Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Instruction for the practice problem can be found here: https://ds.cs.rutgers.edu/assignment-ru-kindergarten/ this is mainly about linkedLists. Note that the SNode class has (.next) that is private.
Instruction for the practice problem can be found here: https://ds.cs.rutgers.edu/assignment-ru-kindergarten/
this is mainly about linkedLists. Note that the SNode class has (.next) that is private. use getNext()
I need help with the last 4 methods in the classroom.java file. Starting with moveStudentFromChairsToLine(int size) method until seatMusicalChairWinner().
SNode.java
package kindergarten; /** * This class represents a student node, with a student * object representing the student, and a next pointer * for the student next in line. * * @author Ethan Chou */ public class SNode { private Student student; // the data part of the node private SNode next; // a link to the next student int the linked list public SNode ( Student s, SNode n ) { student = s; next = n; } public SNode() { this(null, null); } public Student getStudent () { return student; } public void setStudent (Student s) { student = s; } public SNode getNext () { return next; } public void setNext (SNode n) { next = n; } }
Student.java
package kindergarten; /** * This class represents a student, with a string variable for the * student's name and an int variable for the student's height. * * @author Ethan Chou */ public class Student { private String firstName; private String lastName; private int height; /* * Constructor */ public Student ( String f, String l, int h ) { firstName = f; lastName = l; height = h; } /* * Default constructor sets student's height to 0 (zero) */ public Student () { this(null, null, 0); } /** * Compares the names of this student to paremeter student, * returning an int value representing which name comes in first alphabetically. * * @param other the student being compared * * @return an int value of which name comes first alphabetically * n < 0 means this student's name comes before parameter student * n > 0 means parameter student's name comes before this student */ public int compareNameTo ( Student other ) { int lastNameCompare = this.lastName.compareToIgnoreCase(other.getLastName()); if ( lastNameCompare == 0 ) { // same last name, compare first names return this.firstName.compareToIgnoreCase(other.getFirstName()); } return lastNameCompare; } /* * Prints the student object */ public String print () { String printName = ""; printName += this.firstName.charAt(0) + ". "; if ( lastName.length() > 4 ) { printName += this.lastName.substring(0, 4); } else { printName += lastName; } return printName; } /* Getter and setter methods */ public String getFirstName () { return firstName; } public void setFirstName ( String f ) { firstName = f; } public String getLastName () { return lastName; } public void setLastName ( String l ) { lastName = l; } public int getHeight () { return height; } public void setHeight ( int h ) { height = h; } public String getFullName () { return this.firstName + " " + this.lastName;} }
Classroom.java: Methods to be updated!
package kindergarten; /** * This class represents a Classroom, with: * - an SNode instance variable for students in line, * - an SNode instance variable for musical chairs, pointing to the last student in the list, * - a boolean array for seating availability (eg. can a student sit in a given seat), and * - a Student array parallel to seatingAvailability to show students filed into seats * --- (more formally, seatingAvailability[i][j] also refers to the same seat in studentsSitting[i][j]) * * @author Ethan Chou * @author Kal Pandit * @author Maksims Kurjanovics Kravcenko */ public class Classroom { private SNode studentsInLine; // when students are in line: references the FIRST student in the LL private SNode musicalChairs; // when students are in musical chairs: references the LAST student in the CLL private boolean[][] seatingLocation; // represents the classroom seats that are available to students private Student[][] studentsSitting; // when students are sitting in the classroom: contains the students /** * Constructor for classrooms. Do not edit. * @param l passes in students in line * @param m passes in musical chairs * @param a passes in availability * @param s passes in students sitting */ public Classroom ( SNode l, SNode m, boolean[][] a, Student[][] s ) { studentsInLine = l; musicalChairs = m; seatingLocation = a; studentsSitting = s; } /** * Default constructor starts an empty classroom. Do not edit. */ public Classroom() { this(null, null, null, null); } /** * This method simulates students standing in line and coming into the classroom (not leaving the line). * * It does this by reading students from input file and inserting these students studentsInLine singly linked list. * * 1. Open the file using StdIn.setFile(filename); * * 2. For each line of the input file: * 1. read a student from the file * 2. make an object of type Student with the student information * 3. insert the Student