Instructions reside within the uploaded photos. The language is Java
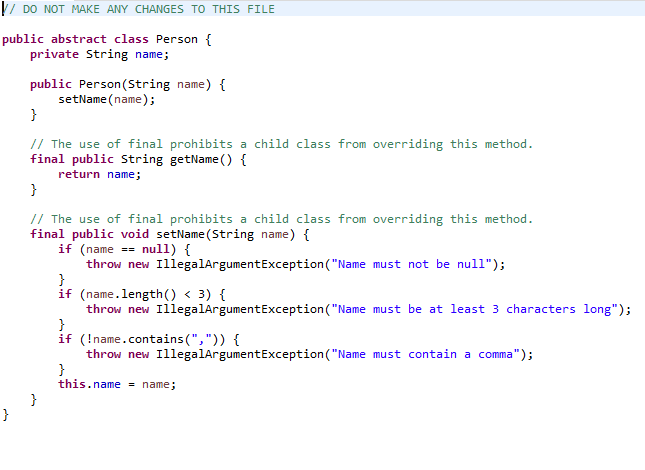
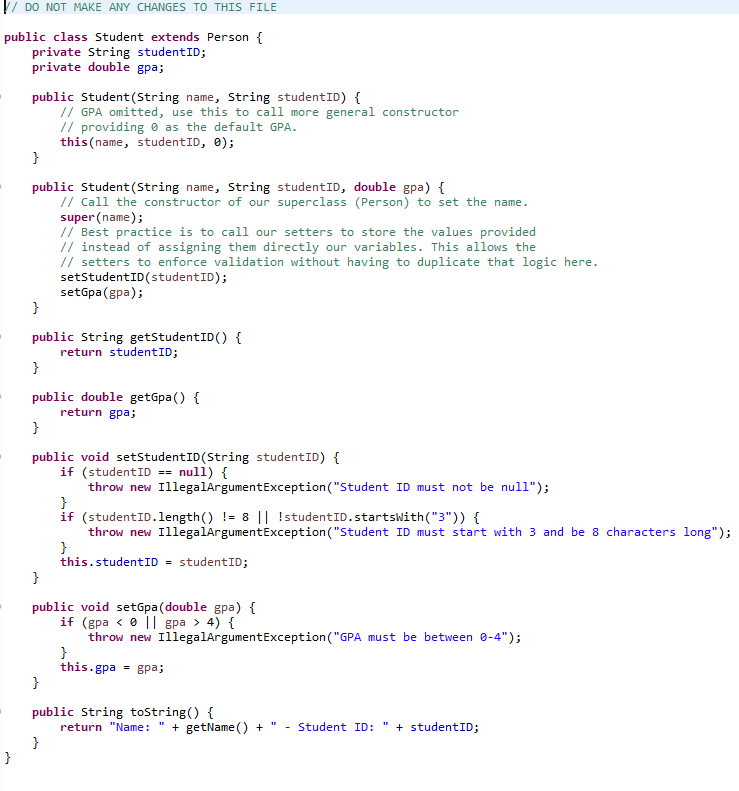
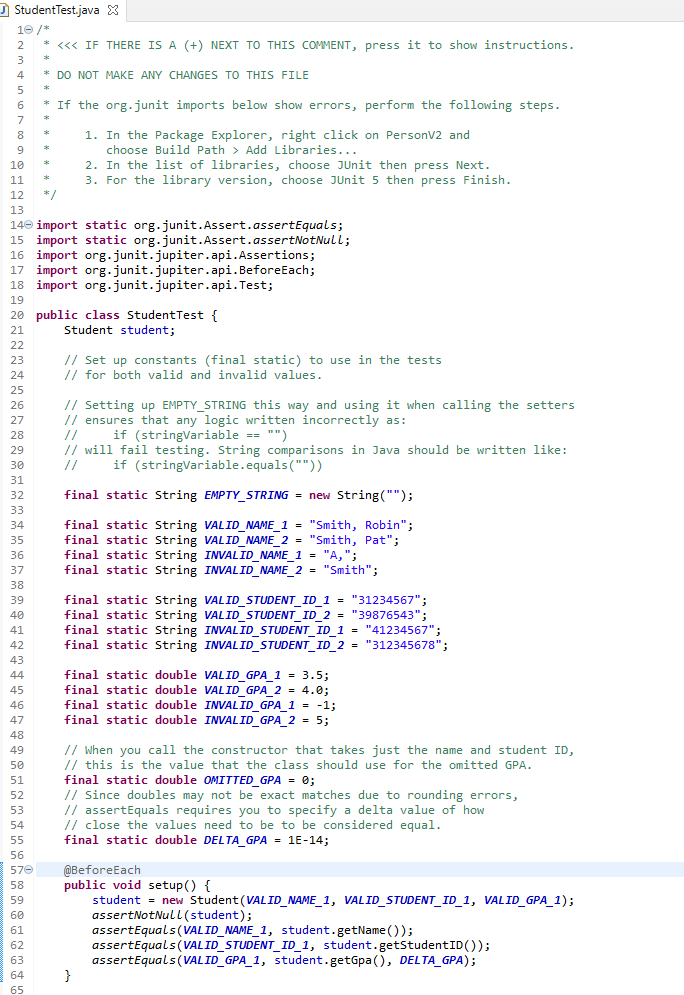
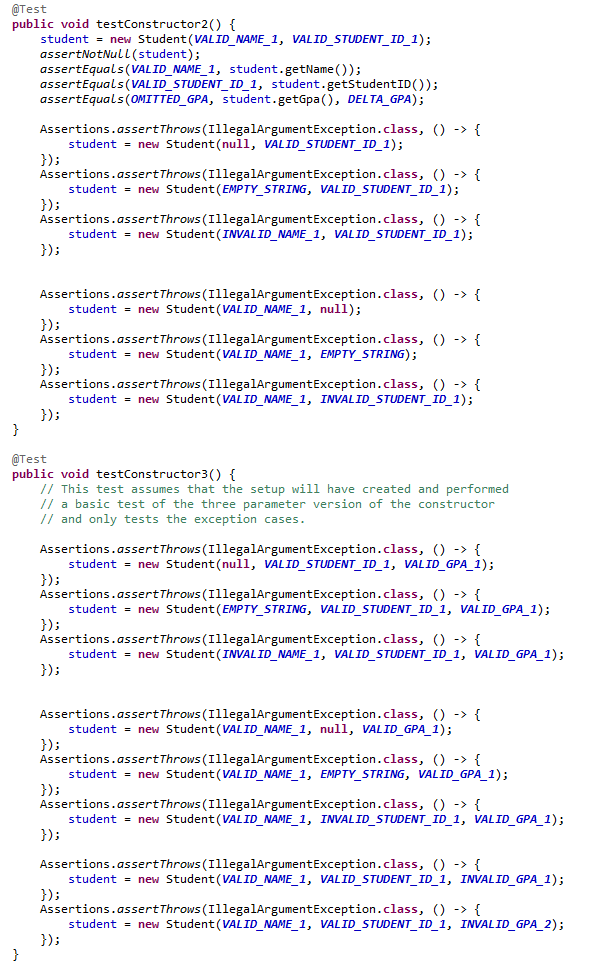
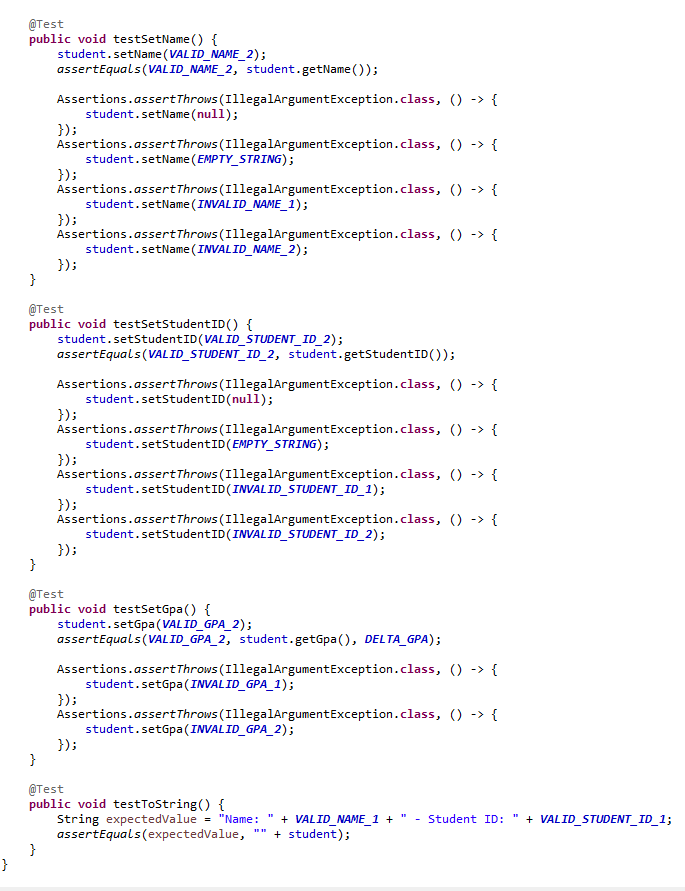
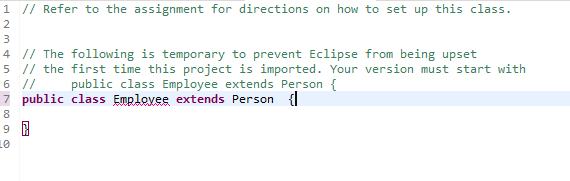
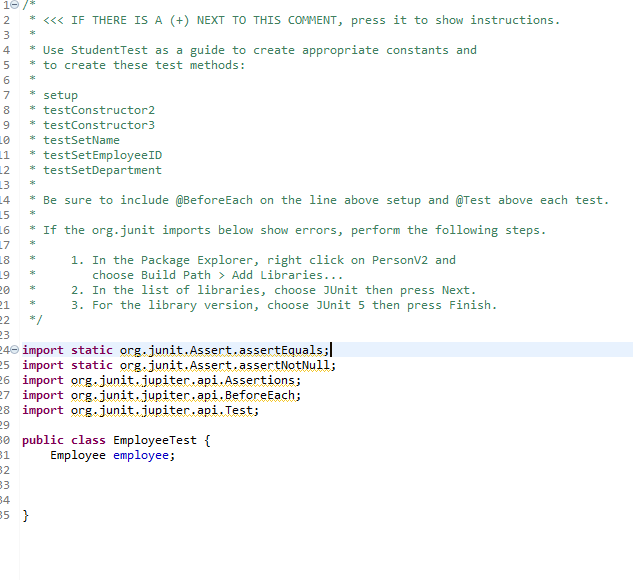
\/ DO NOT MAKE ANY CHANGES TO THIS FILE public abstract class Person { private String name; public Person(String name) { setName(name); } // The use of final prohibits a child class from overriding this method. final public String getName() { return name; } // The use of final prohibits a child class from overriding this method. final public void setName(String name) { if (name == null) { throw new IllegalArgumentException ("Name must not be null"); } if (name.length() 4) { throw new IllegalArgumentException("GPA must be between 0-4"); } this.gpa - spa; } public String toString() { return "Name: + getName() + } Student ID: + studentID; } 3 4 * * * * * Student Test.java X 10 /* 2 * Add Libraries... 10 2. In the list of libraries, choose JUnit then press Next. 11 3. For the library version, choose JUnit 5 then press Finish. 12 */ 13 140 import static org.junit. Assert.assertEquals; 15 import static org.junit. Assert. assertNotNull; 16 import org.junit.jupiter.api. Assertions; 17 import org. junit.jupiter.api. BeforeEach; 18 import org. junit.jupiter.api. Test; 19 20 public class Student Test { 21 Student student; 22 23 // Set up constants (final static) to use in the tests 24 // for both valid and invalid values. // Setting up EMPTY_STRING this way and using it when calling the setters // ensures that any logic written incorrectly as: if (stringVariable == "") // will fail testing. String comparisons in Java should be written like: if (stringVariable.equals("")) final static String EMPTY_STRING = new String(""); final static String VALID_NAME_1 = "Smith, Robin"; final static String VALID_NAME_2 = "Smith, Pat"; final static String INVALID_NAME_1 = "A,"; final static String INVALID_NAME_2 = "Smith"; final static String VALID_STUDENT_ID_1 = "31234567"; final static String VALID_STUDENT_ID_2 = "39876543"; final static String INVALID_STUDENT_ID_1 = "41234567"; final static String INVALID STUDENT_ID_2 = "312345678"; 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 570 58 59 60 61 62 63 64 65 final static double VALID_GPA_1 = 3.5; final static double VALID_GPA_2 = 4.0; final static double INVALID_GPA 1 = -1; final static double INVALID_GPA_2 = 5; // when you call the constructor that takes just the name and student ID, // this is the value that the class should use for the omitted GPA. final static double OMITTED_GPA = ; // Since doubles may not be exact matches due