Question
Instructions:7.CodeMethods in PA3: DO NOT code the methods in any way other than what is instructed. A. Values that are returned by methods, and used
Instructions:7.CodeMethods in PA3: DO NOT code the methods in any way other than what is instructed.
A. Values that are returned by methods, and used multiple times in the method that calls for the value, should be stored in local variables
B. Variables used in more than one method should be class-level (fields).There are 7 class-level (fields)variables. The remaining variables are either local to the main() or some of the other methods in which they are used for processing.There are 4 variables in the main().
C. main():The logical control structures remain in this method. The other methods are called by the main(). The methods are called in the order of the code that they replace in the main(). These methods contain the code that was originally in the main()
D. setCustName():Prompt1.Assign user entry to field.
E. login(): Prompt 2 andreturns from the keyboard. It will be called by the main() in the test expression that uses the login variable, so you no longer need a login variable.
F. password(): Prompt 3and returns from the keyboard. It will be called by the main() in the test expressionthat uses the password variable, so you no longer need a
passwordvariable.
G. setShares(): Prompt 5,validate the inputas an integer before returning from the keyboard.
H. setSharePrice(): Prompt 6,validate the input as a double before returningfrom the keyboard
I. onlineTrade():Prompt 7 and returns form the keyboard.It will be called by the main() in the test expression that uses the onlineTrade variable, so you no longer need an onlineTrade variable.
J.onlineFees(): Adds the onlineFee to totalOnlineFees and totalCost.onlineFee variable scoped to this method.
K. brokerTrade(): Prompt 8 and returns form the keyboard.It will be called by the main()in the test expression that uses the brokerTrade variable, so you no longer need a brokerTrade variable.
L. commissions():Receives stockCost and calculates the commission, totalCommissions and totalCost.commission variable scoped to this method.
M. displayStockInfo():Prints the final output.Scope variables associated with the date to this method.
Code that needs to be modified.
import java.util.Scanner; import java. util.Calendar; public class PA3 { public static void main(String[] args) { String customerName = ""; int shares = 0;//declare and initialize integer for shares int noOfStocks = 0; double sharePrice = 0.0; //declare and initialize share price to double to store the price double stockCost = 0.0; //declare and initialize stock cost to double to store the cost of the stock double commissions = 0.0; //declare and initialize commissions to double to store the commissions double totalCost = 0.0; // to store the total cost of the trade double onlineFee = 0.0; // to add the online fee double totalStockCost = 0.0; //to store the total cost of the stock double totalCommissions = 0.0; double totalOnlineFees = 0.0; String userName = "", confirmUserName = "Buffet2011", password = "", confirmPassword = "Rank1Bill2008"; int attempts = 1; boolean successful = false; Scanner input = new Scanner(System.in); Calendar dateTime = Calendar.getInstance(); String date = String.format("%1$TB,%1$Td,%1$TY", dateTime);// to put the date into the correct format char onlineTrade = ' '; char brokerAssisted = ' '; char another = 'N';//the loop character set to N System.out.printf("%nYEE-TRADE, INC - The Wild West of Electronic Trading"//beginning of the program + "%nWelcome to YEE-Trade's stock purchase calculator %n"); System.out.printf("%nWhat is your name? "); customerName = input.nextLine();//store users name
for(int numAttempts = 0; numAttempts <= attempts; numAttempts++) { System.out.printf("%nPlease enter your user name: ");//collect username from user userName = input.nextLine();
System.out.printf("%nPlease enter your password: ");//collect password from user password = input.nextLine();
if(userName.equals(confirmUserName) && password.equals(confirmPassword))//confirms username and password given { attempts = -1;//ends here and goes into program
} else { switch(numAttempts) {//start of switch case 0: System.out.printf("%nInvalid log-in! %d more attempt(s).%n", attempts);//error message for amount of attempts break;
case 1://if numAttempts reaches total 1 this will output the error messages below System.out.printf("%nNo more attempts left! Contact technical support at 1-800-111-2222 "); System.out.printf("%nThank you for using Yee-Trade's stock purchase calculator!"); System.exit(0); } } } // end for { System.out.printf("%nDo you want to calculate your stock purchases? Enter 'Y' or 'N' to exit "); another = input.nextLine().charAt(0);//loop variable while (Character.toUpperCase(another) == 'Y')//to keep the program going as long as the answer is 'Y' { noOfStocks = (noOfStocks +1);//counter System.out.printf("%nHow many shares did you purchase?" ); shares = input.nextInt();//store the share integer System.out.printf("%nWhat is the price per share?" ); sharePrice = input.nextInt();//store the share price integer stockCost = (shares * sharePrice);//caluclate the stock cost totalStockCost = (totalStockCost + stockCost);//calculate the total stock cost totalCost = (totalCost + stockCost);//calculate the total cost System.out.printf("%nIs this an online trade? Enter 'Y' or 'N': "); onlineTrade = input.next().charAt(0); if(Character.toUpperCase(onlineTrade) == 'Y')//the if statement for online trade if yes add the online fee if 'N' continue to broker trade question { onlineFee = 5.95; totalOnlineFees = (totalOnlineFees + onlineFee); totalCost = (totalCost + onlineFee); } else { System.out.printf("%nIs this a broker assisted trade? Enter'Y' or 'N': "); brokerAssisted = input.next().charAt(0); if (Character.toUpperCase(brokerAssisted) == 'Y')//the broker assisted portion if the online trade is 'N' the program comes here to caclculate. { commissions = (stockCost *.02); totalCommissions = (totalCommissions + commissions); totalCost = (totalCost + commissions); } else { System.out.printf("%nINVALID TRADE TYPE!" );//if the answer to online and broker assisted is 'N' print this noOfStocks = (noOfStocks -1); totalStockCost = (totalStockCost - stockCost); totalCost = (totalCost - stockCost); } } if (noOfStocks > 0)//when noOfStocks is greater than 0 then put together the final output for that transaction { System.out.printf("%nYEE-Trade Inc. %nTOTAL COST OF STOCK PURCHASES" +"%nFOR " + customerName +"%n AS OF " +date +"%nTotal Stock Cost: $" + totalStockCost +"%nTotal Online Fees: $" + totalOnlineFees +"%ntotalCommissions: $" + totalCommissions +"%n%nTOTAL COST: $" + totalCost); } System.out.printf("%nEnter 'Y' to calculate the cost of another stock purchase or 'N' to exit: ");//to continue the transaction loop if needed another = input.next().charAt(0); } another = 'N';//loop vairable to continue or not } System.out.printf("%nThank you for using Yee-Trade's stock purchase calculator!");//end of program noOfStocks = 0; System.exit(0); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
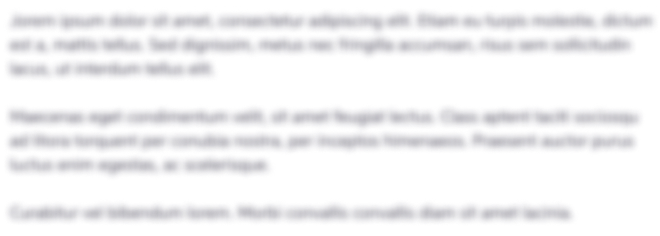
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started