Question
#include #include using namespace std; //Prototypes for the functions that you will write int CharToInt(char v); char IntToChar(int v); int StringToInt(string val); string IntToString(int val);
#include#include using namespace std; //Prototypes for the functions that you will write int CharToInt(char v); char IntToChar(int v); int StringToInt(string val); string IntToString(int val); int main(int argc, char *argv[]) { string sresult; int left; int right; char op; if (4 != argc) { printf("Usage: %s ", argv[0]); return -1; } //Notice that this calls your StringToInt. So, make sure you debug //StringToInt() to make sure that left and right get a proper //value. left = StringToInt(argv[1]); right = StringToInt(argv[3]); op = argv[2][0]; //Calculate based on the op. Notice that this calls IntToString, //so debug your IntToString() function to make sure that sresult //is given the proper result. This assumes your StringToInt already //works. switch (op) { case 'x': sresult = IntToString(left * right); break; case '/': sresult = IntToString(left / right); break; case '+': sresult = IntToString(left + right); break; case '-': sresult = IntToString(left - right); break; case '%': sresult = IntToString(left % right); break; default: sresult = IntToString(left); break; } //Remember, printf is the only output function you may use for this lab! //The format string is %d %c %d = %s. This means that the first argument //is %d (decimal / integer), %c (character), %d (decimal /integer), //%s (string). Notice that because printf() is a C-style function, you //must pass strings as character arrays. We can convert a C++ string //to a character array (C-style string) by using the c_str() member function. printf("%d %c %d = %s ", left, op, right, sresult.c_str()); return 0; }
---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Write four functions: IntToString, StringToInt, CharToInt, IntToChar, which are already prototyped in the above template.
CharToInt
This function will take a single, positive character between the values 0 - 9 and return the single digit integer equivalent. For example, '0' will return 0 and '8' will return 8 (as an 8-bit integer).
IntToChar
This function will take a single digit, positive integer and return the character equivalent. This is essentially the reverse of CharToInt. For example, 0 will return '0', 8 will return '8'.
IntToString
This function will take any sized integer, including negative numbers, and convert it into a string. For example, -12345678 will return "-12345678".
StringToInt
This function will take a string and convert it to the equivalent number, including negative numbers. For example, "-123456" will return -123456.
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Restrictions / Hints
1. You may NOT use string streams for this lab.
2. You MUST use the given template.
3. You may ONLY write the four prototyped functions: CharToInt, IntToChar, StringToInt, IntToString.
4. You may NOT call any C++ library function from within your four functions. However, you may call one of your other four functions.
5. No error checking is required.
Compiling
You will be programming a COSC102-type problem to review writing C++ programs and to test your build environment. You will be using a new function called "printf. This is a function typically used in C (not C++). This allows you to print to the console using "format" tags, which start with a percent sign: % and a letter to control what data type is being output For example: printf("%s %d %ld Xc %fX1fin", "A string", 123, 1234L, .., 7.89, 7.89); In the example above: The suffix L (for 1234L) tells C++ to generate a 64-bit integer literal. Without a suffix, C++ produces a 32-bit integer literal The suffix F (for 7.89F) tells C++ to generate a 32-bit floating point. Without a suffix, C++ produces a 64-bit floating point (double). sPrints a C-style string (character array) %d -Prints a 32-bit integer (int) 1d - Prints a 64-bit integer (long) 6c Prints a single character (char) %f - Prints a 32-bit float (float) %lf - Prints a 64-bit float (double) Use the following template for your lab1a.cpp: You MUST build your program using the following command to make sure your program is correct: g++ -std-c++17 -Wall -Wextra -pedantic -Werror -o lab1 lab1.cpp The following command (notice the riscv64- prefix) will test to see if you set up your build environment properly and see if the RISC-V tools are working properly. riscv64-g++ -std-c++17-Wall -Wextra -pedantic -Werror -o lab1 lab1.cpp Both of the commands above should work properly. If they don't, make sure that you have set up your paths correctly. Then execute your program by typing: ./labla 2 -8 This should print the following to the screer: 2-8 -6 Try the following to test your code (NOTE: this doesn't test everything!) /lab1 8 8 ./lab1 8 x 2 ./lab1 2 x 0 ./lab1 1234 18
Step by Step Solution
There are 3 Steps involved in it
Step: 1
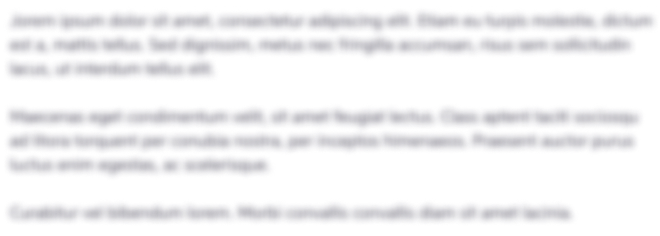
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started