to rounding errors, // assertEquals requires you to specify a delta value of how // close the values need to be to be considered equal. final static double DELTA_GPA = 1E-14; @BeforeEach public void setup() { student = new Student (VALID_NAME_1, VALID_STUDENT_ID_1, VALID_GPA_1); assertNotNull(student); assertEquals(VALID_NAME_1, student.getName()); assertEquals(VALID_STUDENT_ID_1, student.getStudentID); assertEquals(VALID_GPA_1, student.getGpa(), DELTA_GPA); } @Test public void testConstructor2() { student = new Student (VALID_NAME_1, VALID_STUDENT_ID_1); assertNotNull(student); assertEquals(VALID_NAME_1, student.getName(); assertEquals(VALID_STUDENT_ID_1, student.getStudentID); assertEquals(OMITTED_GPA, student.getGpa(), DELTA_GPA); Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (null, VALID_STUDENT_ID_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (EMPTY_STRING, VALID_STUDENT_ID_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student(INVALID_NAME_1, VALID_STUDENT_ID_1); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, null); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, EMPTY_STRING); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, INVALID_STUDENT_ID_1); }); } @Test public void test Constructor3() { // This test assumes that the setup will have created and performed // a basic test of the three parameter version of the constructor // and only tests the exception cases. Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (null, VALID_STUDENT_ID_1, VALID_GPA_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (EMPTY_STRING, VALID_STUDENT_ID_1, VALID_GPA_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student(INVALID_NAME_1, VALID_STUDENT_ID_1, VALID_GPA_1); Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, null, VALID_GPA_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, EMPTY_STRING, VALID_GPA_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, INVALID_STUDENT_ID_1, VALID_GPA_1); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, VALID_STUDENT_ID_1, INVALID_GPA_1); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, VALID_STUDENT_ID_1, INVALID_GPA_2); }); } @Test public void testSetName() { student.setName(VALID_NAME_2); assertEquals(VALID_NAME_2, student.getName()); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setName(null); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setName (EMPTY_STRING); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student.setName(INVALID_NAME_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setName(INVALID_NAME_2); }); } @Test public void testSetStudentID) { student.setStudentID(VALID_STUDENT_ID_2); assertEquals(VALID_STUDENT_ID_2, student.getStudentID); Assertions.assertThrows(IllegalArgumentException.class, () -> { student.setStudentID(null); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setStudent