Question
A servo motor (or servo) is a continuous DC motor packaged together with reduction gears, a potentiometer to measure rotation, and electronics connecting the potentiometer
A servo motor (or "servo") is a continuous DC motor packaged together with
reduction gears, a potentiometer to measure rotation, and electronics connecting
the potentiometer output to the motor drive current in a proportional-integral (PI)
feedback circuit (more on PI feedback control coming in ENME 462). Servo
motors should not be confused with "stepper motors", which typically use
permanent magnets to control the discrete positioning of a motor shaft without the
use of reduction gears, and do not generally have built-in feedback for position
control. Servo motors are exceptionally useful for simple and precise angular
position control. Unlike normal continuous DC motors and stepper motors, servo
motors generally only operate over a fixed range of output angles, typically a
range of 180o. However, servos that operate over other ranges are available, and
it is also possible to modify a servo motor for continuous rotation.
For a detailed description of servo motor fundamentals, checkout the following video:
https://www.youtube.com/watch?v=J8atdmEqZsc
An example application using servo motors to control the position of a robotic gripper is
provided on the first page of this lab. For those interested in the construction of the gripper, here
is a link to the vendor: https://www.servocity.com/parallel-gripper-kit-a
Pulse width modulation (PWM) servo motor control
Servo motors employ a standardized method for communicating the desired output rotation using
a form of pulse width modulation. A typical servo motor expects to see a signal representing the
desired output angle every 20000 µsec, or 20 msec (we will talk in microseconds here, since your
Arduino code will be easier to write if using units of µs). The control signal is simply a voltage
pulse of length tcontrol. A "short" pulse (approaching 0% duty cycle) tells the motor to move (or
"servo") the output shaft all the way to one limit (e.g. 0o), while a "long" pulse (approaching 100%
duty cycle) tells the motor to servo the shaft to the other limit (e.g. 180o). The definition of "short"
and "long" depends on the motor, so it is important to check the datasheet for your motor to find
these values. Typical values are 0o for tcontrol = 1250 µs and 180o for tcontrol = 1750 µs, with
intermediate rotations determined by linear interpolation (e.g. 90o rotation or "neutral position"
occurs for a pulse length of 1500 µs). For example, the specifications for a Futaba S3003 servo
motor are as follows:
Specifications1
Speed: 0.23 sec/60° @ 4.8V
Torque: 44 oz-in (3.2 kg-cm) @ 4.8V
Control System: +Pulse Width Control 1520 µsec Neutral
Required Pulse: 3-5 Volt Peak to Peak Square Wave
Operating Angle: 45 Deg. one side pulse traveling 400 µsec
Direction: Counter Clockwise/Pulse Traveling 1520-1900 µsec
The relevant information for PWM control of the motor is given in lines 3-6 of the specs above.
Interpreting these lines, we find that the 90o ("Neutral") position corresponds to a 1520 µsec pulse
length, and the motor can be moved over an "Operating Angle" +/- 45o from this position by
changing the pulse length by +/- 400 µsec, with an increase in pulse length corresponding to a
counter-clockwise rotation. This means a +/- 800 µsec pulse length will yield a +/- 90º (full 180º)
rotation range. For this motor, using tcontrol= 1500+/-800 µsec with a 20000 µsec total period is
recommended as a starting point.
Again, if using a different servo motor be sure to verify the proper timing signals for that specific
motor.
Note: the Arduino IDE includes a library called Servo.h that is designed to simplify servo control.
DO NOT use this library, for two reasons: (1) the library assumes a 2000 s control period and
may not reliably work for servos with a significantly longer period such as the S3003 motors used
in this lab, and (b) we want you to show that you are able to construct your own PWM code capable
of driving a servo motor!
This is also true for Lab 9: Projects. PLEASE DO NOT USE THE SERVO.H LIBRARY!
LAB INSTRUCTIONS
A. Circuit
1. Wire a potentiometer to an analog input on the Arduino such that turning the pot
generates a digital input value over the full 10-bit (0→1023) input range.
B. Arduino code
1. Need to sketch Arduino code to generate a PWM signal appropriate for driving your servo
motor (using a digital pin of your choice) to any specific angle between the full 0→180o
range based on the rotation of the potentiometer. In other words, turning the pot over its
full range should result in a PWM signal with a constant 20000 µsec total period and
tcontrol ranging from 1500-800 µsec to 1500+800 µsec (again, modified as required for
your motor). This is a good starting point. Refer to the hints at the end of the document to
learn how to figure out the optimum tcontrol for your servo. Makt it without hooking up
your servo motor to start.
a. Use of the map() function is recommended.
b. Verify that your code is working by printing (i) tcontrol and (ii) the expected servo
rotation angle to the serial port and monitoring via the serial monitor.
LAB - DEMONSTRATE IN YOUR LAB SECTION
Exercise 1. Control the servo motor using a potentiometer.
