Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Introduction: This assignment is designed to help you get familiar with the language and structure of the programs we will be writing for this course.
Introduction: This assignment is designed to help you get familiar with the language and structure of the programs we will be writing for this course. Having a centralized logger can be very useful for a program both in debugging and in execution. Implement a simple file- and console-logging facility as a class library in C++, Procedure: Using the editor of your choice, create a blank workspace/project/solution/folder/whatever called "Logging." In this workspace, create a console application called Log Driver, and replace the generated main.cpp file with the name LogDriver.cpp. Then, add code and header files for the implementation of the Logger class, Logger.cpp and Logger.hpp, as well as any other files for your included data structures. Main objective: In the Logger project, modify the provided class called "Logger" in Logger.cpp. The Logger must support all the features listed in Logger.hpp and must faithfully keep the log file, console, and memory log up to date with log messages. To submit your assignment, first you must "make clean your project. Then, go to your project folder and verify that all 'debug.' 'bin' and/or 'obj' files/directories have been deleted. Finally, zip up your project directory and submit it to Canvas. General notes/hints: The Logger should be allowed to run with or without a log file name being specified at instantiation. The memory log should always hold the last 100 log messages. You will need to decide how to handle the case where a user might try to turn on file logging without having first specified a log file to the Logger constructor. You may want to add additional code to the end of the driver program to test more features or functionality of the Logger. Please leave any existing code and hop files unmodified as assurance that the changes you make don't affect the proper execution of the program as given Grading: Program compiles and all Logger methods implemented: Program executes without crashing: Program gives correct output: Program gives correct output using my test-driver-on-steroids: Code is formatted consistently and coding practices are followed: 50% 10% 20% 10% 10% #include #include "Logger.hpp int main() { 17 Instantiate a class which provides ILogger functionality... Logger logger("test.txt"); logger.Log("Test... 1, 2, 3..."); logger.EnableFileLogging(); logger.Log("Test... 4, 5, 6..."); logger.DisableFileLogging(); logger.Log("You should not see this line in the file!"); logger.DisableConsoleLogging(); logger.Log("You should not see this line until memory is purged!"); logger.EnableConsoleLogging(); logger.Log("You should not see this line in the file either."); logger.EnableFileLogging(); logger.Log("This should not be the only line in your log file!"); logger.DisableConsoleLogging(); logger.Log("You should only see this line in the file!"); logger.EnableConsoleLogging(); logger.Log("You should see this line in both."); // write your own tests below here... return 0; 11:59 Done 1 of 7 #include "Logger.hpp" II/ II/ Constructor to start a logger using a specified file name II/ II/ A string containing path for file Logger::Logger(std::string fileName) /// II/ Destructor to tear down a logger II/ Logger::-Logger() II/ II/ Method to log some string data II/ II/ A string to put as a line of text in the log III True if line was logged properly, false otherwise returns> bool Logger::Log(std::string line) return false; III II/ Control method to turn on file-based logging /// void Logger::EnableFileLogging II/ II/ Control method to turn off file-based logging II/ 11:59 Done 1 of 8 Il/ void Logger::DisableFileLogging) II/ II/ Control method to turn on console-based logging II/ void Logger::EnableConsoleLogging II/ Ill Control method to turn off console-based logging II/ void Logger::DisableConsoleLogging /// II/ String property to view details of the implementation II/ std::string Logger::GetInfo() // return the version number of your Logger return ; II/ II/ Boolean property which reflects if the log is currently logging to a file II/ bool Logger::IsFileLogEnabled() return false; Il// /// Boolean property which reflects if the log is currently logging to the console 