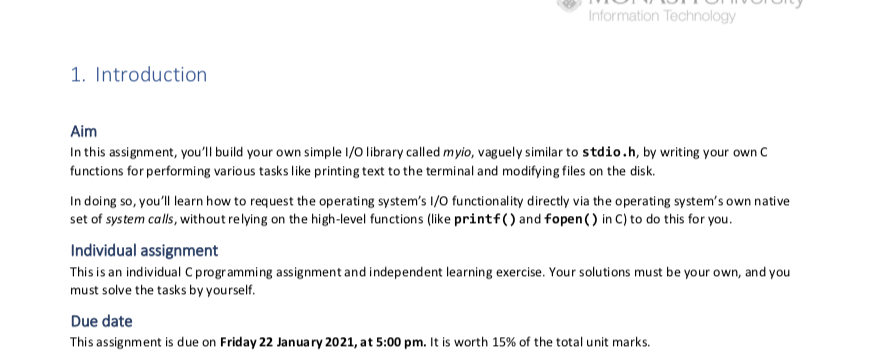
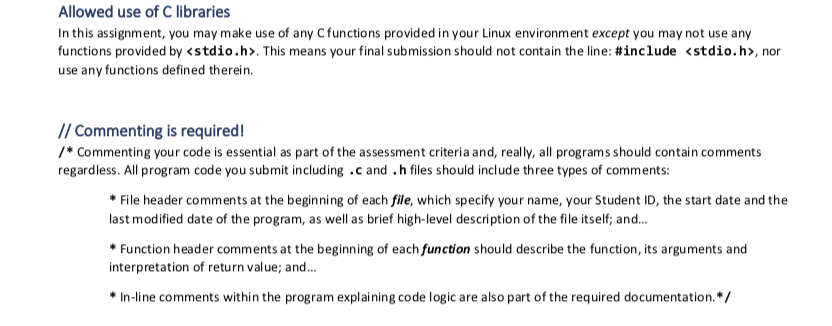
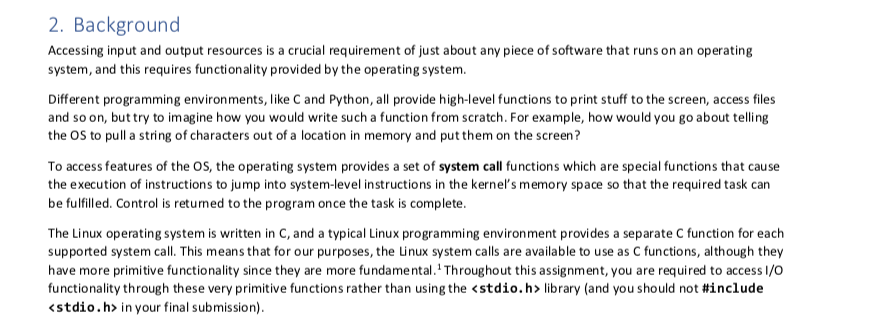
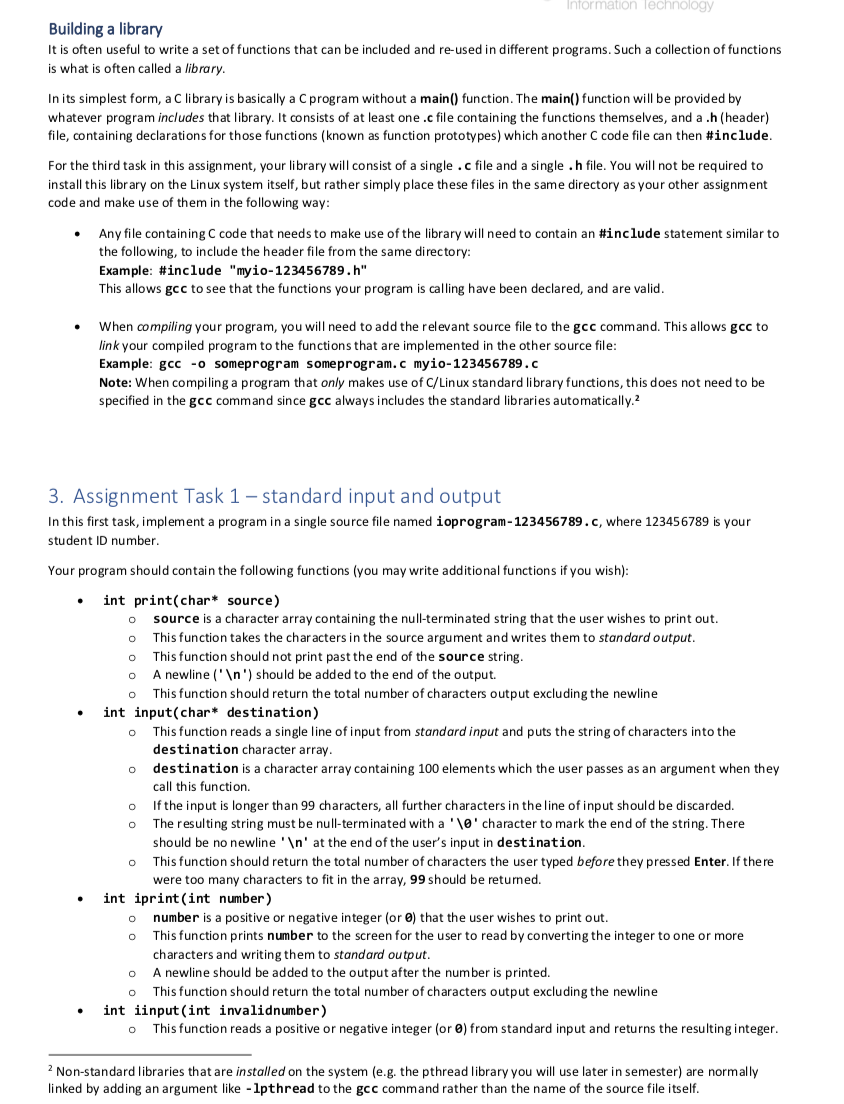
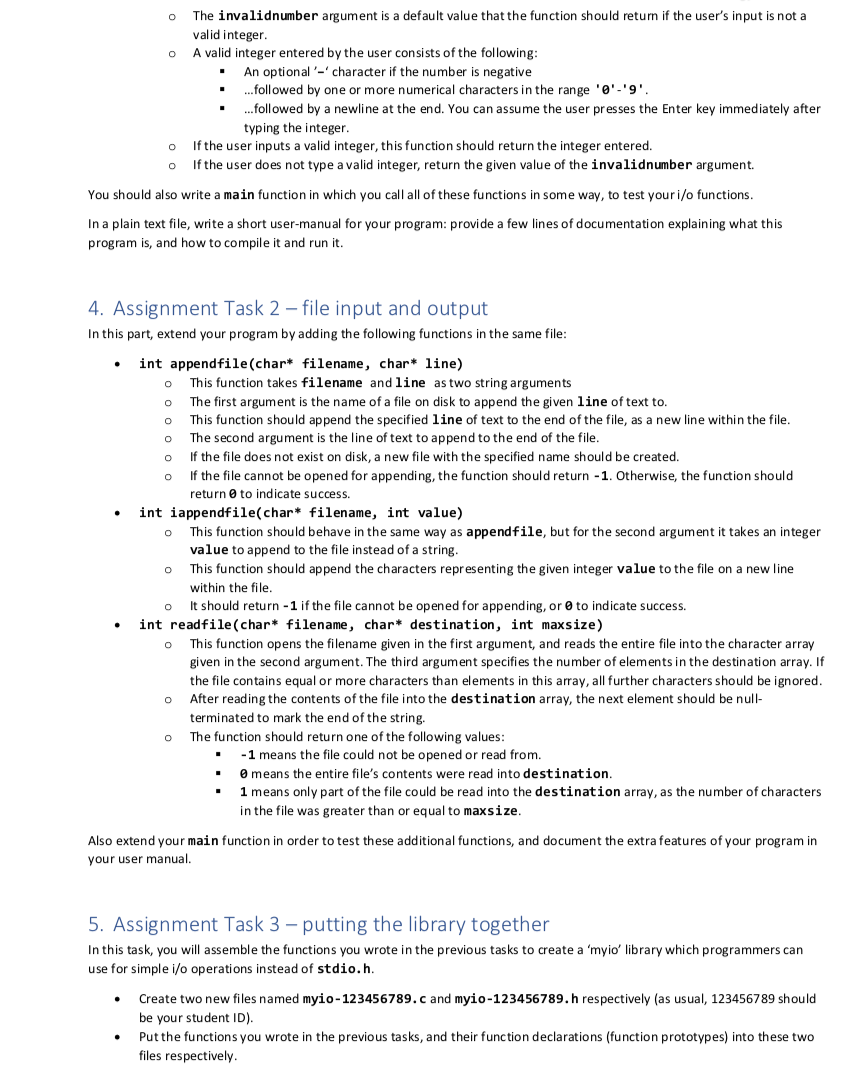
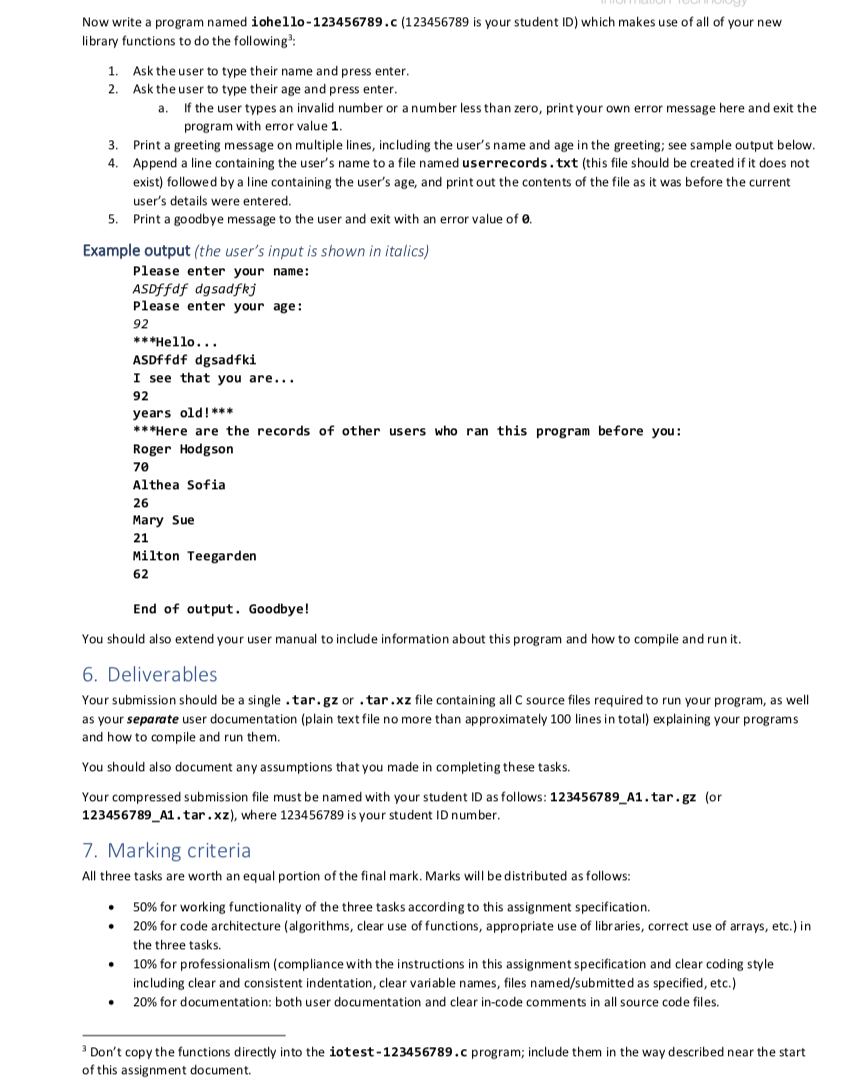
Information Technology 1. Introduction Aim In this assignment, you'll build your own simple 1/0 library called myio, vaguely similar to stdio.h, by writing your own functions for performing various tasks like printing text to the terminal and modifying files on the disk. In doing so, you'll learn how to request the operating system's 1/0 functionality directly via the operating system's own native set of system calls, without relying on the high-level functions (like printf() and fopen() in C) to do this for you. Individual assignment This is an individual programming assignment and independent learning exercise. Your solutions must be your own, and you must solve the tasks by yourself. Due date This assignment is due on Friday 22 January 2021, at 5:00 pm. It is worth 15% of the total unit marks. Allowed use of C libraries In this assignment, you may make use of any functions provided in your Linux environment except you may not use any functions provided by
. This means your final submission should not contain the line: #include , nor use any functions defined therein. // Commenting is required! /* Commenting your code is essential as part of the assessment criteria and, really, all programs should contain comments regardless. All program code you submit including .c and .h files should include three types of comments: * File header comments at the beginning of each file, which specify your name, your Student ID, the start date and the last modified date of the program, as well as brief high-level description of the file itself; and... * Function header comments at the beginning of each function should describe the function, its arguments and interpretation of return value; and... * In-line comments within the program explaining code logic are also part of the required documentation.* / 2. Background Accessing input and output resources is a crucial requirement of just about any piece of software that runs on an operating system, and this requires functionality provided by the operating system. Different programming environments, like C and Python, all provide high-level functions to print stuff to the screen, access files and so on, but try to imagine how you would write such a function from scratch. For example, how would you go about telling the OS to pull a string of characters out of a location in memory and put them on the screen? To access features of the OS, the operating system provides a set of system call functions which are special functions that cause the execution of instructions to jump into system-level instructions in the kernel's memory space so that the required task can be fulfilled. Control is returned to the program once the task is complete. The Linux operating system is written in C, and a typical Linux programming environment provides a separate function for each supported system call. This means that for our purposes, the Linux system calls are available to use as C functions, although they have more primitive functionality since they are more fundamental. Throughout this assignment, you are required to access I/O functionality through these very primitive functions rather than using the library (and you should not #include in your final submission). Information Technology Building a library It is often useful to write a set of functions that can be included and re-used in different programs. Such a collection of functions is what is often called a library. In its simplest form, a C library is basically a C program without a main() function. The main() function will be provided by whatever program includes that library. It consists of at least one .c file