Question
include #include #include using std::cout; using std::cin; using std::endl; using std::string; ////////////////////////////////////////////////////////////////////////// / // Global constants ////////////////////////////////////////////////////////////////////////// / const int MAX_ROWS = 11; const int
include
#include
#include
using std::cout;
using std::cin;
using std::endl;
using std::string;
//////////////////////////////////////////////////////////////////////////
/
// Global constants
//////////////////////////////////////////////////////////////////////////
/
const int MAX_ROWS = 11;
const int MAX_COLS = 11;
const int BLINKER_HEIGHT = 3;
const int BLINKER_WIDTH = 1;
const int GLIDER_SIZE = 3;
const int MAX_STEPS = 50;
const char ALIVE = 'X';
const char DEAD = '.';
//////////////////////////////////////////////////////////////////////////
/
// Utility function declarations
//////////////////////////////////////////////////////////////////////////
/
void clearScreen();
void delay(int ms);
void report();
//////////////////////////////////////////////////////////////////////////
/
// Type definitions
//////////////////////////////////////////////////////////////////////////
/
class World;
class Life {
public:
// Accessors or Getters
int getCol() const;
int getRow() const;
int getHeight() const;
int getWidth() const;
char getFromFigure(int r, int c) const;
protected:
int m_col;
int m_row;
int m_height;
int m_width;
char **m_figure;
World *m_world;
};
class Blinker : public Life {
public:
// Constructor/destructor
Blinker(int r, int c);
~Blinker();
};
class Glider : public Life {
public:
// Constructor/destructor
Glider(int r, int c);
~Glider();
};
class World {
public:
// Constructor/destructor
World();
~World();
// Accessors or Getters
void print() const;
bool hasWorldChanged() const;
// Mutators or Setters
bool addLife(Life *life);
void update();
private:
char _getState(char state, char row, char col, bool toggle);
// The rules of Conway's Game of Life requires each cell to
// examine it's neighbors. So, we have to check the entire world
// first before we change it. We can use two copies of the world,
// one to check the current state then another to generate the
// the next state. We can toggle between them each step, to avoid
// having to copy between worlds each step of the game.
char **m_world;
char **m_otherWorld;
bool m_toggle;
};
class Game {
public:
// Constructor/destructor
Game(Life **life, int numLife);
~Game();
// Mutators
void play();
private:
World * m_world;
int m_steps;
bool m_automate;
};
//////////////////////////////////////////////////////////////////////////
/
// Life Implemention
//////////////////////////////////////////////////////////////////////////
/
int Life::getCol() const {
return m_col;
}
int Life::getRow() const {
return m_row;
}
int Life::getHeight() const {
return m_height;
}
int Life::getWidth() const {
return m_width;
}
char Life::getFromFigure(int r, int c) const {
return m_figure[r][c];
}
//////////////////////////////////////////////////////////////////////////
/
// Blinker Implemention
//////////////////////////////////////////////////////////////////////////
/
Blinker::Blinker(int r, int c) {
m_col = c;
m_row = r;
m_height = BLINKER_HEIGHT;
m_width = BLINKER_WIDTH;
//Allocate space for figure
m_figure = new char*[BLINKER_HEIGHT];
for (int i = 0; i
m_figure[i] = new char[BLINKER_WIDTH];
}
//Initialize figure
for (int i = 0; i
for (int j = 0; j
m_figure[i][j] = ALIVE;
}
}
}
Blinker::~Blinker() {
for (int i = 0; i
delete[] m_figure[i];
}
delete[] m_figure;
}
//////////////////////////////////////////////////////////////////////////
/
// Glider Implemention
//////////////////////////////////////////////////////////////////////////
/
Glider::Glider(int r, int c) {
m_col = c;
m_row = r;
m_height = GLIDER_SIZE;
m_width = GLIDER_SIZE;
// Allocate space for figure
m_figure = new char*[GLIDER_SIZE];
for (int i = 0; i
m_figure[i] = new char[GLIDER_SIZE];
}
// Initialize figure
for (int i = 0; i
for (int j = 0; j
m_figure[i][j] = DEAD;
}
}
m_figure[0][1] = ALIVE;
m_figure[1][2] = ALIVE;
m_figure[2][0] = ALIVE;
m_figure[2][1] = ALIVE;
