Question
Instead of a String[] it must use a Doubly-Linked List with a Dummy Front and Dummy Rear to store the List's data. HOW DO I
Instead of a String[] it must use a Doubly-Linked List with a Dummy Front and Dummy Rear to store the List's data.
HOW DO I MAKE MY NODE A PRIVATE CLASS AND GET THE DESIRED OUTPUT????
//MAIN
public class AssignmentFour { public static void main(String[] args) { List myList = new List(); List emptyList = new List(myList); // Cause List Empty Message myList.removeFront(); myList.removeRear(); myList.removeItem("a"); // Cause Not found message myList.addToFront("x"); myList.removeItem("y"); myList.removeItem("x"); myList.addAfterItem("x", "z"); myList.addBeforeItem("x", "z"); // Normal behavior myList.addToFront("not."); myList.addToFront("or"); myList.addToRear("is"); myList.addToRear("try."); myList.addAfterItem("is", "no"); myList.addBeforeItem("is", "There"); myList.addToFront("Do"); myList.addAfterItem("or", "do"); myList.print("Original list"); myList.printSorted("Sorted Original List"); emptyList.print("Empty List"); List copyOfList = new List(myList); sop(" Front is " + myList.getFront()); sop("Rear is " + myList.getRear()); sop("Count is " + myList.askCount()); sop("Is There present? " + myList.isPresent("There")); sop("Is Dog present? " + myList.isPresent("Dog")); myList.addToFront("junk"); myList.addToRear("morejunk"); myList.addAfterItem("or", "moremorejunk"); myList.print("List with junk"); sop("Count is " + myList.askCount()); copyOfList.print("Untouched copy of the list"); myList.removeFront(); myList.removeRear(); myList.removeItem("moremorejunk"); myList.print("List with junk removed"); sop("Count is " + myList.askCount()); sop(""); copyOfList.print("Untouched copy of the list"); while(myList.askCount() > 0) myList.removeFront(); myList.print("List after removing all items"); copyOfList.print("Copy of List after removing all items"); } private static void sop(String s) { System.out.println(s); } }
//CLASS LIST
public class List{
private class Node{ String item; public Node next; public Node prev; //constructor public Node(java.lang.String item){ this.item = item; this.next = null; this.prev = null; } //copy constructor public List(List list){ this.front = list.front; this.rear = list.rear; this.mCount=list.mCount; }
//default constructor public List() { //list is null and Node count=0 this.mCount = 0; this.front = null; this.rear = null; }
//function addToFront() public void addToFront(java.lang.String item) { //if list is null then new node will be the front and rear if(front == null && rear == null){ this.front = new Node(item); this.rear = front; } else{ Node temp = new Node(item); temp.next = this.front; this.front.prev = temp; this.front = temp; } this.mCount+=1; }
//add To Rear() function public void addToRear(java.lang.String item) { //if list is null then front,rear will be the new node if(front == null){ this.front = new Node(item); this.rear = front; } else{ Node temp = new Node(item); this.rear.next = temp; temp.prev=this.rear; this.rear=temp; } this.mCount+= 1; } //addBeforeItem() function public void addBeforeItem(java.lang.String beforeItem, java.lang.String item) { if(this.mCount == 0){ System.out.println("List is empty"); } else{ int flag = -1; Node temp = this.front; while(temp != null){ if(temp.item.equals(beforeItem)){ flag = 1; break; } temp = temp.next; } if(flag!= -1){ if(temp == this.front){ this.addToFront(item); } else{ Node new_node = new Node(item); Node tempPrev = temp.prev; tempPrev.next = new_node; new_node.prev = tempPrev; temp.prev = new_node; new_node.next = temp; } this.mCount+= 1; } else{ System.out.println("Item Not found"); } } } //addAfterItem() function /** * * @param afterItem * @param item */ public void addAfterItem(java.lang.String afterItem, java.lang.String item) { if(this.mCount == 0){ System.out.println("List is empty"); } else{ int flag = -1; // to check afterItem is present or not Node temp = this.front; while(temp!= null){ if(temp.item.equals(afterItem)){ flag = 1; //afterItem is present break; } temp=temp.next; } if(flag!= -1){ //if afterItem is rear then we have to call addTo Rear() if(temp==this.rear){ this.addToRear(item); } else{ /* * let temp is afterNode temp -> new_node -> tempNext <- <- */ Node new_node = new Node(item); Node tempNext = temp.next; temp.next = new_node; new_node.prev = temp; new_node.next = tempNext; tempNext.prev = new_node; } this.mCount+= 1; } else{ System.out.println("Item Not found"); } }
}