containing the functions themselves, and a .h (header) file, containing declarations for those functions (known as function prototypes) which another C code file can then #include. For the third task in this assignment, your library will consist of a single .c file and a single .h file. You will not be required to install this library on the Linux system itself, but rather simply place these files in the same directory as your other assignment code and make use of them in the following way: Any file containing C code that needs to make use of the library will need to contain an #include statement similar to the following, to include the header file from the same directory: Example: #include "myio-123456789.h" This allows gcc to see that the functions your program is calling have been declared, and are valid. . When compiling your program, you will need to add the relevant source file to the gcc command. This allows gec to link your compiled program to the functions that are implemented in the other source file: Example: gcc - someprogram someprogram.c myio-123456789.c Note: When compiling a program that only makes use of C/Linux standard library functions, this does not need to be specified in the gcc command since gcc always includes the standard libraries automatically.2 3. Assignment Task 1 - standard input and output In this first task, implement a program in a single source file named ioprogram-123456789.c, where 123456789 student ID number. your Your program should contain the following functions (you may write additional functions if you wish): O o O O o . O o int print(char* source) source is a character array containing the null-terminated string that the user wishes to print out. This function takes the characters in the source argument and writes them to standard output. This function should not print past the end of the source string. A newline (' ') should be added to the end of the output. This function should return the total number of characters output excluding the newline int input(char* destination) This function reads a single line of input from standard input and puts the string of characters into the destination character array. destination is a character array containing 100 elements which the user passes as an argument when they call this function. If the input is longer than 99 characters, all further characters in the line of input should be discarded. The resulting string must be null-terminated with a 'lo' character to mark the end of the string. There should be no newline ' ' at the end of the user's input in destination. This function should return the total number of characters the user typed before they pressed Enter. If there were too many characters to fit in the array, 99 should be returned. int iprint(int number) number is a positive or negative integer (or) that the user wishes to print out. This function prints number to the screen for the user to read by converting the integer to one or more characters and writing them to standard output. A newline should be added to the output after the number is printed. o This function should return the total number of characters output excluding the newline int iinput(int invalidnumber) This function reads a positive or negative integer (or ) from standard input and returns the resulting integer. o O . O O O 2 Non-standard libraries that are installed on the system (e.g. the pthread library you will use later in semester) are normally linked by adding an argument like -lpthread to the gcc command rather than the name of the source file itself. O o The invalidnumber argument is a default value that the function should return if the user's input is not a valid integer. A valid integer entered by the user consists of the following: An optional '-' character if the number is negative ...followed by one or more numerical characters in the range '0'-'9'. ...followed by a newline at the end. You can assume the user presses the Enter key immediately after typing the integer. If the user inputs a valid integer, this function should return the integer entered. If the user does not type a valid integer, return the given value of the invalidnumber argument. . 