Question
INLINE ASSEMBLY HELP!! please finish quicky will leave a THUMBS UP!! We included the make file, the main function that calls the program and the
INLINE ASSEMBLY HELP!! please finish quicky will leave a THUMBS UP!!
We included the make file, the main function that calls the program and the header file as well. please implement the myfloat.cpp, the template for it is also provided(bolded).Can you just please implement the given function so that they work when you call in from main.
MyFloat.cpp
#include "MyFloat.h"
MyFloat::MyFloat(){
sign = 0;
exponent = 0;
mantissa = 0;
}
MyFloat::MyFloat(float f){
unpackFloat(f);
}
MyFloat::MyFloat(const MyFloat & rhs){
sign = rhs.sign;
exponent = rhs.exponent;
mantissa = rhs.mantissa;
}
ostream& operator
//this function is complete. No need to modify it.
strm
return strm;
}
MyFloat MyFloat::operator+(const MyFloat& rhs) const{
return *this; //you don't have to return *this. it's just here right now so it will compile
}
MyFloat MyFloat::operator-(const MyFloat& rhs) const{
return *this; //you don't have to return *this. it's just here right now so it will compile
}
bool MyFolat::operator==(const float rhs) const{
return false; //this is just a stub so your code will compile
}
void MyFloat::unpackFloat(float f) {
//this function must be written in inline assembly
//extracts the fields of f into sign, exponent, and mantissa
}//unpackFloat
float MyFloat::packFloat() const{
//this function must be written in inline assembly
//returns the floating point number represented by this
float f = 0;
return f;
}//packFloat
//
MAIN. CPP :
#include#include #include "MyFloat.h" using namespace std; int main(int argc, char** argv){ float f1, f2; //float fres; MyFloat mfres; cout.precision(1000); if(argc != 4){ cout"Usage: " " float_a +/- float_b" else{ f1 = strtof(argv[1],NULL); f2 = strtof(argv[3],NULL); MyFloat mf1(f1); MyFloat mf2(f2); cout ' '' ' if(argv[2][0] == '+'){ //addition //fres = f1 + f2; mfres = mf1.operator+(mf2); //cout cout "My Add: " else if(argv[2][0] == '-'){ //subtraction //fres = f1 - f2; mfres = mf1 - mf2; //cout cout "My Subtraction: " else{ cout "Only + and - are supported but received operator: " //cout } return 0; }
MYFLOAT.h:
#ifndef MY_FLOAT_H #define MY_FLOAT_H #includeusing namespace std; class MyFloat{ public: //constructors MyFloat(); MyFloat(float f); MyFloat(const MyFloat & rhs); virtual ~MyFloat() {}; //output friend ostream& operatorconst MyFloat& f); //addition MyFloat operator+(const MyFloat& rhs) const; //subtraction MyFloat operator-(const MyFloat& rhs) const; //comparison bool operator==(const float rhs) const; private: unsigned int sign; unsigned int exponent; unsigned int mantissa; void unpackFloat(float f); float packFloat() const; }; #endif
MAKEFILE:
fpArithmetic.out: MyFloat.o main.o
g++ -g -Wall -m32 -std=c++11 -o fpArithmetic.out MyFloat.o main.o
main.o: main.cpp MyFloat.h
g++ -g -Wall -m32 -std=c++11 -c -o main.o main.cpp
MyFloat.o: MyFloat.h MyFloat.cpp
g++ -g -Wall -m32 -std=c++11 -c -o MyFloat.o MyFloat.cpp
clean:
rm main.o MyFloat.o main
PROMPT:
Print all real numbers to two decimal places unless otherwise stated .The examples provided in the prompts do not represent all possible input you can receive. All inputs in the examples in the prompt are underlined You don't have to make anything underlined it is just there to help you differentiate between what you are supposed to print and what is being given to your program .If you have questions please post them on Piazza For this assignment you will be implementing floating point add and subtract without using the hardware's floating point or double precision add. This will give you a better understanding of how difficult they are to work with and a higher appreciation of the hardware for doing this for you. You will be turning in a file called MyFloat.cpp and its associated header file, MyFloat.h, that implements your own floating point number. This object should support both + and -. 1. You may not use floating point or double precision add in your solution. This means that 1. you should not have the following in your program 1. float x,y; 2. MyFloat should represent a float using three unsigned integers: sign, exponent, and mantissa. MyFloat must have the following private methods defined on it. These functions must be implemented using inline assembly. 1. void unpackFloat(float f); 3. 1. Given a float f this function should set sign, exponent, and mantissa to the appropriate values based on the value of f. 2. float packFloat) const; 3. MyFloat must have the following public functions defined on it 1. This function should return the floating point representation of MyFloat You may declare and initialize variables to a constant in C as well as return a value but you should do no other calculations. 4. MyFloat operator+(const MyFloat& rhs) const; 1. 1. This function should add this to rhs and return the result of the addition When adding the two numbers, the maximum amount of precision must be maintained 2. Before doing the addition you should restore the leading 1. This means that the mantissa will end up taking 24 bits. Since you are adding two 24 bit numbers together the result could take up to 25 bits Be careful when shifting. Since the numbers are 32 bits, the maximum amount 1. 2. 3. Print all real numbers to two decimal places unless otherwise stated .The examples provided in the prompts do not represent all possible input you can receive. All inputs in the examples in the prompt are underlined You don't have to make anything underlined it is just there to help you differentiate between what you are supposed to print and what is being given to your program .If you have questions please post them on Piazza For this assignment you will be implementing floating point add and subtract without using the hardware's floating point or double precision add. This will give you a better understanding of how difficult they are to work with and a higher appreciation of the hardware for doing this for you. You will be turning in a file called MyFloat.cpp and its associated header file, MyFloat.h, that implements your own floating point number. This object should support both + and -. 1. You may not use floating point or double precision add in your solution. This means that 1. you should not have the following in your program 1. float x,y; 2. MyFloat should represent a float using three unsigned integers: sign, exponent, and mantissa. MyFloat must have the following private methods defined on it. These functions must be implemented using inline assembly. 1. void unpackFloat(float f); 3. 1. Given a float f this function should set sign, exponent, and mantissa to the appropriate values based on the value of f. 2. float packFloat) const; 3. MyFloat must have the following public functions defined on it 1. This function should return the floating point representation of MyFloat You may declare and initialize variables to a constant in C as well as return a value but you should do no other calculations. 4. MyFloat operator+(const MyFloat& rhs) const; 1. 1. This function should add this to rhs and return the result of the addition When adding the two numbers, the maximum amount of precision must be maintained 2. Before doing the addition you should restore the leading 1. This means that the mantissa will end up taking 24 bits. Since you are adding two 24 bit numbers together the result could take up to 25 bits Be careful when shifting. Since the numbers are 32 bits, the maximum amount 1. 2. 3
Step by Step Solution
There are 3 Steps involved in it
Step: 1
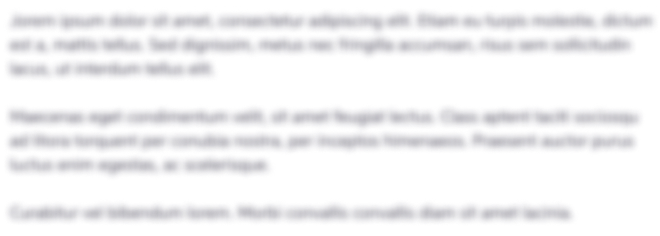
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started