12:00 Done 1 of 8 II/ Ill Control method to turn on console-based logging II/ void Logger::EnableConsoleLogging II/ Ill Control method to turn off console-based logging II/ void Logger::DisableConsoleLogging /// II/ String property to view details of the implementation II/ std::string Logger::GetInfo() // return the version number of your Logger return ; II/ II/ Boolean property which reflects if the log is currently logging to a file III bool Logger::IsFileLogEnabled() return false; II/ II/ Boolean property which reflects if the log is currently logging to the console II/ bool Logger::Is ConsoleLogEnabled() return false; 12:00 Back Logger.hpp #ifndef Logger_hpp #define Logger_hpp #include class Logger private: public: /// 11/ Constructor to start a logger using a specified file name /// /// A string containing path for file Logger (std::string fileName); /// /// Destructor to tear down a logger /// -Logger(); /// /// Method to log some string data /// /// A string to put as a line of text in the log /// true if line was logged properly, false otherwise bool Log(std::string line); /// /// Control method to turn on file-based logging /// void EnableFileLogging(); /// 1/1 Control method to turn off file-based logging /// void DisableFileLogging(); /// /// Control method to turn on console-based locaina Dashboard Calendar To Do Notifications Inbox 12:01 void EnableFileLogging(); /// /// Control method to turn off file-based logging /// void DisableFileLogging(); /// /// Control method to turn on console-based logging /// void EnableConsoleLogging(); /// /// Control method to turn off console-based logging /// void DisableConsoleLogging(); /// /// Control method to purge the memory log of all messages. /// Should honor the destination(s) enabled for log messages /// void PurgeMemory Log(); /// /// String property to view details of the implementation /// std::string GetInfo(); /// /// Boolean property which reflects if the log is currently logging to a file /// bool IsFileLogEnabled(); /// /// Boolean property which reflects if the log is currently logging to the console /// bool IsConsole LogEnabled(); #endif /* Logger_hpp */ 35 Dashboard Calendar To Do Notifications inbox Introduction: This assignment is designed to help you get familiar with the language and structure of the programs we will be writing for this course. Having a centralized logger can be very useful for a program both in debugging and in execution. Implement a simple file- and console-logging facility as a class library in C++, Procedure: Using the editor of your choice, create a blank workspace/project/solution/folder/whatever called "Logging." In this workspace, create a console application called Log Driver, and replace the generated main.cpp file with the name LogDriver.cpp. Then, add code and header files for the implementation of the Logger class, Logger.cpp and Logger.hpp, as well as any other files for your included data structures. Main objective: In the Logger project, modify the provided class called "Logger" in Logger.cpp. The Logger must support all the features listed in Logger.hpp and must faithfully keep the log file, console, and memory log up to date with log messages. To submit your assignment, first you must "make clean your project. Then, go to your project folder and verify that all 'debug.' 'bin' and/or 'obj' files/directories have been deleted. Finally, zip up your project directory and submit it to Canvas. General notes/hints: The Logger should be allowed to run with or without a log file name being specified at instantiation. The memory log should always hold the last 100 log messages. You will need to decide how to handle the case where a user might try to turn on file logging without having first specified a log file to the Logger constructor. You may want to add additional code to the end of the driver program to test more features or functionality of the Logger. Please leave any existing code and hop files unmodified as assurance that the changes you make don't affect the proper execution of the program as given Grading: Program compiles and all Logger methods implemented: Program executes without crashing: Program gives correct output: Program gives correct output using my test-driver-on-steroids: Code is formatted consistently and coding practices are followed: 50% 10% 20% 10% 10% #include #include "Logger.hpp