0 You should also write a main function in which you call all of these functions in some way, to test your i/o functions. In a plain text file, write a short user-manual for your program: provide a few lines of documentation explaining what this program is, and how to compile it and run it. 4. Assignment Task 2 - file input and output In this part, extend your program by adding the following functions in the same file: . 0 0 0 . o o int appendfile(char* filename, char* line) This function takes filename and line as two string arguments The first argument is the name of a file on disk to append the given line of text to. This function should append the specified line of text to the end of the file, as a new line within the file. The second argument is the line of text to append to the end of the file. If the file does not exist on disk, a new file with the specified name should be created. If the file cannot be opened for appending, the function should return - 1. Otherwise, the function should return to indicate success. int iappendfile(char* filename, int value) This function should behave in the same way as appendfile, but for the second argument it takes an integer value to append to the file instead of a string. This function should append the characters representing the given integer value to the file on a new line within the file. It should return - 1 if the file cannot be opened for appending, or to indicate success. int readfile(char* filename, char* destination, int maxsize) This function opens the filename given in the first argument, and reads the entire file into the character array given in the second argument. The third argument specifies the number of elements in the destination array. If the file contains equal or more characters than elements in this array, all further characters should be ignored. After reading the contents of the file into the destination array, the next element should be null- terminated to mark the end of the string. The function should return one of the following values: - 1 means the file could not be opened or read from. means the entire file's contents were read into destination. 1 means only part of the file could be read into the destination array, as the number of characters in the file was greater than or equal to maxsize. O . o Also extend your main function in order to test these additional functions, and document the extra features of your program in your user manual. 5. Assignment Task 3-putting the library together In this task, you will assemble the functions you wrote in the previous tasks to create a 'myio' library which programmers can use for simple i/o operations instead of stdio.h. Create two new files named myio-123456789.c and myio-123456789.h respectively (as usual, 123456789 should be your student ID). Put the functions you wrote in the previous tasks, and their function declarations (function prototypes) into these two files respectively. Now write a program named iohello-123456789.c (123456789 is your student ID) which makes use of all of your new library functions to do the following: 1. Ask the user to type their name and press enter. 2. Ask the user to type their age and press enter. a. If the user types an invalid number or a number less than zero, print your own error message here and exit the program with error value 1. 3. Print a greeting message on multiple lines, including the user's name and age in the greeting; see sample output below. 