Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Introduction Project 1 will cover the following: - Modifying the Is command - Implementing the sleep command - Implementing the uniq command - Implementing the
Introduction Project 1 will cover the following: - Modifying the Is command - Implementing the sleep command - Implementing the uniq command - Implementing the find command This project is a total of 100 points. As we are creating simplified versions of actual Unix commands, please test out the actual Unix commands that you are unfamiliar with before you begin to develop your own. NOTE: Make sure your code compiles using the Makefile! You will automatically lose 50% of the points if your code does not compile or does not add the command to xv6. The remaining points will be up to the discretion of the person grading the assignment based on whether what exists seems to properly implement the expected functionality. Files to Edit and Submit: Modify the Is command in Is.c, implement the sleep, uniq, and find commands in files named sleep.c, uniq.c, and find.c, respectively. Modify the Makefile to compile xv6 with the additional files. Include a Project1-README file with your group members, the system environment you used to develop/test this code, and any additional information about your implementation or compilation that might be helpful for us in grading. You will also take screenshots of your outputs for each condition. Submit your xv6-public directory as a compressed tar.gz file. See the submission section at the end for details. Academic Dishonesty: We will be checking your code against other submissions in the class for logical redundancy. If you copy someone else's code and submit it with minor changes, we will know. These cheat detectors are quite hard to fool, so please don't try. We trust you all to submit your own work only; please don't let us down. If you do, we will pursue the strongest consequences available to us. Getting Help: You are not alone! If you find yourself stuck on something, contact the course staff for help. Office hours are there for your support; please use them. If you can't make our office hours, let us know and we can talk over email or schedule a oneon-one meeting. We want these projects to be rewarding and instructional, not frustrating and demoralizing. But, we don't know when or how to help unless you ask. Modifyina Is (15 points) Currently, the Is command simply lists all files and subdirectories in the current directory. We'll modify it to be a bit more readable. We will make the following changes: 1. Automatically hide hidden files and directories (those that start with .). [5 points] 2. Include a "/" at the end of directory names so that we can differentiate them more easily from regular files. [5 points] 3. Add a "-a" flag to show hidden files and directories. [5 points] You will additionally lose points if the behavior of Is changes in any way beyond this. For example (but not limited to): - Is does not terminate successfully (-1 point) - Extraneous output to the terminal such as debug statements ( 1 point; more if there is so much extra output that it impedes grading) Implementing the sleep command (25 points) Implement the UNIX program sleep for XV6; your sleep should pause for a userspecified number of ticks. A tick is a notion of time defined by the xv6 kernel, namely the time between two interrupts from the timer chip. Your implementation of the command should be in the file sleep.c. You will also modify a few other files to make this command available in the OS. The implementation should not be complicated. This part of the project is designed to make sure you know how to add new commands to xv6 before moving on to more interesting commands. Some hints: - Before you start coding, read Chapter 1 of the xv6 book Download xv6 book. - Look at some of the other user programs (e.g., echo.c, grep.c, and rm.c) to see how you can obtain the command-line arguments passed to a program. - If the user forgets to pass an argument, sleep should print an error message. - The command-line argument is passed as a string; you can convert it to an integer using atoi (see ulib.c). - Use the system call sleep. - See sysproc. c for the xv6 kernel code that implements the sleep system call (look for sys_sleep), user.h for the C definition of sleep callable from a user program, and usys.s for the assembler code that jumps from user code into the kernel for sleep. - main should call exit (0) when it is done. - Add your sleep program to uprogs in Makefile; once you've done that, make qemu will compile your program and you'll