Question
Inheritance and Polimerphism Write the Java code of 6 classes- GeometricObject, Circle, Triangle, Cylinder, Tetrahedron, and TestGeometricObject. Note : 1.The triangle should be equilateral ,2.alternative
Inheritance and Polimerphism
Write the Java code of 6 classes- GeometricObject, Circle, Triangle, Cylinder, Tetrahedron, and TestGeometricObject. Note: 1.The triangle should be equilateral ,2.alternative name of the tetrahedron is pyramid, but Tetrahedron is a special kind of pyramid, so that is why we use this name of the "pyramid" .
.The 2D figures, Circle, and Triangle are direct subclasses of the GeometricObject class and inherit the properties(public and protected data fields and methods) from it. .The 3D figure, Cylinder, is a subclass of Circle and inherits the properties(public and protected data fields and methods) from it. . The 3D Figure, Tetrahedron is a subclass of Triangle and inherits the properties(public and protected data fields and methods from it, but indirectly, from the GeometricObject class, as well. .In General: For each subclass(geometric shape) include methods to calculate the perimeter and the surface area and the volume, for the 3D figures.
I. Draw the UML class diagrams for your design(appropriately connecting the 6 classes) II. Write in Java the code for the 5 classes(the 6th class is the Test class, explained later in III.) as follows:
1)Make the GeometricObject the root class(i.e. , no extends clause) and include in the following: a.A protected String data field named color(default value "black"). b. A no-args constructor that creates a default geometric object. c.A constructor that creates a geometric object with the specified color. d. The accessor(get) and mutator(set) methods for color. e. A method toString that provides the formatting/displaying(when objects are printed) of the 1 data field listed above(Note this will override to String() method that is inherited from the class Object.) The body of this method is the following: String result=" "; result +=("The Geometric Object cold is: " + color + "and The Geometric Figure is: "); return result:
The 2D Geometric Figures
2. In the subclass Circle of the superclass GeometricObject, include the following: a. A protected double data field radius(default value 1) b. A no-args constructor that creates a default circle. c. A constructor that creates a circle with the specified radius and color. d. The accessor(get) and mutator(set) methods for radius.
e. The method to compute the perimeter of the circle( here, in the circle world, called circumference), name it getPertimeter(). f. The method to compute the area of the circle, name it getArea(). g. Override the toString() method to display everything the GeometricObject's toString() method displays(use the super keyword to invoke it.) plus the radius, perimeter(circumference), and the area of the circle, i.e.,
return super.toString() + " Circle: Radius is " +radius+ " Circumference is " +getPerimeter()+ " Area is " +getArea();
3.In the subclass Triangle of the superclass GeometricObject, include the following a. A protected double data field side (default value 1).[Remember the triangle is equilateral, hence all 3 sides are equal, and we name each of them side.] b. A no-args constructor that creates a triangle with the specified side and color. c. The accessor(get) and mutator(set) methods for side. d. A method to calculate the height(use the pythagorean theorem) of the triangle to be used in the getArea() method, shown below, in the Appendix. Name this method getHeight(). e. The method to compute the perimeter of the triangle, name it getPerimeter(). g. The method to compute the area of the triangle, name it getArea(). h. Override the toString() method to display everything the GeometricObject's to String() method displays(use the super keyword to invoke it) plus the side, perimeter, and the area of the triangle, i.e.
return super.toString() + "n Triangle: Side id " + side + " Perimeter is " + getPerimeter()) + " Area is " +getArea();
The 3D GEOMETRIC FIGURES 1. In the subclass Cylinder of the superclass Circle, include the following: a. A protected double data field height (Default value 2 ) of the cylinder. b. A no-args constructor that creates a default cylinder. c. A constructor that creates a cylinder with the specified radius, height, and color. d. The accessor(get) and mutator(set) methods for height. e. The method to compute the surfaceArea of the cylinder, name it getSurfaceArea(). Here use the Java keyword super to invoke the method for calculating the area of the circle(which is the base of the cylinder) [i.e., use super.getArea() ]. f. The method to compute the volume of the cylinder, name it getVolume(). Here, also use the super keyword to call the method for the circle area[.i.e. use super.getArea() ] g. Override the toString() method to display everything thhe GeometricObjects's and Circle's toString() methods display (use the super keyword to invoke it.) plus the height, surface area, and the volume of the cylinder, i.e.,
return super.toString() + " CYLINDER : The BASE is the CIRCLE described above" + " Height is " + height+ " Surface Area is " + getSurfaceArea() + " Volume is " + getVolume();
5. In the subclass Tetrahedron of the superclass Triangle, include the following: a. A protected double data field height (default value 2) of the tetrahedron. b. A no-args constructor that creates a tetrahedron with the specified side, height and color. d. The accessor(get) and mutator(set) methods for height. e. The method to compute the face height of the tetrahedron, name it getFaceHeight(). [This height is the height of one of the sides(Face), not the height of the base of the tetrahedron; the side is also triangle. hence, we use the Pythagorean Theorem to calculate the height of a particular side height from the tetrahedron height(GET it from 5a. above) and the 1/2 of the base height( GET it from calling the method described in 3e above)] Use getFaceHeight() int the getFaceArea() method described below. f. The method to compute the face area of the tetrahedron, name it getFaceArea(). [This area is only of the sides, not of the base of the tetrahedron). g.The method to compute the surface area of the tetrahedron, name it getVolume(). Here, also use the Java super keyword to invoke the method for calculating the area of the triangle (which is the base of the tetrahedron) [i.e. use super.getArea() ].
h. The method to compute the volume of the tetrahedron, name it getVolume(). here, also use the super keyword to call the method for the triangle area. i.Override the toString() + " TETRAHEDRON: The BASE is the TRIANGLE described above " + " Height is "+ height + " Surface Area is " +getSurfaceArea() + " Volume is " +getVolume() ;
6. The above 5.i. , should be rewritten/modified as follows: return super.toString() + " TETRAHEDRON: The BASE is the TRIANGLE described above" + " Height is " + String.format("%6.2f", height) + " Surface Area is " + String.format("%6.2f", getSurfaceArea()) + " Volume is " + String.format("%6.2f", getVolume());
III. Create a testing class called TestGeometricObject to instantiate and use each of the geometric shapes/figures. Create FOUR geometric figures(objects)as follows: circle, triangle, cylinder, and tetrahedron; each, as an instance of the respective classes. a. For each 2D figure, (1) create the instance (object) with the respective property(res) of the figure as argument(s) to its constructor, and (2) print it. i. For example, for the circle(1) to create an object, use double radius =9.8; Circle circle =new Circle (radius, "green"); ii. Then print the shape of object; for example, for the circle, (2) to print it use System.out.println(circle)
b. For each 3D Figure, (1) create the instance (object) with the respective property(res) of the figure as argument(s) to its constructor, and (2) print it.
i. For example, for the cylinder:(1) to create an object, use double radius=9.8, height=12.0; Cylinder cylinder =new Cylinder (radius, height, "red"); -etc., for the other 3D figure(tetrahedron).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
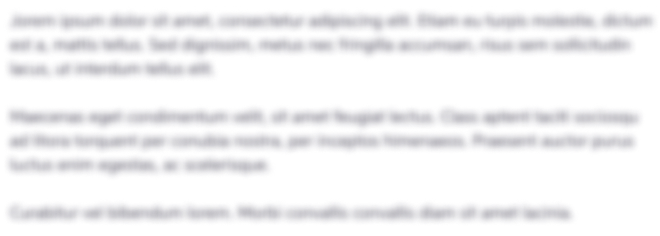
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started