Question
Instructions for Java: Task 1 - The Pair Class Many times in writing software we come across problems thatrequire us to store a pair of
Instructions for Java:
Task 1 - The Pair Class
Many times in writing software we come across problems thatrequire us to store a pair of values. For example, a coordinatesystem would require an x, y value pair or a hash table as a data,key value pair. Your task on this assignment is to create a simpletemplate pair class called Pair that has one generic / templatetype but two type parameters. You should call these parameters Fand S.
Functionality
- Constructor - You should create a working constructor thattakes two type parameters one for each of the pair values.
- getFirst - This is an accessor method that will return thefirst of the pair values.
- getSecond - This is an accessor method that will return thesecond of the pair values.
- setFirst - This is a mutator method that will set the firstpair value
- setSecond - This is a mutator method that will set the secondpair value
Task 2 The PairList Class
Using a vector and the Pair class that you created in Step 1.Create a PairList class that will hold a list of Pairs. Toconstruct the PairList class you should use the vector as a baseclass and derive the PairList class from vector.
Many student will want to derive PairList from vector and thenadd no functionality to it. The idea is that a PairList is aspecialized version of the vector. This means that you should havefunctionality that is specific to a PairList. To help youunderstand this I am going to specify what function are required.You are required at a minimum to have the followingfunctionality:
- void addPair(T first, T second) - Adds the Pair to thelist.
- T getFirst(T second) - searches on second and returnsfirst
- T getSecond(T first) - searches on first and returnssecond
- void deletePair(T first, T second) - Deletes the Pair thatcontains first and second
- void printList() - Prints the entire list of Pairs
It is important to note that the vector for the PairList shouldhold the Pair class.
Whichever method you use make sure you have a reason for doingit and be ready to explain it.
Finally
Make sure you provide code to test your functionalitythoroughly. In addition, make sure you are documenting everything.This means all methods and main with some instructions on how todemonstrate the use of your code.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
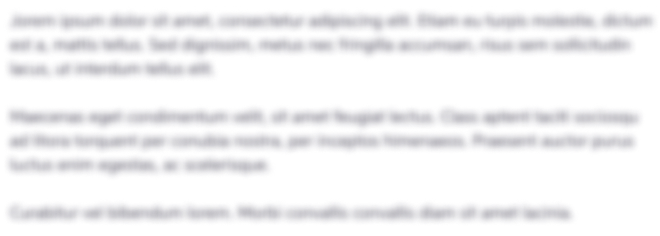
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started