Question
// ******************************************************** // Interface ListInterface for the ADT list. // ********************************************************* public interface ListInterface { boolean isEmpty(); int size(); void add(int index, Object item) throws
// ******************************************************** // Interface ListInterface for the ADT list. // ********************************************************* public interface ListInterface { boolean isEmpty(); int size(); void add(int index, Object item) throws ListIndexOutOfBoundsException; Object get(int index) throws ListIndexOutOfBoundsException; void remove(int index) throws ListIndexOutOfBoundsException; void removeAll(); String toString(); } // end ListInterface
// Please note that this code is slightly different from the textbook code //to reflect the fact that the Node class is implemented using data encapsulation
// **************************************************** // Reference-based implementation of ADT list. // **************************************************** public class ListReferenceBased implements ListInterface { // reference to linked list of items private Node head; private int numItems; // number of items in list
public ListReferenceBased() { numItems = 0; head = null; } // end default constructor
public boolean isEmpty() { return numItems == 0; } // end isEmpty
public int size() { return numItems; } // end size
private Node find(int index) { // -------------------------------------------------- // Locates a specified node in a linked list. // Precondition: index is the number of the desired // node. Assumes that 0
public Object get(int index) throws ListIndexOutOfBoundsException { if (index >= 0 && index
public void add(int index, Object item) throws ListIndexOutOfBoundsException { if (index >= 0 && index
public void remove(int index) throws ListIndexOutOfBoundsException { if (index >= 0 && index
public void removeAll() { // setting head to null causes list to be // unreachable and thus marked for garbage // collection head = null; numItems = 0; } // end removeAll
} // end ListReferenceBased
public class ListIndexOutOfBoundsException extends IndexOutOfBoundsException { public ListIndexOutOfBoundsException(String s) { super(s); } // end constructor } // end ListIndexOutOfBoundsException
//please note that this code is different from the textbook code, because the data is encapsulated! public class Node { private Object item; private Node next;
public Node(Object newItem) { item = newItem; next = null; } // end constructor
public Node(Object newItem, Node nextNode) { item = newItem; next = nextNode; } // end constructor
public void setItem(Object newItem) { item = newItem; } // end setItem
public Object getItem() { return item; } // end getItem
public void setNext(Node nextNode) { next = nextNode; } // end setNext
public Node getNext() { return next; } // end getNext } // end class Node
Problem2: Develop and test a menu-driven application program that works with input of type String with the following options. Note that option 6 and 7 are part of the application and NOT part of the functionality of the MyListReferenceBased class! You can assume unique input data. Optionally (not required for this lab) you can check for uniqueness of the Strings when inserting. 0. Exit program 1. Insert item to list. 2. Remove item from list. 3. Get item from list. 4. Clear list. 5. Display size and content of list. 6. Delete largest item in the list. 7. Reverse the list. A sample run of the program on this input data can be found here. Notes: Option 6 requires deleting the largest string in lexicographic order (use compare To). You can use the application frame from Lab2 and adapt it for this specific application. For this and all future labs: Menu cases should be handled by calling case specific methods that are passed the locally declared collection in main) as a parameterStep by Step Solution
There are 3 Steps involved in it
Step: 1
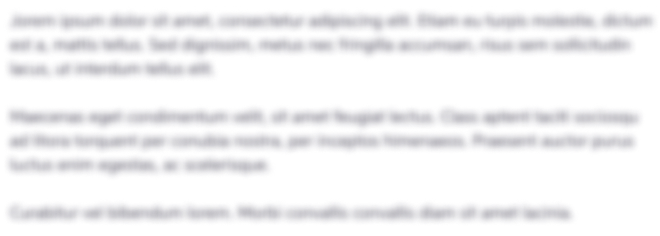
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started