public java.lang.String getFront() { if(this.front == null && this.rear == null){ return null; } else{ return this.front.item; } }
public java.lang.String getRear() { if(this.front == null && this.rear == null){ return null; } else{ return this.rear.item; } }
public boolean isPresent(String item) { Node temp = this.front;
while(temp!= null){ if(temp.item.equals(item)){ return true; //item present } temp=temp.next; } return false; }
public int askCount() { return mCount; } public void removeFront() { if(mCount!= 0){ //signleNode if(mCount == 1){ this.front = null; this.rear = null; } this.mCount-= 1; } //list empty else{ this.front = null; this.rear = null; System.out.println("List is Empty"); } } public void removeRear() { if(mCount!= 0){ //singleNode if(mCount == 1){ this.front = null; this.rear = null; } //MoreNodes else{ Node temp = this.rear.prev; this.rear = null; temp.next = null; this.rear = temp; } this.mCount-= 1; } //List is empty else{ System.out.println("List is Empty"); }
}
public void removeItem(java.lang.String item) { if(mCount == 0){ System.out.println("List is empty"); } else{ int flag=- 1; Node temp = this.front; while(temp!= null){ if(temp.item.equals(item)){ flag=1; //item found break; } temp=temp.next; } if(flag!=-1){ //front node deleted if(temp == this.front){ removeFront(); } //rear node deleted else if(temp == this.rear){ removeRear(); } //not if front or rear else{ Node tempprev = temp.prev; tempprev.next = temp.next; temp=null; this.mCount-= 1; } } else{ System.out.println("Item Not found"); } } }
public void print(java.lang.String title) { System.out.println(title); Node temp = this.front; while(temp!= null){ System.out.printf("%s ",temp.item); temp = temp.next; } System.out.println(); }
public void printSorted(java.lang.String title) { Node temp = this.front; Node temp1 = this.front; String str; for(temp1=temp;temp1!= null;temp1 = temp1.next){ if(temp.item.compareTo(temp1.item)>0){ str = temp.item; temp.item = temp1.item; temp1.item = str; } }
} //variables private int mCount; private Node front; private Node rear;
}
public class AssignmentFour { public static void main(String[] args) { List myList = new List(); List emptyList = new List(myList); // Cause List Empty Message myList.removeFront(); myList.removeRear(); myList.removeItem("a"); // Cause Not found message myList.addToFront("x"); myList.removeItem("y"); myList.removeItem("x"); myList.addAfterItem("x", "z"); myList.addBeforeItem("x", "z"); // Normal behavior myList.addToFront("not."); myList.addToFront("or"); myList.addToRear("is"); myList.addToRear("try."); myList.addAfterItem("is", "no"); myList.addBeforeItem("is", "There"); myList.addToFront("Do"); myList.addAfterItem("or", "do"); myList.print("Original list"); myList.printSorted("Sorted Original List"); emptyList.print("Empty List"); List copyOfList = new List(myList); sop(" Front is " + myList.getFront()); sop("Rear is " + myList.getRear()); sop("Count is " + myList.askCount()); sop("Is There present? " + myList.isPresent("There")); sop("Is Dog present? " + myList.isPresent("Dog")); myList.addToFront("junk"); myList.addToRear("morejunk"); myList.addAfterItem("or", "moremorejunk"); myList.print("List with junk"); sop("Count is " + myList.askCount()); copyOfList.print("Untouched copy of the list"); myList.removeFront(); myList.removeRear(); myList.removeItem("moremorejunk"); myList.print("List with junk removed"); sop("Count is " + myList.askCount()); sop(""); copyOfList.print("Untouched copy of the list"); while(myList.askCount() > 0) myList.removeFront(); myList.print("List after removing all items"); copyOfList.print("Copy of List after removing all items"); } private static void sop(String s) { System.out.println(s); } }
// DESIRED OUTPUT:
List Empty List Empty List Empty Item not found Item Not Found Item Not Found Original list Do or do not. There is no try. Sorted Original List Do There do is no not. or try. Empty List Front is Do Rear is try. Count is 8 Is There present? true Is Dog present? false List with junk junk Do or moremorejunk do not. There is no try. morejunk Count is 11 Untouched copy of the list Do or do not. There is no try. List with junk removed Do or do not. There is no try. Count is 8 Untouched copy of the list Do or do not. There is no try. List after removing all items Copy of List after removing all items Do or do not. There is no try.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
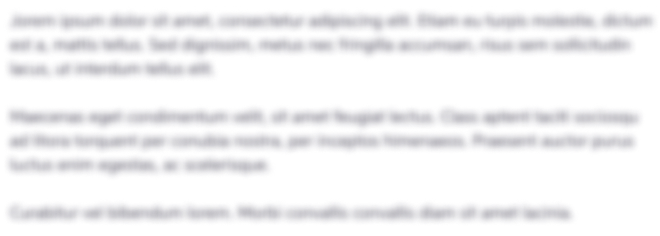
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started