4. Append a line containing the user's name to a file named userrecords.txt (this file should be created if it does not exist) followed by a line containing the user's age, and print out the contents of the file as it was before the current user's details were entered. 5. Print a goodbye message to the user and exit with an error value of 0. Example output (the user's input is shown in italics) Please enter your name: ASDffdf dgsadfkj Please enter your age: 92 ***Hello... ASDffdf dgsadfki I see that you are... 92 years old!*** ***Here are the records of other users who ran this program before you: Roger Hodgson 70 Althea Sofia 26 Mary Sue 21 Milton Teegarden 62 End of output. Goodbye! You should also extend your user manual to include information about this program and how to compile and run it. 6. Deliverables Your submission should be a single.tar.gz or .tar.xz file containing all source files required to run your program, as well as your separate user documentation (plain text file no more than approximately 100 lines in total) explaining your programs and how to compile and run them. You should also document any assumptions that you made in completing these tasks. Your compressed submission file must be named with your student ID as follows: 123456789_A1.tar.gz (or 123456789_A1.tar.xz), where 123456789 is your student ID number. 7. Marking criteria All three tasks are worth an equal portion of the final mark. Marks will be distributed as follows: 50% for working functionality of the three tasks according to this assignment specification. 20% for code architecture (algorithms, clear use of functions, appropriate use of libraries, correct use of arrays, etc.) in the three tasks. 10% for professionalism (compliance with the instructions in this assignment specification and clear coding style including clear and consistent indentation, clear variable names, files named/submitted as specified, etc.) 20% for documentation: both user documentation and clear in-code comments in all source code files. Don't copy the functions directly into the iotest-123456789.c program; include them in the way described near the start of this assignment document. Information Technology 1. Introduction Aim In this assignment, you'll build your own simple 1/0 library called myio, vaguely similar to stdio.h, by writing your own functions for performing various tasks like printing text to the terminal and modifying files on the disk. In doing so, you'll learn how to request the operating system's 1/0 functionality directly via the operating system's own native set of system calls, without relying on the high-level functions (like printf() and fopen() in C) to do this for you. Individual assignment This is an individual programming assignment and independent learning exercise. Your solutions must be your own, and you must solve the tasks by yourself. Due date This assignment is due on Friday 22 January 2021, at 5:00 pm. It is worth 15% of the total unit marks. Allowed use of C libraries In this assignment, you may make use of any functions provided in your Linux environment except you may not use any functions provided by . This means your final submission should not contain the line: #include , nor use any functions defined therein. // Commenting is required! /* Commenting your code is essential as part of the assessment criteria and, really, all programs should contain comments regardless. All program code you submit including .c and .h files should include three types of comments: * File header comments at the beginning of each file, which specify your name, your Student ID, the start date and the last modified date of the program, as well as brief high-level description of the file itself; and... * Function header comments at the beginning of each function should describe the function, its arguments and interpretation of return value; and... * In-line comments within the program explaining code logic are also part of the required documentation.