object at the FRONT of the linked list * * Input file has: * 1) one line containing an integer representing the number of students in the file, say x * 2) x lines containing one student per line. Each line has the following student * information separated by spaces: FirstName LastName Height * * To read a string using StdIn use StdIn.readString() * * The input file has Students in REVERSE alphabetical order. So, at the end of this * method all students are in line in alphabetical order. * * DO NOT implement a sorting method, PRACTICE add to front. * * @param filename the student information input file */ public void enterClassroom ( String filename ) { //CODE HERE } } /** * * This method creates and initializes the seatingAvailability (2D array) of * available seats inside the classroom. Imagine that unavailable seats are broken and cannot be used. * * 1. Open the file using StdIn.setFile(seatingChart); * * 2. You will read the seating chart input file with the format: * An integer representing the number of rows in the classroom, say r * An integer representing the number of columns in the classroom, say c * Number of r lines, each containing c true or false values (true represents that a * seat is present in that column) * * 3. Initialize seatingLocation and studentsSitting arrays with r rows and c columns * * 4. Update seatingLocation with the boolean values read from the input file * * This method does not seat students on the seats. * * @param seatingChart the seating chart input file */ public void setupSeats(String seatingChart) { // CODE HERE } /** * * This method simulates students standing inline and then taking their seats in the classroom. * * 1. Starting from the front of the studentsInLine singly linked list * 2. Remove one student at a time from the list and insert the student into studentsSitting according to * seatingLocations * * studentsInLine will then be empty * * If the students just played musical chairs, the winner of musical chairs is seated separately * by seatMusicalChairsWinner(). */ public void seatStudents () { // CODE HERE } /** * Traverses studentsSitting row-wise (starting at row 0) removing a seated * student and adding that student to the end of the musicalChairs list. * * row-wise: starts at index [0][0] traverses the entire first row and then moves * into second row. */ public void insertMusicalChairs () { // CODE HERE } /** * * Removes a random student from the musicalChairs. * * @param size represents the number of students currently sitting in musicalChairs * * 1. Select a random student to be removed from the musicalChairs CLL. * 2. Searches for the selected student, deletes that student from musicalChairs * 3. Calls insertByHeight to insert the deleted student into studentsInLine list. * * Requires the use of StdRandom.uniform(int b) to select a random student to remove, * where b is the number of students currently sitting in the musicalChairs. * * The random value denotes the refers to the position of the student to be removed * in the musicalChairs. 0 is the first student */ public void moveStudentFromChairsToLine(int size) { // CODE HERE } /** * Inserts a single student, eliminated from musical chairs, to the studentsInLine list. * The student is inserted in ascending order by height (shortest to tallest). * * @param studentToInsert the student eliminated from chairs to be inserted into studentsInLine */ public void insertByHeight(Student studentToInsert) { // CODE HERE } /** * * Removes eliminated students from the musicalChairs and inserts those students * back in studentsInLine in ascending height order (shortest to tallest). * * At the end of this method, all students will be in studentsInLine besides * the winner, who will be in musicalChairs alone. * * 1. Find the number of students currently on musicalChairs * 2. While there are more than 1 student in musicalChairs, call moveRandomStudentFromChairsToLine() */ public void eliminateLosingStudents() { // CODE HERE } /* * If musicalChairs (circular linked list) contains ONLY ONE student (the winner), * this method removes the winner from musicalChairs and inserts that student * into the first available seat in studentsSitting. musicChairs will then be empty. * * This only happens when the musical chairs was just played. * * This methods does nothing if there is more than one student in musicalChairs * or if musicalChairs is empty. */ public void seatMusicalChairsWinner () { // CODE HERE } /** * * This method represents the game of musical chairs! * * This method calls previously written methods to repeatedly remove students from * the musicalChairs until there is only one student (the winner), seats the winner * and seat the students from the studentsInline. * * *****DO NOT****** UPDATE THIS METHOD */ public void playMusicalChairs() { /* DO NOT UPDATE THIS METHOD */ eliminateLosingStudents(); seatMusicalChairsWinner(); seatStudents(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
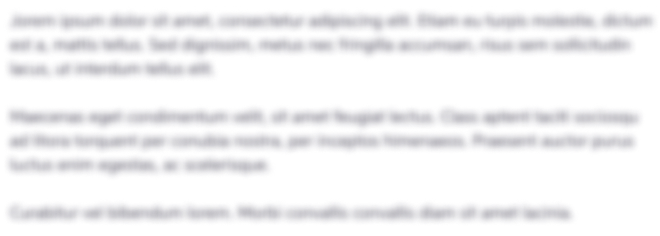
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started