Question
// *************************************************************// IntegerList.java//// Define an IntegerList class with methods to create, fill,// sort, and search in a list of integers.//// ************************************************************* public class IntegerList {
// *************************************************************// IntegerList.java//// Define an IntegerList class with methods to create, fill,// sort, and search in a list of integers.//// *************************************************************
public class IntegerList
{
int[] list; //values in the list //-------------------------------------------------------//create a list of the given size//-------------------------------------------------------public IntegerList(int size)
{
list = new int[size];
} //-------------------------------------------------------//fill array with integers between 1 and 100, inclusive//-------------------------------------------------------
public void randomize()
{
for (int i=0; i list[i] = (int)(Math.random() * 100) + 1; } //-------------------------------------------------------//print array elements with indices//-------------------------------------------------------public void print() { for (int i=0; i System.out.println(i + ":\t" + list[i]); } //-------------------------------------------------------//return the index of the first occurrence of target in the list.//return -1 if target does not appear in the list//------------------------------------------------------- public int search(int target) { int location = -1; for (int i=0; i if (list[i] == target) location = i; return location; } //-------------------------------------------------------//sort the list into ascending order using the selection sortalgorithm//------------------------------------------------------- public void selectionSort() { int minIndex; for (int i=0; i { //find smallest element in list starting at location iminIndex = i; for (int j = i+1; j if (list[j] Chapter 10: Polymorphism list[i] = list[minIndex];list[minIndex] = temp; } } } ------------------------------------------------------------------------------------------------------------------------------------------------ // ****************************************************************// IntegerListTest.java//// Provide a menu-driven tester for the IntegerList class.//// **************************************************************** import java.util.Scanner; public class IntegerListTest { static IntegerList list = new IntegerList(10); static Scanner scan = new Scanner(System.in); //-------------------------------------------------------// Create a list, then repeatedly print the menu and do what the// user asks until they quit//------------------------------------------------------- public static void main(String[] args) { printMenu(); int choice = scan.nextInt(); while (choice != 0) { dispatch(choice); printMenu(); choice = scan.nextInt(); } } //-------------------------------------------------------// Do what the menu item calls for//-------------------------------------------------------public static void dispatch(int choice) { int loc; switch(choice) { case 0: System.out.println("Bye!"); break; case 1: System.out.println("How big should the list be?"); int size = scan.nextInt(); list = new IntegerList(size); list.randomize(); break; case 2: list.selectionSort(); break; case 3: System.out.print("Enter the value to look for: "); loc = list.search(scan.nextInt()); if (loc != -1) System.out.println("Found at location " + loc); else System.out.println("Not in list"); break; case 4: list.print(); break; default: System.out.println("Sorry, invalid choice"); } } //------------------------------------------------------// Print the user's choices//------------------------------------------------------ public static void printMenu() { System.out.println(" Menu "); System.out.println(" ===="); System.out.println("0: Quit"); System.out.println("1: Create a new list (** do this first!! **)"); System.out.println("2: Sort the list using selection sort"); System.out.println("3: Find an element in the list using linear search"); System.out.println("4: Print the list"); System.out.print(" Enter your choice: "); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
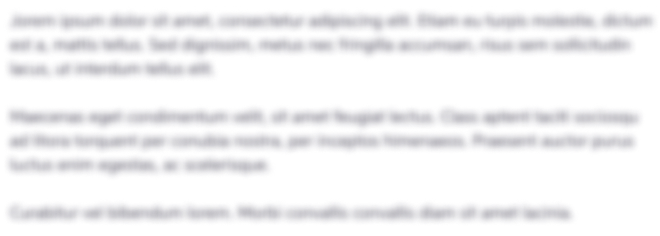
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started