* / 2. Background Accessing input and output resources is a crucial requirement of just about any piece of software that runs on an operating system, and this requires functionality provided by the operating system. Different programming environments, like C and Python, all provide high-level functions to print stuff to the screen, access files and so on, but try to imagine how you would write such a function from scratch. For example, how would you go about telling the OS to pull a string of characters out of a location in memory and put them on the screen? To access features of the OS, the operating system provides a set of system call functions which are special functions that cause the execution of instructions to jump into system-level instructions in the kernel's memory space so that the required task can be fulfilled. Control is returned to the program once the task is complete. The Linux operating system is written in C, and a typical Linux programming environment provides a separate function for each supported system call. This means that for our purposes, the Linux system calls are available to use as C functions, although they have more primitive functionality since they are more fundamental. Throughout this assignment, you are required to access I/O functionality through these very primitive functions rather than using the library (and you should not #include in your final submission). Information Technology Building a library It is often useful to write a set of functions that can be included and re-used in different programs. Such a collection of functions is what is often called a library. In its simplest form, a C library is basically a C program without a main() function. The main() function will be provided by whatever program includes that library. It consists of at least one .c file containing the functions themselves, and a .h (header) file, containing declarations for those functions (known as function prototypes) which another C code file can then #include. For the third task in this assignment, your library will consist of a single .c file and a single .h file. You will not be required to install this library on the Linux system itself, but rather simply place these files in the same directory as your other assignment code and make use of them in the following way: Any file containing C code that needs to make use of the library will need to contain an #include statement similar to the following, to include the header file from the same directory: Example: #include "myio-123456789.h" This allows gcc to see that the functions your program is calling have been declared, and are valid. . When compiling your program, you will need to add the relevant source file to the gcc command. This allows gec to link your compiled program to the functions that are implemented in the other source file: Example: gcc - someprogram someprogram.c myio-123456789.c Note: When compiling a program that only makes use of C/Linux standard library functions, this does not need to be specified in the gcc command since gcc always includes the standard libraries automatically.2 3. Assignment Task 1 - standard input and output In this first task, implement a program in a single source file named ioprogram-123456789.c, where 123456789 student ID number. your Your program should contain the following functions (you may write additional functions if you wish): O o O O o . O o int print(char* source) source is a character array containing the null-terminated string that the user wishes to print out. This function takes the characters in the source argument and writes them to standard output. This function should not print past the end of the source string. A newline (' ') should be added to the end of the output. This function should return the total number of characters output excluding the newline int input(char* destination) This function