ID(EMPTY_STRING); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student.setStudent ID(INVALID_STUDENT_ID_1); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student.setStudent ID(INVALID_STUDENT_ID_2); }); } @Test public void testSetGpa() { student.setGpa (VALID_GPA_2); assertEquals(VALID_GPA_2, student.getGpa(), DELTA_GPA); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setGpa (INVALID_GPA_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setGpa (INVALID_GPA_2); }); } @Test public void testToString() { String expectedValue = "Name: assertEquals(expectedValue, Student ID: + VALID_STUDENT_ID_1; + VALID_NAME_1 + + student); } } 1 // Refer to the assignment for directions on how to set up this class. 2 3 4 // The following is temporary to prevent Eclipse from being upset 5 // the first time this project is imported. Your version must start with 6 // public class Employee extends Person { 7 public class Employee extends Person {ll 8 10/ 2 * 3 Add Libraries... 2. In the list of libraries, choose JUnit then press Next. 3. For the library version, choose JUnit 5 then press Finish. */ 21 22 23 240 import static org. junit. Assert.assertEquals;|| 25 import static org. junit. Assert.assertNotNull; 26 import org. junit.jupiter.api.Assertions; 7 import org. junit.jupiter.api.BeforeEach; 28 import org. junit.jupiter.api.Test; 29 so public class EmployeeTest { Employee employee; UWNI 35 } \/ DO NOT MAKE ANY CHANGES TO THIS FILE public abstract class Person { private String name; public Person(String name) { setName(name); } // The use of final prohibits a child class from overriding this method. final public String getName() { return name; } // The use of final prohibits a child class from overriding this method. final public void setName(String name) { if (name == null) { throw new IllegalArgumentException ("Name must not be null"); } if (name.length() 4) { throw new IllegalArgumentException("GPA must be between 0-4"); } this.gpa - spa; } public String toString() { return "Name: + getName() + } Student ID: + studentID; } 3 4 * * * * * Student Test.java X 10 /* 2 * Add Libraries... 10 2. In the list of libraries, choose JUnit then press Next. 11 3. For the library version, choose JUnit 5 then press Finish. 