Question
Instructions: Call your class Program8, so your filename will be Program8.java. It is essential for grading purposes that everyone have the same class name. Create
Instructions:
Call your class Program8, so your filename will be Program8.java. It is essential for grading purposes that everyone have the same class name.
Create several lines of comments of identification and description at the top of the file (it doesnt have to look exactly like this, in particular, if your editor wants to put * in front of every line, thats fine):
/*
Assignment : Program 8 Gradebook Program
Author : First Last
Course : Programming I, RSU
Created : nn/nn/2016
Description : [You will need to replace this with your own description.]
*/
Use descriptive variable names in camel-case with the first letter in lowercase.
Copy the methods called main, printStudentNames, and sortStudentNames from Program 8A.
In main, remove the first call to the printStudentNames method. You will still have the call to that after the call to sortStudentNames.
X
X
Move all of the statements that are currently in the main method to a new method that you will call initialize. Then in the main method, you simply call initialize(). This will help keep your program clean and much more readable and maintainable.
Add these two statements with your other static variables above the first method but after the class declaration:
private static Scanner input;
In initialize, where you declared it, remove the first word Scanner so that you just say,
input = new Scanner(System.in);
Remove all other declarations of input. You should only have one version of Scanner open at any given time. Remove all of your input.close(); statements and put one at the end of main after the brace that closes the while loop.
Test your program at this point. It should do exactly what it did in the previous assignment. Do not move on until this works! Make a backup in case you mess up later. You should make a backup after every significant change has been tested and debugged. This is for two reasons: 1. If you totally mess up on the next few steps and have trouble debugging it, you can go back to the last backup and start over from that point. 2. If you run out of time, you can turn in the last backup and still get some credit instead of taking a zero.
In the initialize method, before asking the user for the number of students, you need to ask the user how many maximum assignments there will be, and call that maxNumberAssignments. This will be a class-level static variable. Also create a class-level static final int called MISSING_GRADE and set it to -1. Then create a class-level static array called grades that has maxNumberStudents rows (rows are horizontal) and maxNumberAssignments columns (columns are vertical) and instantiate that in the main method (unless you moved all the initialization code to another method called initialize). Remember class-level, static variables are defined between the class statement and the first method:
static int [] [] grades;
They are instantiated in one of your methods, in this case in the initialize method (something is instantiated, or created, when the new statement is there):
// create the array grades and fill it with missingGrade grades = new int [maxNumberStudents][maxNumberAssignments]; for (int i = 0; i < maxNumberStudents; i++) { for (int j = 0; j < maxNumberAssignments; j++) { grades[i][j] = MISSING_GRADE; } }
In the main method, after the call to initialize, you need a loop that first asks the user what they want to do, but make a boolean done variable first and initialize it to false. There will be several options on the switch: E = Enter assignment grades, P = Print gradebook, S = Print single student grades, X = Exit. And convert selection to uppercase in case the user enters lowercase. Use a switch statement on the selection char to call a method that will do what the selection implies. For X, just set done = true and break. Here is what the code for the menu should look like:
boolean done = false; char selection = ' '; // loop for user's selection while ( ! done ) { System.out.println("Please enter a single character for your selection:"); System.out.println("E = Enter grades for an assignment"); System.out.println("P = Print all grades"); System.out.println("S = Print all grades for a given student"); System.out.println("X = Exit"); selection = input.next().charAt(0); selection = Character.toUpperCase(selection); System.out.printf("selection = %c ", selection); switch (selection) { case 'X': done = true; break; case 'E': enterAssignmentGrades(); break; case 'P': printGradebook(); break; case 'S': printStudentGrades(); break; default: break; }
E = Enter assignment grades:
Make a method called enterAssignmentGrades. This will read in all the grades for the current assignment number for each student.
Print Enter grades for Assignment N (enter -1 for missing grade), where N is the next assignment number. You will need a variable called actualNumberAssignments. Assignment numbers from the users point of view start at 1, not 0. You can handle this either by skipping the 0th element altogether, or you can just add one to the assignment number when dealing with your user.
Print Please enter each students grade after his/her name.
Go into a for-loop for each student.
Print the students name followed by a colon and space. Do NOT start a new line.
Read in the grade and store it in the proper element of your grades array. Remember the first subscript is for the student number and the second is for the assignment number, so you will be storing the grade in grades[i][actualNumberAssignents], assuming that i is your loop control variable for the student index. (Do NOT put in a 2nd loop for the assignment numbers since you are ONLY entering one assignment here.)
Test this option. Since you dont have a print method yet, you wont know for sure if its working completely right, but youll at least know that there are no compile or run-time errors.
S = Print grades for a single student:
Make a method called printStudentGrades.
Ask the user for the students number. Remember that the subscript number in your program will be one-off of the student number that was printed next to the students name. So if they give you studentNumber 4, you will set studentIndex to 3 and use studentIndex to access your arrays. You will need to make sure that the studentIndex is valid.
Call a method printHeader. Make a method called printHeader, which will print the header # Name 1 2 3 N Avg where N is the actualNumberAssignments. Youll need a for loop to print the assignment numbers.
Call a method printRow. Make a method that will print info about the student, his grades, and his average.In printRow:
Print the studentNumber, which is one more than studentIndex. Do not go to the next line.