reads a single line of input from standard input and puts the string of characters into the destination character array. destination is a character array containing 100 elements which the user passes as an argument when they call this function. If the input is longer than 99 characters, all further characters in the line of input should be discarded. The resulting string must be null-terminated with a 'lo' character to mark the end of the string. There should be no newline ' ' at the end of the user's input in destination. This function should return the total number of characters the user typed before they pressed Enter. If there were too many characters to fit in the array, 99 should be returned. int iprint(int number) number is a positive or negative integer (or) that the user wishes to print out. This function prints number to the screen for the user to read by converting the integer to one or more characters and writing them to standard output. A newline should be added to the output after the number is printed. o This function should return the total number of characters output excluding the newline int iinput(int invalidnumber) This function reads a positive or negative integer (or ) from standard input and returns the resulting integer. o O . O O O 2 Non-standard libraries that are installed on the system (e.g. the pthread library you will use later in semester) are normally linked by adding an argument like -lpthread to the gcc command rather than the name of the source file itself. O o The invalidnumber argument is a default value that the function should return if the user's input is not a valid integer. A valid integer entered by the user consists of the following: An optional '-' character if the number is negative ...followed by one or more numerical characters in the range '0'-'9'. ...followed by a newline at the end. You can assume the user presses the Enter key immediately after typing the integer. If the user inputs a valid integer, this function should return the integer entered. If the user does not type a valid integer, return the given value of the invalidnumber argument. . 0 You should also write a main function in which you call all of these functions in some way, to test your i/o functions. In a plain text file, write a short user-manual for your program: provide a few lines of documentation explaining what this program is, and how to compile it and run it. 4. Assignment Task 2 - file input and output In this part, extend your program by adding the following functions in the same file: . 0 0 0 . o o int appendfile(char* filename, char* line) This function takes filename and line as two string arguments The first argument is the name of a file on disk to append the given line of text to. This function should append the specified line of text to the end of the file, as a new line within the file. The second argument is the line of text to append to the end of the file. If the file does not exist on disk, a new file with the specified name should be created. If the file cannot be opened for appending, the function should return - 1. Otherwise, the function should return to indicate success. int iappendfile(char* filename, int value) This function should behave in the same way as appendfile, but for the second argument it takes an integer value to append to the file instead of a string. This function should append the characters representing the given integer value to the file on a new line within the file. It should return - 1 if the file cannot be opened for appending, or to indicate success. int readfile(char* filename, char* destination, int maxsize) This function opens the filename given in the first argument, and reads the entire file into the character array given in the second argument. The third argument specifies the number of elements in the destination array. If the file contains equal or more characters than elements in this array, all further characters should be ignored. After reading the contents of the file into the destination array, the next element should be null- terminated to mark the end of the string. The function should return one of the following values: - 1 means the file could not be opened or read from. means the entire file's contents were read into destination. 