m_figure[2][2] = ALIVE;
}
Glider::~Glider() {
for (int i = 0; i
delete[] m_figure[i];
}
delete[] m_figure;
}
//////////////////////////////////////////////////////////////////////////
/
// World Implemention
//////////////////////////////////////////////////////////////////////////
/
World::World() :
m_toggle(true)
{
m_world = new char*[MAX_ROWS];
m_otherWorld = new char*[MAX_ROWS];
for (char i = 0; i
m_world[i] = new char[MAX_COLS];
m_otherWorld[i] = new char[MAX_COLS];
}
for (char i = 0; i
for (char j = 0; j
m_world[i][j] = DEAD;
}
}
}
World::~World() {
for (char i = 0; i
delete[] m_world[i];
delete[] m_otherWorld[i];
}
delete[] m_world;
delete[] m_otherWorld;
}
void World::print() const {
clearScreen();
if (m_toggle) {
for (char i = 0; i
for (char j = 0; j
std::cout
}
std::cout
}
}
else {
for (char i = 0; i
for (char j = 0; j
std::cout
}
std::cout
}
}
for (char i = 0; i
std::cout
}
std::cout
}
bool World::hasWorldChanged() const {
for (char i = 0; i
for (char j = 0; j
if (m_otherWorld[i][j] != m_world[i][j]) {
return true;
}
}
}
return false;
}
void World::update() {
if (m_toggle) {
for (char i = 0; i
for (char j = 0; j
m_otherWorld[i][j] =
_getState(m_world[i][j], i,
j, m_toggle);
}
}
m_toggle = !m_toggle;
}
else {
for (char i = 0; i
for (char j = 0; j
m_world[i][j] =
_getState(m_otherWorld[i][j], i, j, m_toggle);
}
}
m_toggle = !m_toggle;
}
}
char World::_getState(char state, char row, char col, bool toggle) {
int yCoord = row;
int xCoord = col;
char neighbors = 0;
if (toggle) {
for (char i = yCoord - 1; i
for (char j = xCoord - 1; j
j++) {
if (i == yCoord && j == xCoord) {
continue;
}
if (i > -1 && i -1 &&
j
if (m_world[i][j] == ALIVE)
{
neighbors++;
}
}
}
}
}
else {
for (char i = yCoord - 1; i
for (char j = xCoord - 1; j
j++) {
if (i == yCoord && j == xCoord) {
continue;
}
if (i > -1 && i -1 &&
j
if (m_otherWorld[i][j] ==
ALIVE) {
neighbors++;
}
}
}
}
}
if (state == ALIVE) {
return (neighbors > 1 && neighbors
}
else {
return (neighbors == 3) ? ALIVE : DEAD;
}
}
bool World::addLife(Life *life) {
if (life == nullptr) {
cout
return false;
}
// Should check life extents with world bounds.
for (char i = life->getRow(); i - life->getRow()
life->getHeight(); i++) {
for (char j = life->getCol(); j - life->getCol()
life->getWidth(); j++) {
if (i
m_world[i][j] =
life->getFromFigure(i -
life->getRow(), j - life->getCol());
}
}
}
return true;
}
//////////////////////////////////////////////////////////////////////////
/
// Game Implemention
//////////////////////////////////////////////////////////////////////////
/
Game::Game(Life **life, int numLife) {
m_steps = 0;
m_automate = false;
m_world = new World();
if (life != nullptr) {
for (int i = 0; i
if (life[i] != nullptr) {
bool success =
m_world->addLife(life[i]);
if (!success) {
cout
to the world"
}
}
}
}
}
Game::~Game() {
delete m_world;
}
void Game::play()
{
while (true)
{
m_world->print();
if (!m_world->hasWorldChanged()) {
cout
game."
return;
}
else {
if (!m_automate) {
cout
to see stats, to automate, to quit): ";
string action;
getline(cin, action);
switch (action[0])
{
default:
cout
beep
continue;
case 'q':
cout
endl;
return;
case 's':
continue;
case ' ':
break;
case 'a':
m_automate = true;
break;
}
}
else {
if (m_steps >= MAX_STEPS) {
cout
quitting."
return;
}
delay(500);
}
m_steps++;
}
m_world->update();
}
}
//////////////////////////////////////////////////////////////////////////
/
// Main
//////////////////////////////////////////////////////////////////////////
/
int main() {
Life **population = new Life*[2];
// Polymorphism: Don't worry about understanding this.
population[0] = new Glider(0, 0); // Blinker and Glider
population[1] = new Blinker(4, 5);
int numLife = 2;
//population[0] = new Blinker(4, 5); //blinker alone
//int numLife = 1;
//population[0] = new Glider(0,0); //glider alone
//int numLife = 1;
// Create the game
Game g(population, numLife);
// Run the game
g.play();
// Short Answer
report();
// Clean up
delete population[0];
delete population[1];
delete[] population;
}
void report() {
string msg = "Hello World!"; // Replace Hello World with your
answer.
cout
}
// DO NOT MODIFY ANY CODE BETWEEN HERE AND THE END OF THE FILE!!!
