Question
#include using namespace std; struct BinNode { int key; BinNode *left; BinNode *right; }; class myBST { public: myBST () { root = NULL; }
#include
using namespace std;
struct BinNode {
int key;
BinNode *left;
BinNode *right;
};
class myBST {
public:
myBST () { root = NULL; }
~myBST ();
BinNode* findInBST(int k); // return NULL if not found
void insertToBST(int k);
void preOrderTraversal();
void postOrderTraversal();
void inOrderTraversal();
void rotatedPrintTree();
private:
BinNode* root;
void free_helper(BinNode* node);
// BinNode* find_helper(BinNode* node, int k); //optional helper function for findInBST
// void insert_helper(BinNode* node, int k); //optional helper function for insertToBST
void preOrder(BinNode* node);
void postOrder(BinNode* node);
void inOrder(BinNode* node);
void rotatedPrint(BinNode* node, int d);
};
myBST::~myBST() {
free_helper(root);
}
void myBST::free_helper(BinNode* node) {
if(node != NULL) {
free_helper(node->left);
free_helper(node->right);
delete node;
}
}
// //***************************
// //recursive implementation***
// //***************************
// BinNode* myBST::findInBST(int k) {
// }
// BinNode* myBST::find_helper(BinNode* node, int k) {
// }
//*******************************
/on-recursive implementation***
//*******************************
BinNode* myBST::findInBST(int k) {
}
// //***************************
// //recursive implementation***
// //***************************
// void myBST::insertToBST(int k) {
// }
// void myBST::insert_helper(BinNode* node, int k) {
// }
//*******************************
/on-recursive implementation***
//*******************************
void myBST::insertToBST(int k) {
}
//***********************************
//preOrderTraversal implementation***
//***********************************
void myBST::preOrderTraversal() {
preOrder(root);
cout
}
void myBST::preOrder(BinNode* node) {
}
//************************************
//postOrderTraversal implementation***
//************************************
void myBST::postOrderTraversal() {
postOrder(root);
cout
}
void myBST::postOrder(BinNode* node) {
}
//**********************************
//inOrderTraversal implementation***
//**********************************
void myBST::inOrderTraversal() {
inOrder(root);
cout
}
void myBST::inOrder(BinNode* node) {
}
//***********************************
//print the tree (left rotated)******
//***********************************
void myBST::rotatedPrintTree() {
rotatedPrint(root, 0);
}
void myBST::rotatedPrint(BinNode* node, int d) {
if(node == NULL)
return;
if (node->right != NULL)
rotatedPrint(node->right, d+1);
for(int i = 0; i
cout
cout key
if (node->left != NULL)
rotatedPrint(node->left, d+1);
}
int main()
{
myBST testTree;
int user_input = 0;
while (user_input != -1) {
cout
cout
cout
cin >> user_input;
if (user_input >= 0 and user_input
testTree.insertToBST(user_input);
else if (user_input != -1)
cout
}
cout
testTree.rotatedPrintTree();
cout
testTree.preOrderTraversal();
cout
testTree.postOrderTraversal();
cout
testTree.inOrderTraversal();
user_input = 0;
while (user_input != -1) {
cout
cout
cout
cin >> user_input;
if (user_input >= 0 and user_input
BinNode* temp = testTree.findInBST(user_input);
if(temp == NULL)
cout
else {
cout
if(temp->left != NULL)
cout left->key
if(temp->right != NULL)
cout right->key
}
}
else if (user_input != -1)
cout
}
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
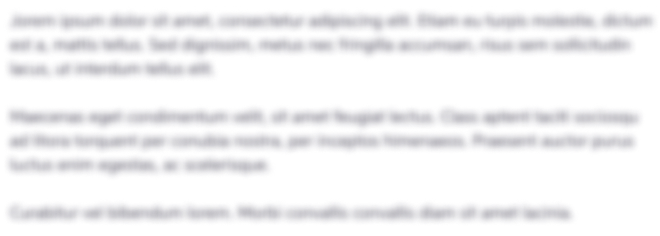
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started