1. Unplug your Arduino from the USB cable to power it down.
2. Connect your servo motor. The servo in your kit may look
like the one in the figure at right.
The motor wires must be connected as follows:
• Orange/yellow: digital PWM signal from your
Arduino
• Red: +5 V (pro tip: use an external supply if
available. You may notice buzzing in the servo if
you use the Arduino's power supply for the motor)
• Brown: ground
Note: if your servo does not look like the above figure (if
it is a different motor), refer to the motor's datasheet online to ensure proper connections.
For example, if you have a Futaba S3003 motor, the wires must be connected as follows:
• White: digital PWM signal from your Arduino
• Red: +5 V
• Black: ground (connect the Arduino and external power supply grounds together)
3. Ensure that you are using a common ground for both the Arduino and the servo.
4. Power up the Arduino and verify that you can control the motor position over the full
180o range using the potentiometer. If necessary, modify your sketch to correct any
problems. Demonstrate your results and describe any deviations from optimal
performance.
IMPORTANT: In general, it is not a good idea to power any motor directly from the Arduino
regulated power output. The Arduino can source up to ~200 mA from its 5V output. The Futaba
3003 draws 8 mA when simply idling, and around 125 mA under no-load operation, but can
draw up to 400 mA when operating at maximum (stall) torque - DO NOT APPLY
SIGNIFICANT EXTERNAL LOAD TO YOUR MOTOR DURING OPERATION!
Otherwise there is a good chance that you will damage your Arduino.
HINTS
In order to find the correct tcontrol for your servo, you can make a test circuit:
• Use a pot as a voltage divider to input 0-5 V to an Arduino analog input pin. Convert the pot
input to duty cycle, with 0 V corresponding to 0% and 5 V to 100%.
• Declare a fixed tperiod variable in your sketch and make a manual PWM code in which the
duty cycle (tcontrol) is determined by the pot.
• Set a value for tperiod as 20 ms and turn the pot. Check whether your servo has a smooth
motion spanning 180°. Usually tperiod, also known as the servo update rate, is around 20 ms.
However, update rates as fast as 10 ms can also be used.
o If tcontrol is too short, the servo will stop at some point between 0° and 180° when you
turn the pot.
o if tcontrol is too long, you may hear "cracking" sounds at the extremes, and may see
some reverse motion. If you hear that sound, immediately turn the pot back to a point
at which the sound stops (do not destroy your servo motor).
DIGITAL TO ANALOG CONVERSION
As discussed in lecture, a R-2R DAC can be constructed using the parts in your lab kit. Here we
will design, simulate, and build a three-bit R-2R DAC, then use the voltage output from the
DAC to control the position of the servo motor.
1. Based on the theory presented in lecture, what voltage output from the DAC do you
expect for each of the 23 = 8 different bit settings? What is the least significant bit
voltage for this DAC? Be sure to show and discuss your calculations.
2. Using Circuitlab.com, build and simulate the three-bit R-2R DAC. Use a +5V voltage
source to provide VFSR to the circuit, along with a voltmeter to display the voltage output
from the DAC. You may use Single Pole Double Throw switches in place of transistors.
The In-Class Exercise from our DAC lecture provides an example of this configuration.
Simulate your DAC in Circuitlab to demonstrate/prove the output voltage for each of the
different bit settings as calculated in Question 1 above. You may need a table
detailing each output to discuss in your lab section, but you must show the Circuitlab
simulation for at least two different bit settings in your lab section.
3. Using resistor values of your choosing, build the three-bit R-2R DAC from the parts in
your lab kit. Provide +5V to the circuit and measure the output from the DAC via your
Arduino UNO for reach of the different bit settings. You may need a table detailing
each output to discuss in your lab section, but you must show the physical circuit
operating for at least two different bit settings in your lab section.
Discuss your results. How well did the output voltage readings from the physical circuit align
with the theory and simulation? If you needed smaller step sizes in the DAC output voltage for a
particular application, how would you modify the circuit?
Exercise 2. Control the servo motor using the three-bit R-2R DAC.
Repeat the steps of Exercise 1, but now control the position of the servo using only the DAC
output voltage. How many positions can the servo motor be oriented using the DAC? How does
this compare to using the potentiometer in Exercise 1? If you needed smaller step size in angular
position of the servo motor, how could you modify the DAC circuit?
Be sure to discuss the answers to these questions in your lab section.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
This document provides detailed instructions and guidelines for a lab activity involving the use of ...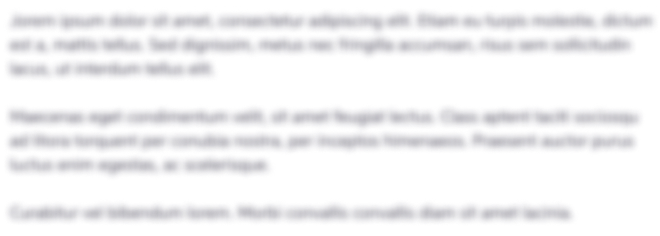
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started