Question
Introduction This is the 1st of a set of 3 exercises that are intended to lead you through the steps necessary to master the use
Introduction
This is the 1st of a set of 3 exercises that are intended to lead you through the steps necessary to master the use of UNIX datagram sockets to build a request-reply protocol. You are to use Linux machines (you do not need administrator privileges to develop these exercises). A good tutorial on UNIX sockets is provided, in addition to references to other useful resources. You are to use C++ prototypes. Definitions for use with C++ are given as appendices at the end of this document.
Exercise 1: A server that echoes client input
You are to produce client and server programs based on the procedures DoOperation , GetRequest and SendReply. These operations have been simplified so that client and server exchange messages consisting of strings.
A client and a server behave as follows: Client: this takes the name of the server computer as an argument (i.e. at the command line). It repeatedly requests a string to be entered by the user, and uses message to send the string to the server, awaiting a reply. Each reply should be printed out. Server: repeatedly receives a string using GetRequest, prints it on the screen and replies with SendReply. The server exits when the string consists of the single character `q' is first received.
The client and the server programs should be built on top of an OO class hierarchy, which utilizes sockets and concurrency primitives. You may use the set of C++ class interfaces presented in the appendix to build your client and server infrastructure, or design your own if you want.
Demonstration
You must demonstrate that your programs work correctly by running two clients and a server on three different computers. As part of this exercise, you should design an experiment to find out whether you can cause datagrams to be dropped. Describe the experiment and discuss its results in a comment in your client program.
Submission
The client and server programs should have separate code (unlike the demonstration program UDPsock.c).
Provide the main program for the client and the server. The code for the client should include a comment containing a short write-up describing the experiment for testing the reliability of datagrams.
APPENDIX 1: Definitions for C++ Programs
In the exercises you are advised to make use of the following:
#ifndef MESSAGE_H #define MESSAGE_H enum MessageType { Request, Reply}; class Message { private: MessageType message_type; int operation; void * message; size_t message_size; int rpc_id; public: Message(int operation, void * p_message, size_t p_message_size,int p_rpc_id); Message(char * marshalled_base64); char * marshal (); int getOperation (); ing getRPCId(); void * getMessage(); size_t getMessageSize(); MessageType getMessageType(); void setOperation (int _operation); void setMessage (void * message, size_t message_size); void setMessageType (MessageType message_type); ~Message(); }; #endif // MESSAGE_H This class encapsulates the state of the message to be sent between a client and a server. Notice the method
"marshal" which converts the data members of an object instantiated from this class to a data stream. I recommend using a data stream format that avoids the null character as you may send binary data; a good candidate for that is the base64 format, and you can read about the base64 encode and decode here http://en.wikipedia.org/wiki/Base64. Of course there are other alternatives that you can use but you need to be very careful and have very good reasons for your choice.
The "unmarshalling" is carried out via the second parameterized constructor, which receives a base64 formatted character array. In exercise 1 you will not need to utilize the "operation" and "rpc_id" data members but they will be needed in the next phases of the project. The message type should be set by the client and the server objects whose class definitions are presented below. For exercise 2 you will definitely need to extend this class either through inheritance of through amendment to account for other data attributes of the media file to be transferred such as file name, file attributes, storage location, etc.
#ifndef UDPSOCKET_H #define UDPSOCKET_H class UDPSocket {
protected: int sock; sockaddr_in myAddr; sockaddr_in peerAddr; char * myAddress; char * peerAddress; int myPort; int peerPort; bool enabled; pthread_mutex_t mutex; public:
UDPSocket (); void setFilterAddress (char * _filterAddress); char * getFilterAddress (); bool initializeServer (char * _myAddr, int _myPort); bool initializeClient (char * _peerAddr, int _peerPort); int writeToSocket (char * buffer, int maxBytes ); int writeToSocketAndWait (char * buffer, int maxBytes,int microSec ); int readFromSocketWithNoBlock (char * buffer, int maxBytes ); int readFromSocketWithTimeout (char * buffer, int maxBytes, int timeoutSec, int timeoutMilli); int readFromSocketWithBlock (char * buffer, int maxBytes ); int readSocketWithNoBlock (char * buffer, int maxBytes ); int readSocketWithTimeout (char * buffer, int maxBytes, int timeoutSec, int timeoutMilli); int readSocketWithBlock (char * buffer, int maxBytes ); int getMyPort (); int getPeerPort (); void enable(); void disable(); bool isEnabled(); void lock(); void unlock(); int getSocketHandler(); ~UDPSocket ( ); }; #endif // UDPSOCKET_H The UDPSocket class encapsulates all the data and methods necessary to establish communication between two entities. This class acts as a parent class to the UDPClientSocket and the UDPServerSocket. Notice the wealth of methods for reading and writing from a UDP socket with different timeout options.
#ifndef UDPCLIENTSOCKET_H #define UDPCLIENTSOCKET_H class UDPClientSocket : public UDPSocket {
public: UDPClientSocket (); bool initializeClient (char * _peerAddr, int _peerPort); ~UDPClientSocket ( ); }; #endif // UDPCLIENTSOCKET_H This class represents a client UDP socket and it inherits from theUDPSocket class. It has an initialization method that initializes the UDPSocket in a client mode #ifndef UDPSERVERSOCKET_H #define UDPSERVERSOCKET_H class UDPClientSocket : public UDPSocket { public: UDPServerSocket (); bool initializeServer (char * _myAddr, int _myPort); ~UDPServerSocket ( ); }; #endif // UDPSERVERSOCKET_H
Step by Step Solution
There are 3 Steps involved in it
Step: 1
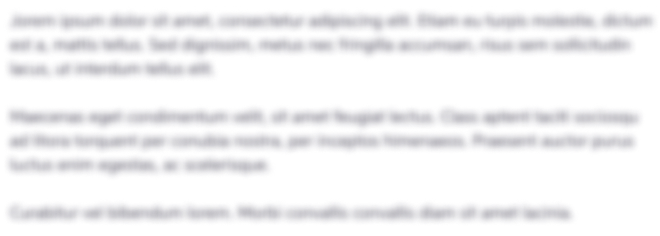
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started