12 */ 13 140 import static org.junit. Assert.assertEquals; 15 import static org.junit. Assert. assertNotNull; 16 import org.junit.jupiter.api. Assertions; 17 import org. junit.jupiter.api. BeforeEach; 18 import org. junit.jupiter.api. Test; 19 20 public class Student Test { 21 Student student; 22 23 // Set up constants (final static) to use in the tests 24 // for both valid and invalid values. // Setting up EMPTY_STRING this way and using it when calling the setters // ensures that any logic written incorrectly as: if (stringVariable == "") // will fail testing. String comparisons in Java should be written like: if (stringVariable.equals("")) final static String EMPTY_STRING = new String(""); final static String VALID_NAME_1 = "Smith, Robin"; final static String VALID_NAME_2 = "Smith, Pat"; final static String INVALID_NAME_1 = "A,"; final static String INVALID_NAME_2 = "Smith"; final static String VALID_STUDENT_ID_1 = "31234567"; final static String VALID_STUDENT_ID_2 = "39876543"; final static String INVALID_STUDENT_ID_1 = "41234567"; final static String INVALID STUDENT_ID_2 = "312345678"; 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 570 58 59 60 61 62 63 64 65 final static double VALID_GPA_1 = 3.5; final static double VALID_GPA_2 = 4.0; final static double INVALID_GPA 1 = -1; final static double INVALID_GPA_2 = 5; // when you call the constructor that takes just the name and student ID, // this is the value that the class should use for the omitted GPA. final static double OMITTED_GPA = ; // Since doubles may not be exact matches due to rounding errors, // assertEquals requires you to specify a delta value of how // close the values need to be to be considered equal. final static double DELTA_GPA = 1E-14; @BeforeEach public void setup() { student = new Student (VALID_NAME_1, VALID_STUDENT_ID_1, VALID_GPA_1); assertNotNull(student); assertEquals(VALID_NAME_1, student.getName()); assertEquals(VALID_STUDENT_ID_1, student.getStudentID); assertEquals(VALID_GPA_1, student.getGpa(), DELTA_GPA); } @Test public void testConstructor2() { student = new Student (VALID_NAME_1, VALID_STUDENT_ID_1); assertNotNull(student); assertEquals(VALID_NAME_1, student.getName(); assertEquals(VALID_STUDENT_ID_1, student.getStudentID); assertEquals(OMITTED_GPA, student.getGpa(), DELTA_GPA); Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (null, VALID_STUDENT_ID_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (EMPTY_STRING, VALID_STUDENT_ID_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student(INVALID_NAME_1, VALID_STUDENT_ID_1); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, null); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, EMPTY_STRING); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, INVALID_STUDENT_ID_1); }); } @Test public void test Constructor3() { // This test assumes that the setup will have created and performed // a basic test of the three parameter version of the constructor // and only tests the exception cases. Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (null, VALID_STUDENT_ID_1, VALID_GPA_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (EMPTY_STRING, VALID_STUDENT_ID_1, VALID_GPA_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student(INVALID_NAME_1, VALID_STUDENT_ID_1, VALID_GPA_1); Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, null, VALID_GPA_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, EMPTY_STRING, VALID_GPA_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, INVALID_STUDENT_ID_1, VALID_GPA_1); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, VALID_STUDENT_ID_1, INVALID_GPA_1); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student = new Student (VALID_NAME_1, VALID_STUDENT_ID_1, INVALID_GPA_2); }); } @Test public void testSetName() { student.setName(VALID_NAME_2); assertEquals(VALID_NAME_2, student.getName()); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setName(null); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setName (EMPTY_STRING); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student.setName(INVALID_NAME_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setName(INVALID_NAME_2); }); } @Test public void testSetStudentID) { student.setStudentID(VALID_STUDENT_ID_2); assertEquals(VALID_STUDENT_ID_2, student.getStudentID); Assertions.assertThrows(IllegalArgumentException.class, () -> { student.setStudentID(null); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setStudent ID(EMPTY_STRING); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student.setStudent ID(INVALID_STUDENT_ID_1); }); Assertions.assertThrows(IllegalArgumentException.class, () -> { student.setStudent ID(INVALID_STUDENT_ID_2); }); } @Test public void testSetGpa() { student.setGpa (VALID_GPA_2); assertEquals(VALID_GPA_2, student.getGpa(), DELTA_GPA); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setGpa (INVALID_GPA_1); }); Assertions.assertThrows (IllegalArgumentException.class, () -> { student.setGpa (INVALID_GPA_2); }); } @Test public void testToString() { String expectedValue = "Name: assertEquals(expectedValue, Student ID: + VALID_STUDENT_ID_1; + VALID_NAME_1 + + student); } } 1 // Refer to the assignment for directions on how to set up this class. 2 3 4 // The following is temporary to prevent Eclipse from being upset 5 // the first time this project is imported. Your version must start with 6 // public class Employee extends Person { 7 public class Employee extends Person {ll 8 10/ 2 * 3 Add Libraries... 2. In the list of libraries, choose JUnit then press Next. 3. For the library version, choose JUnit 5 then press Finish. */ 21 22 23 240 import static org. junit. Assert.assertEquals;|| 25 import static org. junit. Assert.assertNotNull; 26 import org. junit.jupiter.api.Assertions; 7 import org. junit.jupiter.api.BeforeEach; 28 import org. junit.jupiter.api.Test; 29 so public class EmployeeTest { Employee employee; UWNI 35 }