Print studentName[studentIndex]. Do not go to the next line.
Make a for loop and print each grade belonging to that student. You should use your printf and formatters to make the grades line up. If any grade is -1, print --- instead of the -1. You still dont go to the next line.
Print the students average with one digit beyond the decimal (to the nearest tenth) and the end of line character (use %5.1f or you can use %7.1f so that blanks print in front of the average). You should NOT include the -1 in the average, and remember to divide by the number of actual grades that a student has, not by length of the array. If there are no grades, you will print --- instead of the average. So, inside that for-loop in the previous step, youll want to be adding the grade to sumGrades and adding one to numberStudentAssignments, and divide by that instead of dividing by actualNumberAssignments. Make average a double, and when you do the calculation, remember that you should NOT do integer arithmetic, so cast one of your variables to (double). If you are getting a zero for the average, it is probably because you did integer division! All these variables should be local to this method. If all of a students grades are missing (see if numberStudentAssignments is zero), print 5 hyphens -----.
Test this option. Make sure that your header and rows line up nicely.
P = Print gradebook:
Make a method called printGradebook and pass the appropriate arguments.
Call the method printHeader. You should have already made this method.
For each student, call the method printRow. You should have already made this method.
Test this option. Make sure that your header and rows line up nicely.
Sample Runs:
Please enter the maximum number of assignments: 6
Please enter the maximum number of students: 8
Please enter student names. Enter period in first name to quit.
First name: Luke
Last name : Skywalker
First name: Darth
Last name : Vader
First name: Han
Last name : Solo
First name: R2D2
Last name : Droid
First name: 3CPO
Last name : Droid
First name: .
1. Droid , 3CPO
2. Droid , R2D2
3. Skywalker , Luke
4. Solo , Han
5. Vader , Darth
Please enter a single character for your selection:
E = Enter grades for an assignment
P = Print all grades
S = Print all grades for a given student
X = Exit
?: P
selection = P
# Name Avg
1. Droid , 3CPO -----
2. Droid , R2D2 -----
3. Skywalker , Luke -----
4. Solo , Han -----
5. Vader , Darth -----
Please enter a single character for your selection:
E = Enter grades for an assignment
P = Print all grades
S = Print all grades for a given student
X = Exit
?: E
Please enter the grades for Assignment 1 next to the student's name (enter -1 if grade is missing).
1. Droid, 3CPO: 105
2. Droid, R2D2: 90
3. Skywalker, Luke: 75
4. Solo, Han: -1
5. Vader, Darth: 100
Please enter a single character for your selection:
E = Enter grades for an assignment
P = Print all grades
S = Print all grades for a given student
X = Exit
?: P
# Name 1 Avg
1. Droid , 3CPO 105 105.0
2. Droid , R2D2 90 90.0
3. Skywalker , Luke 75 75.0
4. Solo , Han --- -----
5. Vader , Darth 100 100.0
Please enter a single character for your selection:
E = Enter grades for an assignment
P = Print all grades
S = Print all grades for a given student
X = Exit
?: E
Please enter the grades for Assignment 2 next to the student's name (enter -1 if grade is missing).
1. Droid, 3CPO: 105
2. Droid, R2D2: 95
3. Skywalker, Luke: 80
4. Solo, Han: -1
5. Vader, Darth: -1
Please enter a single character for your selection:
E = Enter grades for an assignment
P = Print all grades
S = Print all grades for a given student
X = Exit
?: P
# Name 1 2 Avg
1. Droid , 3CPO 105 105 105.0
2. Droid , R2D2 90 95 92.5
3. Skywalker , Luke 75 80 77.5
4. Solo , Han --- --- -----
5. Vader , Darth 100 --- 100.0
Please enter a single character for your selection:
E = Enter grades for an assignment
P = Print all grades
S = Print all grades for a given student
X = Exit
?: S
Please enter the student's number: 3
# Name 1 2 Avg
3. Skywalker , Luke 75 80 77.5
Please enter a single character for your selection:
E = Enter grades for an assignment
P = Print all grades
S = Print all grades for a given student
X = Exit
?: S
Please enter the student's number: 4
# Name 1 2 Avg
4. Solo , Han --- --- -----
Please enter a single character for your selection:
E = Enter grades for an assignment
P = Print all grades
S = Print all grades for a given student
X = Exit
?: S
Please enter the student's number: 5
# Name 1 2 Avg
5. Vader , Darth 100 --- 100.0
Please enter a single character for your selection:
E = Enter grades for an assignment
P = Print all grades
S = Print all grades for a given student
X = Exit
?: x
Step by Step Solution
There are 3 Steps involved in it
Step: 1
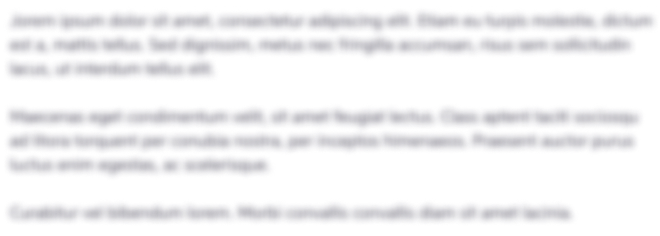
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started