int main() { 17 Instantiate a class which provides ILogger functionality... Logger logger("test.txt"); logger.Log("Test... 1, 2, 3..."); logger.EnableFileLogging(); logger.Log("Test... 4, 5, 6..."); logger.DisableFileLogging(); logger.Log("You should not see this line in the file!"); logger.DisableConsoleLogging(); logger.Log("You should not see this line until memory is purged!"); logger.EnableConsoleLogging(); logger.Log("You should not see this line in the file either."); logger.EnableFileLogging(); logger.Log("This should not be the only line in your log file!"); logger.DisableConsoleLogging(); logger.Log("You should only see this line in the file!"); logger.EnableConsoleLogging(); logger.Log("You should see this line in both."); // write your own tests below here... return 0; 11:59 Done 1 of 7 #include "Logger.hpp" II/ II/ Constructor to start a logger using a specified file name II/ II/ A string containing path for file Logger::Logger(std::string fileName) /// II/ Destructor to tear down a logger II/ Logger::-Logger() II/ II/ Method to log some string data II/ II/ A string to put as a line of text in the log III True if line was logged properly, false otherwise returns> bool Logger::Log(std::string line) return false; III II/ Control method to turn on file-based logging /// void Logger::EnableFileLogging II/ II/ Control method to turn off file-based logging II/ 11:59 Done 1 of 8 Il/ void Logger::DisableFileLogging) II/ II/ Control method to turn on console-based logging II/ void Logger::EnableConsoleLogging II/ Ill Control method to turn off console-based logging II/ void Logger::DisableConsoleLogging /// II/ String property to view details of the implementation II/ std::string Logger::GetInfo() // return the version number of your Logger return ; II/ II/ Boolean property which reflects if the log is currently logging to a file II/ bool Logger::IsFileLogEnabled() return false; Il// /// Boolean property which reflects if the log is currently logging to the console 12:00 Done 1 of 8 II/ Ill Control method to turn on console-based logging II/ void Logger::EnableConsoleLogging II/ Ill Control method to turn off console-based logging II/ void Logger::DisableConsoleLogging /// II/ String property to view details of the implementation II/ std::string Logger::GetInfo() // return the version number of your Logger return ; II/ II/ Boolean property which reflects if the log is currently logging to a file III bool Logger::IsFileLogEnabled() return false; II/ II/ Boolean property which reflects if the log is currently logging to the console II/ bool Logger::Is ConsoleLogEnabled() return false; 12:00 Back Logger.hpp #ifndef Logger_hpp #define Logger_hpp #include class Logger private: public: /// 11/ Constructor to start a logger using a specified file name /// /// A string containing path for file Logger (std::string fileName); /// /// Destructor to tear down a logger /// -Logger(); /// /// Method to log some string data /// /// A string to put as a line of text in the log /// true if line was logged properly, false otherwise bool Log(std::string line); /// /// Control method to turn on file-based logging /// void EnableFileLogging(); /// 1/1 Control method to turn off file-based logging /// void DisableFileLogging(); /// /// Control method to turn on console-based locaina Dashboard Calendar To Do Notifications Inbox 12:01 void EnableFileLogging(); /// /// Control method to turn off file-based logging /// void DisableFileLogging(); /// /// Control method to turn on console-based logging /// void EnableConsoleLogging(); /// /// Control method to turn off console-based logging /// void DisableConsoleLogging(); /// /// Control method to purge the memory log of all messages. /// Should honor the destination(s) enabled for log messages /// void PurgeMemory Log(); /// /// String property to view details of the implementation /// std::string GetInfo(); /// /// Boolean property which reflects if the log is currently logging to a file /// bool IsFileLogEnabled(); /// /// Boolean property which reflects if the log is currently logging to the console /// bool IsConsole LogEnabled(); #endif /* Logger_hpp */ 35 Dashboard Calendar To Do Notifications inbox







Step by Step Solution
There are 3 Steps involved in it
Step: 1
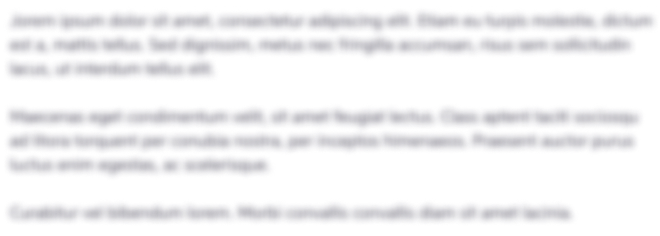
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started