Question
#include #include // malloc #include // srand #include // optarg char **create_board(int board_sz); void print_board(char **Board, int board_sz); int is_draw(char **Board, int board_sz); char winning_move(char
#include
#include
#include
#include
char **create_board(int board_sz);
void print_board(char **Board, int board_sz);
int is_draw(char **Board, int board_sz);
char winning_move(char **Board, int board_sz, int row_index, int col_index);
int play_tictactoe(int board_sz, int computer_enabled );
// Prints the Board
void print_board(char **Board, int board_sz)
{
printf(" ");
for (int i = 0; i < board_sz; i++)
{
printf("|%d", i); // column
}
printf("| ");
for (int i = 0; i < board_sz; i++)
{
printf("%c", 'a' + i); // row
for (int j = 0; j < board_sz; j++)
{
printf("|%c", Board[i][j]); // index for each square
}
printf("| ");
}
}
// Creates nxn tic tac toe Board
char **create_board(int board_sz)
{
char **Board = (char **)malloc(board_sz * sizeof(char *));
for (int i = 0; i < board_sz; i++)
{
Board[i] = (char *)malloc(board_sz * sizeof(char));
}
for (int i = 0; i < board_sz; i++)
{
for (int j = 0; j < board_sz; j++)
{
Board[i][j] = ' ';
}
}
return Board;
}
// Returns true if the game is a draw
int is_draw(char **Board, int board_sz)
{
for (int i = 0; i < board_sz; i++)
{
for (int j = 0; j < board_sz; j++)
{
if (Board[i][j] == ' ')
{
// empty square, so game ain't over yet
return 0;
}
}
}
// no empty squares, so it's a draw
return 1;
}
// Returns 'X' if (i,j) was a winning move for X
// Returns 'O' if (i,j) was a winning move for O
// Returns ASCII value 0 otherwise
char winning_move(char **Board, int board_sz, int row_index, int col_index)
{
int win = 1;
// check row
for (int k = 0; k < board_sz; k++)
{
if (Board[row_index][k] != Board[row_index][col_index])
{
win = 0;
break;
}
}
if (win) // means Board[i][k] == Board[i][j]
{
return Board[row_index][col_index];
}
// check column
win = 1;
for (int k = 0; k < board_sz; k++)
{
if (Board[k][col_index] != Board[row_index][col_index])
{
win = 0;
break;
}
}
if (win)
{
return Board[row_index][col_index];
}
// check forward diagonal
win = 1;
for (int k = 0; k < board_sz; k++)
{
if (Board[k][k] != Board[row_index][col_index])
{
win = 0;
break;
}
}
if (win)
{
return Board[row_index][col_index];
}
// check reverse diagonal
win = 1;
for (int k = 0; k < board_sz; k++)
{
if (Board[k][board_sz - k - 1] != Board[row_index][col_index])
{
win = 0;
break;
}
}
if (win)
{
return Board[row_index][col_index];
}
// got nothing
return 0;
}
// main function where program start
int main(int argc, char **argv)
{
int opt;
int board_sz = 3;
int computer_enabled;
srand(time(NULL));
// Parse command line arguments using getopt()
while ((opt = getopt(argc, argv, "s:i")) != -1)
{
switch (opt)
{
case 's':
board_sz = atoi(optarg);
break;
case 'i':
computer_enabled = 1;
break;
default:
break;
}
}
play_tictactoe(board_sz, computer_enabled);
return 0;
}
int play_tictactoe(int board_sz, int computer_enabled ){
char **Board = create_board(board_sz);
char winner = '\0';
char row;
char col;
char turn = 'X';
// standard game loop
while (!winner && !is_draw(Board, board_sz))
{
print_board(Board, board_sz);
if (turn == 'X' || !computer_enabled)
{
printf("computer 'O' Moves are 'enabled' ");
printf("-Player's %c turn (qq to quit) ", turn);
// suggestion
do
{
row = rand() % board_sz + 'a'; //
col = rand() % board_sz + '0';
} while (Board[row - 'a'][col - '1'] != ' ');
printf("*---> suggestion:\t(%c %c) ", row, col);
printf("(X) Enter Move (row column) ---------------------------> ");
fflush(stdout);
scanf(" %c %c", &row, &col);
// quit when enter qq.
if (row == 'q' && col == 'q'){
exit(0);
}
}
else
{
printf("computer 'O' Moves are 'enabled' ");
printf("*Player's O turn (qq to quit) ");
// Randomly pick a move
do
{
row = rand() % board_sz + 'a'; //
col = rand() % board_sz + '0';
} while (Board[row - 'a'][col - '1'] != ' ');
printf("(O) computer Picks (%c %c) (hit a key to continue) ----> ", row, col);
}
// Make move if square is free
int rowind = row - 'a';
int colind = col - '0';
if (rowind >= board_sz || colind >= board_sz)
{
printf("Invalid move ");
}
else if (Board[rowind][colind] == ' ')
{
char enter;
enter = getchar();
printf("Move is %c %c (%d, %d) ", row, col, rowind, colind);
Board[rowind][colind] = turn;
if (turn == 'X')
{
turn = 'O';
}
else
{
turn = 'X';
}
winner = winning_move(Board, board_sz, rowind, colind);
}
else
{
printf("Square is occupied; try again. ");
}
}
// Game over - print Board & declare finish
print_board(Board, board_sz);
if (winner == 'X' || winner == 'O')
{
printf("Congratulations %c! ", winner);
}
else
{
printf("Game ends in a draw. ");
}
return 0;
}
this is the tic tac toe game code in c.
(you can turn this code with command line input ex) ./ttt -s 4 -i)
I'd like to split it into a header file(.h) and a source file(.c)
I need at least two .c file here and .h file for each .c file.
*.c (should have at least 2 .c files)
*.h (should have at least 1 .h file)
please make those files and explain please. showing results would be great. thank you.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
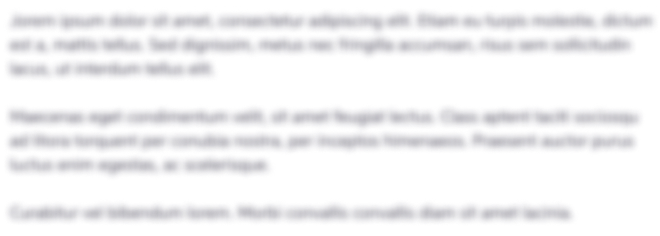
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started