Answered step by step
Verified Expert Solution
Question
1 Approved Answer
instead of fixed beta and gama, i want to implement the formula likes this beta = ( Number of New Infections per Unit Time
instead of fixed beta and gama, i want to implement the formula likes this beta Number of New Infections per Unit TimeNumber of Susceptible Individualstimes Number of Infectious Individuals
gamma Number of Recoveries per Unit TimeNumber of Infectious Individualsimport numpy as np
import matplotlib.pyplot as plt
# Parameters
beta # transmission rate
gamma # recovery rate
# Initial conditions
initialinfectious infectiousdata.iloc
initialsusceptible susceptibledata.iloc
initialrecoveries recoveriesdata.iloc
# Time settings
numdays lendates
h # time step
# SIR model differential equations
def sirmodelt y beta, gamma:
S I, R y
dSdt beta S I population
dIdt beta S I population gamma I
dRdt gamma I
return dSdt dIdt, dRdt
# RK method
def rkmethodt y h beta, gamma:
k nparraysirmodelt y beta, gamma
k nparraysirmodelt h y h k beta, gamma
k nparraysirmodelt h y h k beta, gamma
k nparraysirmodelt h y h k beta, gamma
return y hkkk k
# Heun's method
def heunmethodt y h beta, gamma:
ypredictor y h nparraysirmodelt y beta, gamma
ycorrector y hnparraysirmodelt y beta, gamma nparraysirmodelt h ypredictor, beta, gamma
return ycorrector
# Initial conditions vector
initialconditionsrkinitialsusceptible, initialinfectious, initialrecoveries
initialconditionsheun initialsusceptible, initialinfectious, initialrecoveries # Adding to make initial conditions different
# Initialize arrays to store results
resultrk npzerosnumdays,
resultheun npzerosnumdays,
# Set initial conditions
resultrk : initialconditionsrk
resultheun : initialconditionsheun
# Time integration loop
for i in range numdays:
t i h
resultrki : rkmethodt resultrki : h beta, gamma
resultheuni : heunmethodt resultheuni : h beta, gamma
printfDay i: RKresultrki : Heun resultheuni :
# Plot results for RK
dates nparange lenresultrk # resultrk contains data for each day
pltfigurefigsize
pltplotdates resultrk: label'Susceptible RK
pltplotdates resultrk: label'Infectious RK
pltplotdates resultrk: label'Recovered RK
# Plot results for Heun
dates nparange lenresultheun #resultheun contains data for each day
pltplotdates resultheun: label'Susceptible Heun
pltplotdates resultheun: label'Infectious Heun
pltplotdates resultheun: label'Recovered Heun
# Customize plot
pltxlabelDays
pltylabelNumber of Cases'
plttitleSIR Model with RK and Heun Methods'
pltlegendloc'upper right'
pltshow
Step by Step Solution
There are 3 Steps involved in it
Step: 1
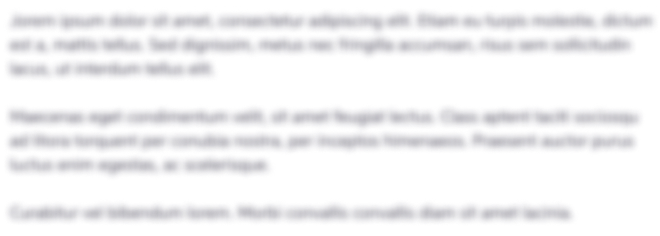
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started