// THE CODE IS SUITABLE FOR VISUAL C++, XCODE, AND g++ UNDER LINUX.
#include
#include
void delay(int ms) {
std::this_thread::sleep_for(std::chrono::milliseconds(ms));
}
//////////////////////////////////////////////////////////////////////////
/
// clearScreen implementation
//////////////////////////////////////////////////////////////////////////
/
// Note to Xcode users: clearScreen() will just write a newline instead
// of clearing the window if you launch your program from within Xcode.
// That's acceptable. (The Xcode output window doesn't have the
capability
// of being cleared.)
#ifdef _MSC_VER // Microsoft Visual C++
#include
void clearScreen()
{
HANDLE hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
CONSOLE_SCREEN_BUFFER_INFO csbi;
GetConsoleScreenBufferInfo(hConsole, &csbi);
DWORD dwConSize = csbi.dwSize.X * csbi.dwSize.Y;
COORD upperLeft = { 0, 0 };
DWORD dwCharsWritten;
FillConsoleOutputCharacter(hConsole, TCHAR(' '), dwConSize,
upperLeft,
&dwCharsWritten);
SetConsoleCursorPosition(hConsole, upperLeft);
}
#else // not Microsoft Visual C++, so assume UNIX interface
#include
#include
#include
void clearScreen() // will just write a newline in an Xcode output window
{
static const char* term = getenv("TERM");
if (term == nullptr || strcmp(term, "dumb") == 0)
std::cout
else
{
static const char* ESC_SEQ = "\x1B["; // ANSI Terminal
esc seq: ESC [
std::cout
std::flush;
}
}
#endif
CS 20A: Data Structures with C++ Project 1 Specification Santa Monica College Spring 2019 Santa Monica College Spring 2019 CS 20A: Data Structures wth C Project 1 Specification Programming Project Conway's Game of Life Submission: Submit via canvas one zip file containing only the 13 source filcs produced for this project do not include any IDE specific project files: Overview life h life.cpp blinker.cpp glider.cpp world.cpp game.cpp utils.cpp main.cpp blinker.h liderh world.h game.h globals.h The goal of the project is to organize the code according to the multiple file etiquette we discussed in class Which involves separaring the single provided source into the specified files There might be many language details that are unfamiliar to you. That is complenely fine, we will discuss them in time, and you do not need to know anything about them to complete this project. In addition to File Etiquette and Class Implementation, there are several aspects of programming that this project stresses The aip file should be named lastname id> aip;for example nguyen 123456.ip. Working with unfamiliar code and being able to get a general understanding of the overall flow of execution. Using the IDE's debuger can help in this regard using breakpoints Figuring out what is being specified, and for what aspect of the project the given information relates. Read everything first and devise a plan, as you become more familar with what you need to achieve modify the plan accondingy. If I take these 13 filles, I must be able to compile them using VS2017without any emors or warnings Be sure to you do not introduce any compilation or linking errors Work incrementally, compak often and submit regularly, your previous subemissions will be overaritten on canvas. What you submit must compile, incomplete code that works is always better than any code that does not compile negardlless of how "correct" most of the implementations an. Additional Considerations Other than in the fumction report,you u should not make any actual changes to any of the functions Organize the code: or classes, you just need to move them around. The word friend and the word sequence pragma once must not appear in any of the files you submit Following what we disecussed on mulltiple file etiquette take the single source file provided, and divide it into appropriate header files and implementation fikes, one pair of files for each class. Place the main routine in its own file named Tain.cpp. Make sure each file #indudes the headers it needs. Each header file must have include guards. . Your program must not use any global variables whose values may change during exccution. Global constants (eg. MAXRows) are all right Hints: Now what about the global constants? Place them in their own header file named globals.h. And what abour uniiry fanctions like delay, clearScreen, or repot? Pace them in their own implementation file named utilscpp, and place their prototype declarations in globals.h Start with making sure the original source compiles, then spend some time playing around with the code to try to get a sense of what is going on. Feel free to go cray, you can always re-download the source. Start at the "top", the main function. See what objects are created and then take a look at those objects' construction/member function calls. It is ok if you don't completely understand what is going on, there is some funky inheritance action going which we'll discuss in detail later. The first ching you should do is make sare that the single source compiles as is. Play around with it to get comfortable the program. The program implements Comaay's Game of Life . If we replace your main.cpp fik with the following, the program must build sucocessfully under both either alive or dead based simple rules hasod on under population or overcroweling Visual C++ and clangt+ #include #include +include include "game . h. "game . h. "world.h" world.h Analysis: Get a high level understanding of the behavior of the program (You do not need to know anything about inheritance or polymorphism at this point). How do we go about denenmining if a "birth" has occurred in any particular cell of the world? In other words, using the information we have available (variables) what is the logic in identifying a birth occurring in any cell on any day? Note, that a cell being alive on any particular day does not imply that was birthed. In what class and in which member function of that class can we obtain that information? #include include include *include include #include include int main "life,h" 'blinker.h "blinker. "glider,h" "gliderh" "globals. "globals.h You should notice that the utility function report does not do anything useful other than printing "Hello World to the console. Replace "Hcllo Wordld with a brief statement (a few sentences) with your ansaer to the above questions CS 20A: Data Structures with C++ Project 1 Specification Santa Monica College Spring 2019 Santa Monica College Spring 2019 CS 20A: Data Structures wth C Project 1 Specification Programming Project Conway's Game of Life Submission: Submit via canvas one zip file containing only the 13 source filcs produced for this project do not include any IDE specific project files: Overview life h life.cpp blinker.cpp glider.cpp world.cpp game.cpp utils.cpp main.cpp blinker.h liderh world.h game.h globals.h The goal of the project is to organize the code according to the multiple file etiquette we discussed in class Which involves separaring the single provided source into the specified files There might be many language details that are unfamiliar to you. That is complenely fine, we will discuss them in time, and you do not need to know anything about them to complete this project. In addition to File Etiquette and Class Implementation, there are several aspects of programming that this project stresses The aip file should be named lastname id> aip;for example nguyen 123456.ip. Working with unfamiliar code and being able to get a general understanding of the overall flow of execution. Using the IDE's debuger can help in this regard using breakpoints Figuring out what is being specified, and for what aspect of the project the given information relates. Read everything first and devise a plan, as you become more familar with what you need to achieve modify the plan accondingy. If I take these 13 filles, I must be able to compile them using VS2017without any emors or warnings Be sure to you do not introduce any compilation or linking errors Work incrementally, compak often and submit regularly, your previous subemissions will be overaritten on canvas. What you submit must compile, incomplete code that works is always better than any code that does not compile negardlless of how "correct" most of the implementations an. Additional Considerations Other than in the fumction report,you u should not make any actual changes to any of the functions Organize the code: or classes, you just need to move them around. The word friend and the word sequence pragma once must not appear in any of the files you submit Following what we disecussed on mulltiple file etiquette take the single source file provided, and divide it into appropriate header files and implementation fikes, one pair of files for each class. Place the main routine in its own file named Tain.cpp. Make sure each file #indudes the headers it needs. Each header file must have include guards. . Your program must not use any global variables whose values may change during exccution. Global constants (eg. MAXRows) are all right Hints: Now what about the global constants? Place them in their own header file named globals.h. And what abour uniiry fanctions like delay, clearScreen, or repot? Pace them in their own implementation file named utilscpp, and place their prototype declarations in globals.h Start with making sure the original source compiles, then spend some time playing around with the code to try to get a sense of what is going on. Feel free to go cray, you can always re-download the source. Start at the "top", the main function. See what objects are created and then take a look at those objects' construction/member function calls. It is ok if you don't completely understand what is going on, there is some funky inheritance action going which we'll discuss in detail later. The first ching you should do is make sare that the single source compiles as is. Play around with it to get comfortable the program. The program implements Comaay's Game of Life . If we replace your main.cpp fik with the following, the program must build sucocessfully under both either alive or dead based simple rules hasod on under population or overcroweling Visual C++ and clangt+ #include #include +include include "game . h. "game . h. "world.h" world.h Analysis: Get a high level understanding of the behavior of the program (You do not need to know anything about inheritance or polymorphism at this point). How do we go about denenmining if a "birth" has occurred in any particular cell of the world? In other words, using the information we have available (variables) what is the logic in identifying a birth occurring in any cell on any day? Note, that a cell being alive on any particular day does not imply that was birthed. In what class and in which member function of that class can we obtain that information? #include include include *include include #include include int main "life,h" 'blinker.h "blinker. "glider,h" "gliderh" "globals. "globals.h You should notice that the utility function report does not do anything useful other than printing "Hello World to the console. Replace "Hcllo Wordld with a brief statement (a few sentences) with your ansaer to the above questionsStep by Step Solution
There are 3 Steps involved in it
Step: 1
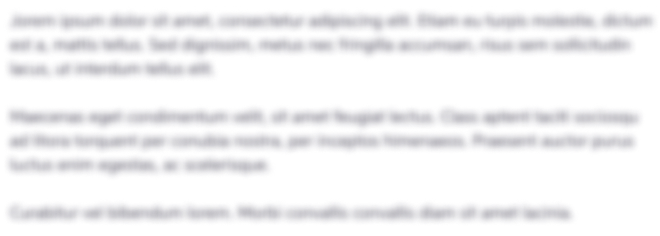
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started