be able to run it from the xv6 shell. Run the program from the xv6 shell: $ make qemu init: starting sh \$sleep 10 (nothing happens for a little while) $ You will additionally lose points if the behavior of Is changes in any way beyond this. For example (but not limited to): - sleep does not terminate successfully (-1 point) - Extraneous output to the terminal such as debug statements ( 1 point; more if there is so much extra output that it impedes grading) Implementing the uniq command (30 points) The uniq command in Linux is a command-line utility that reports or filters out the repeated lines in a file. In simple words, uniq is the tool that detects and deletes the adjacent duplicate lines. uniq filters out the adjacent matching lines from the input file (that is required as an argument) and writes the filtered data to the output file. For instance look at the simple file OS6611_example.txt. cat prints out the contents from the file and uniq removes duplicates from the adjacent line. Example \$ cat 0S611_example.txt I understand Operating system. I understand Operating system. I understand Operating system. I love to work on OS. I love to work on 0S. Thanks xv6. \$ uniq OS611_example.txt I understand Operating system. I love to work on OS. Thanks xv6. You will additionally implement the -c, -i, and -d flags for uniq. Please refer to the man pages and test this functionality yourself to understand what you need to implement. Functionality that we will grade you on are the following: 1. Basic uniq functionality (no flags) [15 points] 2. uniq -c filename [3 points] 3. uniq -i filename [5 points] 4. uniq -d filename [ 3 points] 5. cat filename uniq [4 points] As with the other parts of this project, you will additionally lose points if the behavior of uniq is unexpected in any way. For example (but not limited to): - uniq does not terminate successfully ( 1 point) - Extraneous output to the terminal such as debug statements (-1 point; more if there is so much extra output that it impedes grading) Implementing the find command (30 points) Write a simple version of the UNIX find program: find all the files in a directory tree with a specific name (given by the flag -name). Your solution should be in the file find.c. Some hints: - Look at Is.c to see how to read directories. - Changes to the file system persist across runs of qemu; to get a clean file system run make clean and then make qemu. Your solution is correct if produces the following output (when the file system contains the files b,a/b and a/aa/b ): $ make qemu init: starting sh $ echo >b $ mkdir a $ echo >a/b $ mkdir a/aa $ echo >a/aa/b $ find. -name b ./b ./a/b Additionally, implement simple versions of two flags. 1. the -type flag with the f and d options (for files and directories). 2. the -size flag, assuming bytes as its argument (in the complete version of find, we need to add "c" at the end for this functionality). Functionality that we will grade you on are the following (including variations without or -name included): 1. Default find functionality, find -name [15 points] 2. find -name -type f [ 3 points] 3. find name -type d [3 points] 4. find name size [3 points] 5. find -name size + [ 3 points] 6. find -name size [ 3 points] As with the other parts of this project, you will additionally lose points if the behavior of find is unexpected in any way. For example (but not limited to): - find does not terminate successfully ( 1 point) - Extraneous output to the terminal such as debug statements ( 1 point; more if there is so much extra output that it impedes grading) Submission A tar.gz file of the xv6-public with 1. All of your modified/added code and modified Makefile 2. A Project1-README file with your group member names, system environment (operating system, processor, any special steps you took for installing xv6). You may also include comments here for the graders regarding your implementation approach. The graders may use this information to help give partial credit. [if missing, 5 points] 3. Screenshots after executing "make clean", "make", and "make qemu" (or one of the alternatives such as "make qemu-nox") [if missing, 5 points] 4. Folders called Is, sleep, uniq, and find with screenshots showing outputs for each of the grading criteria (e.g., one for "uniq ", one for "uniq -c ", etc. -- don't worry about variations with > and -name omitted variations for find; we'll test those ourselves). [if missing, 1 point for each criterion]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
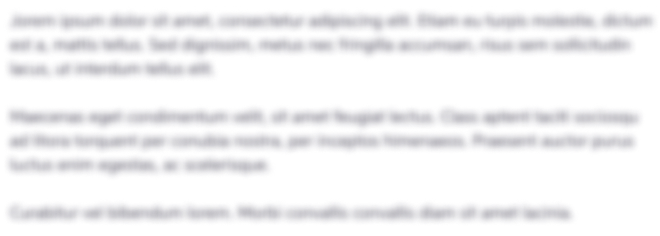
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started