tempList) { // Enter code here }
// Q6: removeBook (15 points) // This function removes a book from the list. // Parse the list to locate the book and delete that 'book' node. // You need not check if the book exists because that is done in executeAction() // removeBook() is supposed to remove book details like title and aisle number. // The function will remove bookTypes of the book too. // When the book is located in the 'list', after removing the title and aisle number, parse the 'bookType' list of that book // and remove the book types.
void removeBook(char* bookTitleInput) {
struct libraryList* tempList = list; // work on a copy of 'list' struct bookType* tempBookType; // Enter code here
}
need help with q3,4,5,6.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
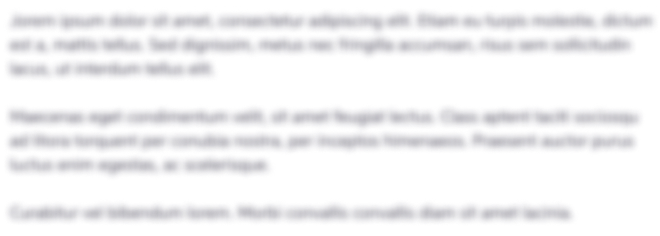
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started