Question
#include #include #include using namespace std; // implementing the dynamic List ADT using Linked List class Node{ private : int data; Node* nextNodePtr; public :
#include
#include
#include
using namespace std;
// implementing the dynamic List ADT using Linked List
class Node{
private:
int data;
Node* nextNodePtr;
public:
Node(){}
void setData(int d){
data = d;
}
int getData(){
return data;
}
void setNextNodePtr(Node* nodePtr){
nextNodePtr = nodePtr;
}
Node* getNextNodePtr(){
return nextNodePtr;
}
};
class List{
private:
Node *headPtr;
public:
List(){
headPtr = new Node();
headPtr->setNextNodePtr(0);
}
Node* getHeadPtr(){
return headPtr;
}
bool isEmpty(){
if (headPtr->getNextNodePtr() == 0)
return true;
return false;
}
void insert(int data){
Node* currentNodePtr = headPtr->getNextNodePtr();
Node* prevNodePtr = headPtr;
while (currentNodePtr != 0){
prevNodePtr = currentNodePtr;
currentNodePtr = currentNodePtr->getNextNodePtr();
}
Node* newNodePtr = new Node();
newNodePtr->setData(data);
newNodePtr->setNextNodePtr(0);
prevNodePtr->setNextNodePtr(newNodePtr);
}
void insertAtIndex(int insertIndex, int data){
Node* currentNodePtr = headPtr->getNextNodePtr();
Node* prevNodePtr = headPtr;
int index = 0;
while (currentNodePtr != 0){
if (index == insertIndex)
break;
prevNodePtr = currentNodePtr;
currentNodePtr = currentNodePtr->getNextNodePtr();
index++;
}
Node* newNodePtr = new Node();
newNodePtr->setData(data);
newNodePtr->setNextNodePtr(currentNodePtr);
prevNodePtr->setNextNodePtr(newNodePtr);
}
void deleteALLElements(int data){
// Insert code here
}
void deleteElement(int deleteIndex){
Node* currentNodePtr = headPtr->getNextNodePtr();
Node* prevNodePtr = headPtr;
Node* nextNodePtr = headPtr;
int index = 0;
while (currentNodePtr != 0){
if (index == deleteIndex){
nextNodePtr = currentNodePtr->getNextNodePtr();
break;
}
prevNodePtr = currentNodePtr;
currentNodePtr = currentNodePtr->getNextNodePtr();
index++;
}
prevNodePtr->setNextNodePtr(nextNodePtr);
}
void IterativePrint(){
Node* currentNodePtr = headPtr->getNextNodePtr();
while (currentNodePtr != 0){
cout getData()
currentNodePtr = currentNodePtr->getNextNodePtr();
}
cout
}
};
int main(){
srand(time(NULL));
int listSize;
cout
cin >> listSize;
int maxValue;
cout
cin >> maxValue;
List integerList; // Create an empty list
for (int i = 0; i
int value = 1 + rand()%maxValue;
integerList.insertAtIndex(i, value);
}
cout
integerList.IterativePrint();
int deleteData;
cout
cin >> deleteData;
integerList.deleteALLElements(deleteData);
cout
integerList.IterativePrint();
system("pause");
return 0;
}
In this programming assignment, you will design and implement algorithms to delete all the occurrences of an element (integer data) in a List ADT: implemented using a singly linked list. You are given the code for the singly linked list-based implementations of a List ADT. The main function in this program is already setup to generate random integers and fill up the contents of the list. The deleteALL(int deleteData) function is called on the list to delete all the occurrences of the 'deleteData' in the list. Your task is to implement the delete ALL(...) function in the singly linked list-based implementation of the List ADT. Your algorithm/implementation should run in O(n) time, where n is the number of elements in the list before the deletions. The main function is written in such a way that it will print the contents of the list before and after the deletions. Testing Procedure: You would test your code by entering 15 as the list size and 5 as the maximum value for any element. The list will be then filled with random integers in the range [1...5]. As there are going to be 15 integers in the list, it will be definitely the case that there will be more than one occurrence for one or more integers in the list. Enter one of such integers as the input for the 'deleteData' and the delete ALL(...) function is called with the deleteData to delete all the occurrences of deleteData in the list. Submission: 1) The entire .cpp file for the singly linked list-based implementation of the List ADT. 2) Screenshot of the execution of the implementation (using the testing procedure described above) submitted as a jpeg or in some other image file formatStep by Step Solution
There are 3 Steps involved in it
Step: 1
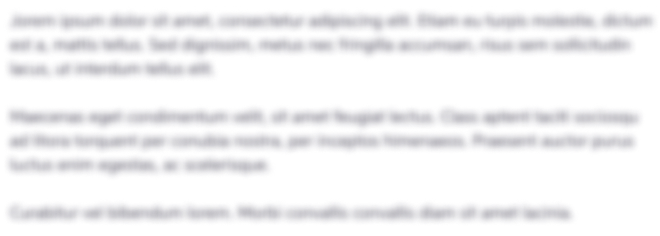
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started