Question
Introduction This weeks lab will look at a bad design using a combination of static and instance variables. Like in the homework, we have a
Introduction
This weeks lab will look at a bad design using a combination of static and instance variables. Like in the homework, we have a LandPlot class. There is a problem with the interaction between static and instance variables. Your task is to identify a problem and come up with a design change.
The Design
The LandPlot class has four private object fields: a String for the owner, a double for the length, a double for the width, and a double for the value of the LandPlot. It also has one private static class field: a double for the pricePerSquareFoot for all LandPlots. (You can see static variables in the debugger by using Show Static Variables see the picture in the slides)
The constructor takes values for the owner, width, and length from its parameters, and it computes the value based in this plots length and width, and the current pricePerSquareFoot. The only method that changes the state of the object is updatePrice, which takes a new value to update the static pricePerSquareFoot, and then updates the value for this LandPlot based on the new pricePerSquareFoot.
The Problem
You can infer from the unit tests that there is an expectation about LandPlot objects. We expect that, at any given time, if we multiply the length and width of a LandPlot with the pricePerSquareFoot for LandPlots, we should get the value of the plot. This assumption is being violated.
To Get Credit
Bare minimum: write an explanation of the design problem. Why is the test failing where it is? What is wrong with the design?
Good: come up with and describe a better design for the LandPlot class that has the same public methods, but will also pass the given test.
Excellent: implement this better design in the project. You may write answers on the reverse of this document.
public class LandPlot {
//private object fields
private String owner;
private double length;
private double width;
private double value;
//private static mutable variable
private static double pricePerSquareFoot = 1;
public LandPlot(String name, double lengthInFeet, double widthInFeet) {
owner = name;
length = lengthInFeet;
width = widthInFeet;
value = length * width * pricePerSquareFoot;
}
public String getOwner() {
return owner;
}
public double getLength() {
return length;
}
public double getWidth() {
return width;
}
public double getValue() {
return value;
}
//static getter
public static double getPricePerSquareFoot() {
return pricePerSquareFoot;
}
/**
* object method to update the current price Per Acre
* set the static pricePerSquareFoot to equal the passed value
* then update the value field accordingly
* @param newPrice
*/
public void updatePrice(double newPrice) {
pricePerSquareFoot = newPrice;
value = length * width * pricePerSquareFoot;
}
}
import junit.framework.TestCase;
public class TestLandPlot extends TestCase{
private static final double EPSILON = 0.005;
public void setUp() {
}
//test default values (for primitive numeric variables these are generally 0 values)
public void test00() {
assertEquals(LandPlot.getPricePerSquareFoot(), 1.0, EPSILON);
LandPlot plotA = new LandPlot("A", 20, 20);
LandPlot plotB = new LandPlot("B", 20, 10);
assertEquals(computeValue(plotA), plotA.getValue(), EPSILON);
assertEquals(computeValue(plotB), plotB.getValue(), EPSILON);
plotA.updatePrice(1.5);
assertEquals(computeValue(plotA), plotA.getValue());
assertEquals(computeValue(plotB), plotB.getValue());
}
private double computeValue(LandPlot plot) {
return plot.getLength() * plot.getWidth() * LandPlot.getPricePerSquareFoot();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
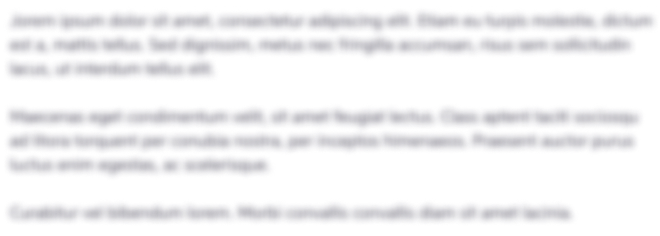
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started