1 means only part of the file could be read into the destination array, as the number of characters in the file was greater than or equal to maxsize. O . o Also extend your main function in order to test these additional functions, and document the extra features of your program in your user manual. 5. Assignment Task 3-putting the library together In this task, you will assemble the functions you wrote in the previous tasks to create a 'myio' library which programmers can use for simple i/o operations instead of stdio.h. Create two new files named myio-123456789.c and myio-123456789.h respectively (as usual, 123456789 should be your student ID). Put the functions you wrote in the previous tasks, and their function declarations (function prototypes) into these two files respectively. Now write a program named iohello-123456789.c (123456789 is your student ID) which makes use of all of your new library functions to do the following: 1. Ask the user to type their name and press enter. 2. Ask the user to type their age and press enter. a. If the user types an invalid number or a number less than zero, print your own error message here and exit the program with error value 1. 3. Print a greeting message on multiple lines, including the user's name and age in the greeting; see sample output below. 4. Append a line containing the user's name to a file named userrecords.txt (this file should be created if it does not exist) followed by a line containing the user's age, and print out the contents of the file as it was before the current user's details were entered. 5. Print a goodbye message to the user and exit with an error value of 0. Example output (the user's input is shown in italics) Please enter your name: ASDffdf dgsadfkj Please enter your age: 92 ***Hello... ASDffdf dgsadfki I see that you are... 92 years old!*** ***Here are the records of other users who ran this program before you: Roger Hodgson 70 Althea Sofia 26 Mary Sue 21 Milton Teegarden 62 End of output. Goodbye! You should also extend your user manual to include information about this program and how to compile and run it. 6. Deliverables Your submission should be a single.tar.gz or .tar.xz file containing all source files required to run your program, as well as your separate user documentation (plain text file no more than approximately 100 lines in total) explaining your programs and how to compile and run them. You should also document any assumptions that you made in completing these tasks. Your compressed submission file must be named with your student ID as follows: 123456789_A1.tar.gz (or 123456789_A1.tar.xz), where 123456789 is your student ID number. 7. Marking criteria All three tasks are worth an equal portion of the final mark. Marks will be distributed as follows: 50% for working functionality of the three tasks according to this assignment specification. 20% for code architecture (algorithms, clear use of functions, appropriate use of libraries, correct use of arrays, etc.) in the three tasks. 10% for professionalism (compliance with the instructions in this assignment specification and clear coding style including clear and consistent indentation, clear variable names, files named/submitted as specified, etc.) 20% for documentation: both user documentation and clear in-code comments in all source code files. Don't copy the functions directly into the iotest-123456789.c program; include them in the way described near the start of this assignment document