Question
IntNode struct I am providing the IntNode class you are required to use. Notice that you will not code an implementation file for the IntNode
IntNode struct
I am providing the IntNode class you are required to use. Notice that you will not code an implementation file for the IntNode class. The IntNode constructor has been defined inline (within the class declaration). Do not write any other functions for the IntNode class. Use as is.
struct IntNode { int value; IntNode *next; IntNode(int value) : value(value), next(nullptr) {} };
IntList class
Encapsulated (Private) Data Fields
- head: IntNode *
- tail: IntNode *
Public Interface (Public Member Functions)
- IntList(): Initializes an empty list.
- ~IntList(): Deallocates all remaining dynamically allocated memory (all remaining IntNodes).
- void push_front(int value): Inserts a data value (within a new node) at the front end of the list.
- void pop_front(): Removes the value (actually removes the node that contains the value) at the front end of the list. Does nothing if the list is already empty.
- bool empty() const: Returns true if the list does not store any data values (does not have any nodes), otherwise returns false.
- const int & front() const: Returns a reference to the first value in the list. Calling this on an empty list causes undefined behavior.
- const int & back() const: Returns a reference to the last value in the list. Calling this on an empty list causes undefined behavior.
Global (non-member) Friend Function
- friend ostream & operator<<(ostream &, const IntList &): Overloads the insertion operator (<<) so that it sends to the output stream (ostream) a single line all of the int values stored in the list, each separated by a space. This function does NOT send a newline or space at the end of the line.
IntList.h
#ifndef INTLIST_H #define INTLIST_H
#include
struct IntNode { int value; IntNode *next; IntNode(int value) : value(value), next(nullptr) { } };
class IntList { private: IntNode *head; IntNode *tail; public: IntList(); ~IntList(); void push_front(int); void pop_front(); bool empty() const; const int & front() const; const int & back() const; friend ostream & operator<<(ostream &, const IntList &); };
#endif
main.cpp
#include
#include "IntList.h"
int main() {
//tests constructor, destructor, push_front, pop_front, display
{ cout << " list1 constructor called" << endl; IntList list1; cout << "pushfront 10" << endl; list1.push_front(10); cout << "pushfront 20" << endl; list1.push_front(20); cout << "pushfront 30" << endl; list1.push_front(30); cout << "list1: " << list1 << endl; cout << "pop" << endl; list1.pop_front(); cout << "list1: " << list1 << endl; cout << "pop" << endl; list1.pop_front(); cout << "list1: " << list1 << endl; cout << "pop" << endl; list1.pop_front(); cout << "list1: " << list1 << endl; cout << "pushfront 100" << endl; list1.push_front(100); cout << "pushfront 200" << endl; list1.push_front(200); cout << "pushfront 300" << endl; list1.push_front(300); cout << "list1: " << list1 << endl; cout << endl; cout << "Calling list1 destructor..." << endl; } cout << "list1 destructor returned" << endl; // Test destructor on empty IntList { IntList list2; cout << "Calling list2 destructor..." << endl; } cout << "list2 destructor returned" << endl; return 0; }
IDE: zybook C++
Step by Step Solution
There are 3 Steps involved in it
Step: 1
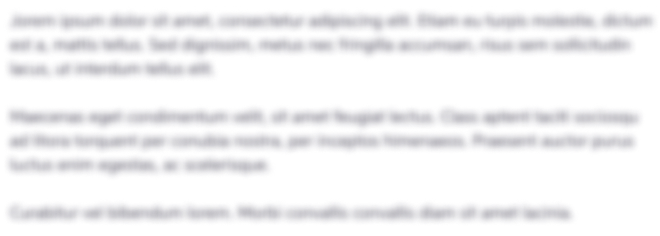
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started