Question
Include the methods size(), clear(), and isEmpty() in your List interface: /** @return the size of this List */ int size(); /** Clear this List
Include the methods size(), clear(), and isEmpty() in your List interface:
/** @return the size of this List */
int size();
/** Clear this List */
void clear();
/** @return true iff this List is empty */
boolean isEmpty();
Create a new package in your dsa project, listDriver, and copy HomeworkArrayListDriver to this package.
Implement these methods in your ArrayList class, and test your solution using HomeworkArrayListDriver. >> >> >> CODE TO BE MODIFIED BELOW: >>>>>> LIST INTERFACE
package list;
public interface List
>>>>>> >>>>>>> ARRAYLIST CLASS
package list;
public class ArrayList
public E set(int ndx, E value) { E result = values[ndx]; values[ndx] = value; return result; }
public void add(E value) { add(size,value); }
public void add(int ndx, E value) { if (values.length == size) alloc(); for(int i = size; i > ndx; i--) values[i] = values[i-1]; values[ndx] = value; size++; } private void alloc() { E[] tempArray = (E[]) new Object[2*values.length]; for(int i = 0; i < size; i++) tempArray[i] = values [i]; values = tempArray; } public E remove(int ndx) { E result = values[ndx]; for(int i = ndx; i < size-1; i++) values[i] = values [i + 1]; size--; return result; } } >>>> >>>> >>>> HOMEWORKARRAYLIST DRIVER:
package listDriver;
import list.*;
public class HomeworkArrayListDriver
{
public static void main()
{
List
System.out.println ("Testing ArrayLists");
names = new ArrayList
names.add ("jim");
names.add ("mary");
names.add ("joe");
names.add ("sue");
System.out.println (names.get(2)); // Should be joe
names.set (2, "Joe");
System.out.println (names.get(2)); // Should be Joe
System.out.println (names.size()); // Should be 4
names.remove (0);
System.out.println (names.size()); // Should be 3
names.add(0,"joe");
System.out.println (names.size()); // Should be 4
System.out.println (names.get(3)); // Should be sue
if (names.isEmpty())
System.err.println ("Incorrect, error in isEmpty");
names.clear();
if (!names.isEmpty())
System.err.println ("Incorrect, error in isEmpty or clear");
names.add("sue");
for (int i=0; i<20; i++)
names.add ("sue" + i); // Add 20 more strings
if (! names.get(20).equals("sue19"))
System.err.println ("Error, possibly in alloc");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
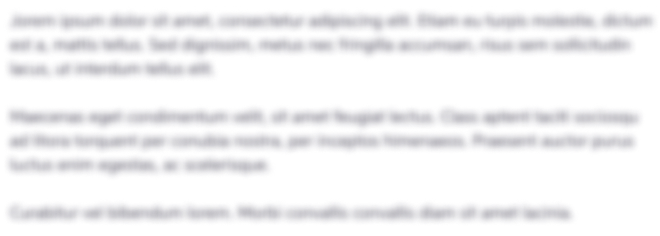
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started